How should PHP handle response formats when implementing APIs
Today, API has become an indispensable part of major enterprises and developers. PHP is a widely used language, so understanding how to handle API response formats is crucial for PHP developers. When writing an API, the response format is usually JSON, XML or YAML. So, how to handle API response format in PHP? This article will answer this question.
- JSON response format
First, let’s take a look at the JSON response format. JSON (JavaScript Object Notation) is a lightweight data exchange format that is easy to read and write. When writing APIs in PHP, it is very common to return JSON format.
The method to implement the JSON response format is very simple. You just need to use the json_encode() function to convert the data and key/value pairs into JSON format. For example:
$person = array( "name" => "John Doe", "age" => 30, "city" => "New York" ); echo json_encode($person);
The above code will display the response in the following JSON format:
{"name":"John Doe","age":30,"city":"New York"}
This is a basic example, but from here you can extend it to more complex and deeply nested data structure, depending on your API and application needs.
- XML response format
Many older versions of the API use XML (Extensible Markup Language) to handle responses. This format is still very common as well. Although less readable, its structured organization and good scalability still make it a popular data exchange format.
Compared with JSON, the method of implementing XML response format is slightly more complicated. PHP provides two methods, namely DOM (Document Object Model) and SimpleXML (Simple XML). DOM is more powerful and supports more XML operations, while SimpleXML is simpler and easier to use.
The following is some sample code using SimpleXML to implement the XML response format:
$person = new SimpleXMLElement('<person/>'); $person->addChild('name', 'John Doe'); $person->addChild('age', 30); $person->addChild('city', 'New York'); echo $person->asXML();
The above code will display the response in the following XML format:
<person> <name>John Doe</name> <age>30</age> <city>New York</city> </person>
- YAML response format
Finally, let's take a look at a relatively new format, YAML (YAML Ain't Markup Language). It is more readable than XML and JSON due to its concise syntax and readable format. Similar to JSON, YAML also has a nested structure.
PHP does not support YAML by default, but you can use the LibYAML extension to use the YAML format. The following is a sample code that implements the YAML response format:
$person = array( "name" => "John Doe", "age" => 30, "city" => "New York" ); echo yaml_emit($person);
The above code will display the response in the following YAML format:
age: 30 city: New York name: John Doe
- Handling Error Responses
In When writing an API, you also need to consider how to handle error responses. For example, if the API request is invalid, an error message needs to be returned. Each format offers different handling of this situation.
In JSON, you can return a response similar to the following format:
{"status": "error", "message": "Invalid API request"}
In XML, you can return:
<error> <status>error</status> <message>Invalid API request</message> </error>
YAML response format An error response similar to JSON.
Summary
Handling API response formats in PHP is very simple, just use the appropriate functions and data structures. Whichever format you choose, you need to make sure it is easy to read and parse, and correctly reflects your API and application data. At the same time, you also need to ensure that error responses are handled correctly to ensure the stability and security of the API.
The above is the detailed content of How should PHP handle response formats when implementing APIs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
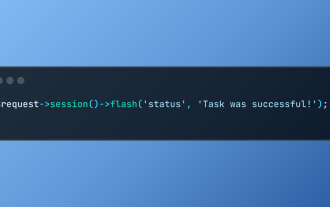
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
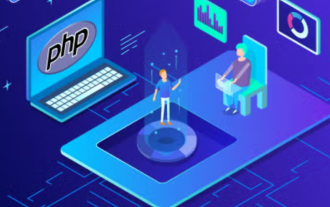
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
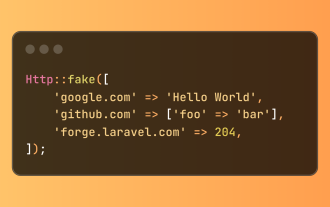
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
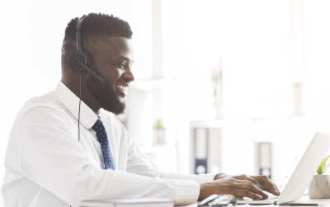
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
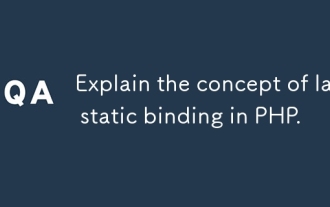
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
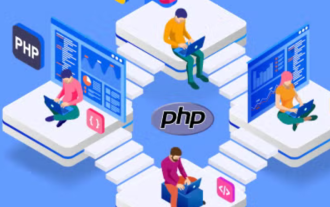
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
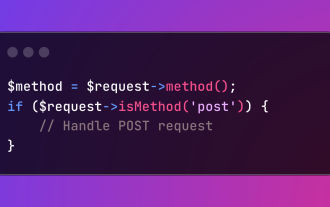
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building
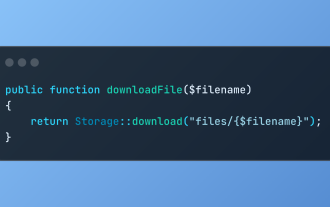
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
