How to build a RESTful API using Flask
In today's software development field, RESTful API has become a core component of many applications, which can provide reliable, decoupled, and easy-to-maintain services. Flask is a lightweight Python web framework that provides a flexible way to build web applications as well as RESTful APIs. In this article, we will introduce how to use Flask to build a RESTful API.
- Installing Flask
First, you need to make sure that Python and pip are installed. Open a terminal or command prompt on your system and enter the following command to install Flask:
pip install Flask
- Create project folder
In this example, we create a project folder named It is the project folder of "flask-restful". Enter the following command in the terminal to create the folder:
mkdir flask-restful
- Create a virtual environment
It is recommended to use a virtual environment to avoid installing Flask globally on the system, this can Help us develop multiple projects on the same computer. Enter the following command in the terminal to create the virtual environment:
python3 -m venv env
- Activate the virtual environment
To activate the virtual environment in the terminal, enter the following command:
For Mac/Linux:
source env/bin/activate
For Windows:
envScriptsctivate
- Add necessary dependencies
In a Flask application, you need to use flask_restful library and other libraries to safely perform RESTful API requests. These dependencies can be installed using the pip command in a virtual environment:
pip install flask_restful pip install flask_cors
- Creating the application master file
Before creating our RESTful API, we need to create a Application master file. Create a file called "app.py" in the project folder and add the following code:
from flask import Flask from flask_restful import Api, Resource, reqparse app = Flask(__name__) api = Api(app) users = [ { "name": "Alice", "age": 22, "occupation": "Software Engineer" }, { "name": "Bob", "age": 26, "occupation": "Data Analyst" }, { "name": "Charlie", "age": 33, "occupation": "UI/UX Designer" } ] class User(Resource): def get(self, name): for user in users: if(name == user["name"]): return user, 200 return "User not found", 404 api.add_resource(User, "/user/<string:name>") if __name__ == '__main__': app.run(debug=True)
The above code creates a Flask application instance called "app" and an instance called "api "flask_restful object. It also defines some data to store user information. In this application, we also define a class called "User", which inherits from flask_restful's "Resource" class. The User class defines two methods - get and post. We only implemented the get method, which is used to obtain user data with a specified name. Finally, bind the User class to the /user/
- Run the application
In a terminal or command prompt, enter the following command to start the application:
python app.py
Then, visit http:// /localhost:5000/user/Alice (or the name of other users) can obtain the user data.
- Add CORS support
CORS stands for cross-origin resource sharing. When using RESTful APIs, CORS support can be used in the development environment to avoid cross-domain resource issues. In our application, we added CORS support using the flask_cors library. To add CORS support, simply add the following code to your application main file:
from flask_cors import CORS app = Flask(__name__) api = Api(app) CORS(app)
This code is added after the application instance and api definition. It binds CORS middleware to the application instance.
Conclusion
In this article, we introduced how to build a RESTful API using Flask. We start by installing Flask, then create the project folder, virtual environment, add dependencies, and create the main application file. We also learned how to add CORS support to help solve cross-origin resource request issues. Now you can easily build RESTful API services using Flask.
The above is the detailed content of How to build a RESTful API using Flask. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
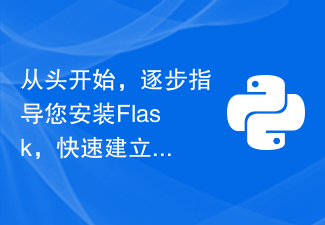
Starting from scratch, I will teach you step by step how to install Flask and quickly build a personal blog. As a person who likes writing, it is very important to have a personal blog. As a lightweight Python Web framework, Flask can help us quickly build a simple and fully functional personal blog. In this article, I will start from scratch and teach you step by step how to install Flask and quickly build a personal blog. Step 1: Install Python and pip Before starting, we need to install Python and pi first
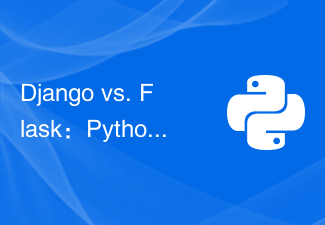
Django and Flask are both leaders in Python Web frameworks, and they both have their own advantages and applicable scenarios. This article will conduct a comparative analysis of these two frameworks and provide specific code examples. Development Introduction Django is a full-featured Web framework, its main purpose is to quickly develop complex Web applications. Django provides many built-in functions, such as ORM (Object Relational Mapping), forms, authentication, management backend, etc. These features allow Django to handle large
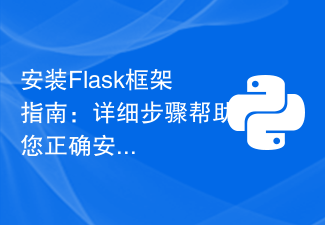
Flask framework installation tutorial: Teach you step by step how to correctly install the Flask framework. Specific code examples are required. Introduction: Flask is a simple and flexible Python Web development framework. It's easy to learn, easy to use, and packed with powerful features. This article will lead you step by step to correctly install the Flask framework and provide detailed code examples for reference. Step 1: Install Python Before installing the Flask framework, you first need to make sure that Python is installed on your computer. You can start from P
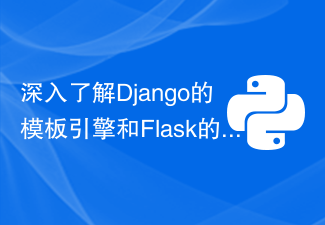
In-depth understanding of Django's template engine and Flask's Jinja2 requires specific code examples. Introduction: Django and Flask are two commonly used and popular web frameworks in Python. They both provide powerful template engines to handle the rendering of dynamic web pages. Django uses its own template engine, while Flask uses Jinja2. This article will take an in-depth look at Django’s template engine and Flask’s Jinja2, and provide some concrete code examples to illustrate their use.
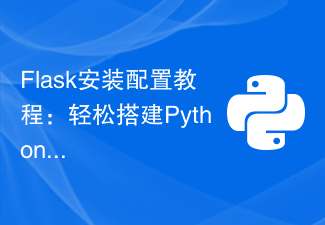
Flask installation and configuration tutorial: A tool to easily build Python Web applications, specific code examples are required. Introduction: With the increasing popularity of Python, Web development has become one of the necessary skills for Python programmers. To carry out web development in Python, we need to choose a suitable web framework. Among the many Python Web frameworks, Flask is a simple, easy-to-use and flexible framework that is favored by developers. This article will introduce the installation of Flask framework,
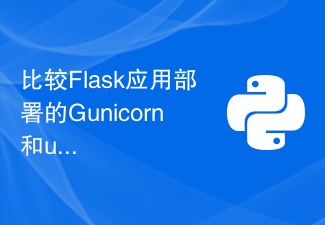
Flask application deployment: Comparison of Gunicorn vs suWSGI Introduction: Flask, as a lightweight Python Web framework, is loved by many developers. When deploying a Flask application to a production environment, choosing the appropriate Server Gateway Interface (SGI) is a crucial decision. Gunicorn and uWSGI are two common SGI servers. This article will describe them in detail.
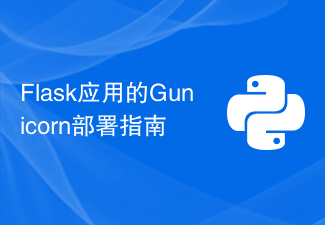
How to deploy Flask application using Gunicorn? Flask is a lightweight Python Web framework that is widely used to develop various types of Web applications. Gunicorn (GreenUnicorn) is a Python-based HTTP server used to run WSGI (WebServerGatewayInterface) applications. This article will introduce how to use Gunicorn to deploy Flask applications, with

Django, Flask, and FastAPI: Choose the one that best suits your development needs, specific code examples required Introduction: In modern web development, choosing the right framework is crucial. As Python continues to develop in the field of web development, frameworks such as Django, Flask and FastAPI are becoming more and more popular among developers. This article will introduce the characteristics and applicable scenarios of these three frameworks, combined with specific code examples, to help you choose the framework that best suits your development needs. 1. D
