


How to handle parallel and asynchronous requests in PHP backend API development
With the continuous development and changes of network applications, handling parallel and asynchronous requests has become an important topic in PHP back-end API development. In traditional PHP applications, requests are performed synchronously, that is, a request will wait until a response is received, which will affect the response speed and performance of the application. However, PHP now has the ability to process parallel and asynchronous requests. These features allow us to better handle a large number of concurrent requests and improve the response speed and performance of the application.
This article will discuss how to handle parallel and asynchronous requests in PHP backend API development. We'll introduce PHP's parallel and asynchronous request handling mechanisms and discuss how to apply them to API development.
What is parallel and asynchronous request processing?
Parallel processing refers to processing multiple requests or tasks at the same time, that is, performing multiple operations at the same time. This is a way to speed up application processing. Parallel processing can be done using multiple threads, multiple processes, or multiple servers, depending on the characteristics of the application and the environment.
Asynchronous processing is an event-driven programming model that does not block during program execution, but continues processing after execution. In PHP, asynchronous processing is usually done using callback functions or coroutines, which makes it easier for applications to implement non-blocking I/O and high concurrent requests.
PHP’s asynchronous request processing
PHP version 5.3 introduces support for asynchronous request processing. PHP uses multiple extensions to implement asynchronous processing, the most commonly used of which are libevent and event. These extensions provide a set of APIs that can be used to create event loops, register callback functions, listen to sockets, and more. PHP's asynchronous request processing mechanism can realize non-blocking I/O, high concurrent requests, long connections, etc.
The following is a sample code using libevent extension:
$base = event_base_new();
$dns_base = evdns_base_new($base, 1);
$event = event_new();
event_set($event, $socket, EV_READ | EV_PERSIST, function($fd, $what, $arg) {
// Processing the socket Word event
});
event_base_set($event, $base);
event_add($event);
event_base_loop($base);
In this example, we use the event_base_new() function to create an event loop, then use the event_new() function to create an event object, and use the event_set() function to register an event handler for the event object. Finally, we start the event loop to listen for socket events through the event_base_loop() function.
Parallel processing of PHP
PHP can use multiple processes or threads when processing parallel requests. PHP's multi-process support is provided by the pcntl extension, while multi-thread support is implemented through the pthreads extension. We will learn about PHP's parallel processing mechanism by introducing these two extensions.
Multi-process processing
Using PHP's pcntl extension, we can run multiple processes at the same time, thereby speeding up application processing. The following is a sample code that uses the pcntl_fork() function to create a child process:
$pid = pcntl_fork();
if ($pid == -1) {
// Failed to create child process
die('Could not fork');
} else if ($pid) {
//The parent process executes the code here
// Wait for the child process to end
pcntl_wait($status);
} else {
// The child process executes the code here
// Processing requests or tasks
exit;
}
In this example, we use the pcntl_fork() function to create a child process, and then in the child process Process the request or task, and finally use the exit() function to end the child process.
Multi-threading
Using PHP's pthreads extension, we can use threads to process requests or tasks. The following is a sample code that uses pthreads extension to create a thread:
class MyThread extends Thread {
public function run() {
// Processing requests or tasks
}
}
$myThread = new MyThread();
$myThread -> start();
$myThread -> ; join();
In this example, we use the pthreads extension to create a thread object, use the start() function to start the thread, and then use the join() function to wait for the thread to end.
Summary
This article introduces parallel and asynchronous request processing technology in PHP back-end API development. Parallel processing in PHP can use multiple processes or threads, while asynchronous processing is usually done using callback functions or coroutines. These technologies can help us better handle a large number of concurrent requests and improve application response speed and performance.
The above is the detailed content of How to handle parallel and asynchronous requests in PHP backend API development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
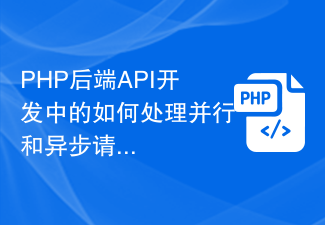
As web applications continue to develop and change, handling parallel and asynchronous requests has become an important topic in PHP backend API development. In traditional PHP applications, requests are performed synchronously, that is, a request will wait until a response is received, which will affect the response speed and performance of the application. However, PHP now has the ability to handle parallel and asynchronous requests. These features allow us to better handle a large number of concurrent requests and improve the response speed and performance of the application. This article will discuss how to deal with PHP backend API development
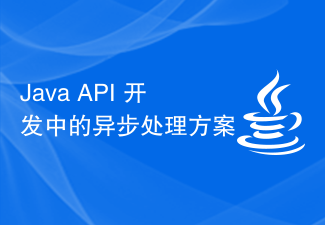
With the continuous development of Java technology, Java API has become one of the mainstream solutions developed by many enterprises. During the development process of Java API, a large number of requests and data often need to be processed, but the traditional synchronous processing method cannot meet the needs of high concurrency and high throughput. Therefore, asynchronous processing has become one of the important solutions in JavaAPI development. This article will introduce asynchronous processing solutions commonly used in Java API development and how to use them. 1. Java differences
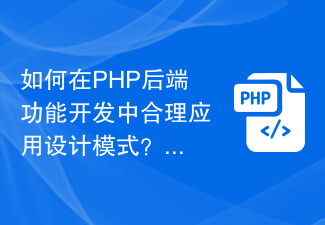
How to reasonably apply design patterns in PHP back-end function development? A design pattern is a proven solution template for solving a specific problem that can be used to build reusable code, improving maintainability and scalability during the development process. In PHP back-end function development, reasonable application of design patterns can help us better organize and manage code, improve code quality and development efficiency. This article will introduce commonly used design patterns and give corresponding PHP code examples. Singleton mode (Singleton) Singleton mode is suitable for those who need to maintain
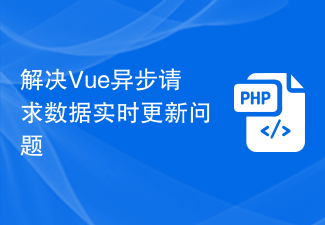
How to solve the problem of real-time update of asynchronous request data in Vue development. With the development of front-end technology, more and more web applications use asynchronous request data to improve user experience and page performance. In Vue development, how to solve the problem of real-time update of asynchronous request data is a key challenge. Real-time update means that when the asynchronously requested data changes, the page can be automatically updated to display the latest data. In Vue, there are multiple solutions to achieve real-time updates of asynchronous data. 1. Responsive machine using Vue
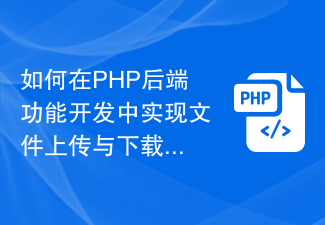
How to implement file upload and download in PHP back-end function development? In web development, file upload and download are very common functions. Whether users upload images, documents or download files, back-end code is required to process them. This article will introduce how to implement file upload and download functions on the PHP backend, and attach specific code examples. 1. File upload File upload refers to transferring files from the local computer to the server. PHP provides a wealth of functions and classes to implement file upload functions. Create HTML form first, in HTM
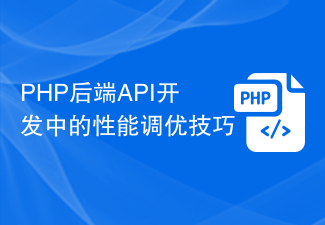
With the rapid development of the Internet, more and more applications adopt the Web architecture, and PHP, as a scripting language widely used in Web development, has also received increasing attention and application. With the continuous development and expansion of business, the performance problems of PHPWeb applications have gradually been exposed. How to perform performance tuning has become an important challenge that PHPWeb developers have to face. Next, this article will introduce performance tuning techniques in PHP back-end API development to help PHP developers better
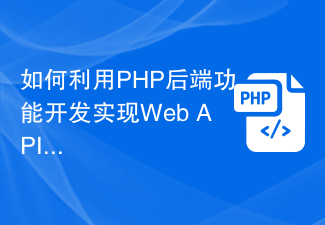
How to use PHP backend functions to develop and implement WebAPI? With the development of the Internet, the importance of WebAPI is increasingly recognized and valued by people. WebAPI is an application programming interface that allows information exchange and interoperability between different software applications. PHP, as a back-end language widely used in web development, can also be used well to develop and implement WebAPI. This article will introduce how to use PHP backend functions to implement a simple WebAPI, and give relevant
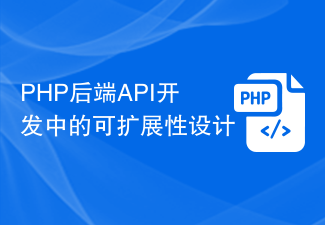
With the rapid development of the Internet, more and more business requirements require data exchange through the network, in which API plays a vital role as an intermediary for data transmission. In PHP back-end API development, scalability design is a very important aspect, which can make the system more adaptable and scalable, and improve the robustness and stability of the system. 1. What is scalability design? Scalability means that a certain function of the system can add additional functions to meet business needs without affecting the original performance of the system. exist
