Use MySQL in Go language to implement non-relational data processing
With the rapid development of the Internet, non-relational databases (NoSQL) are becoming more and more widely used, and in the Go language, there are also some non-relational ways of processing data. However, for some traditional relational databases, such as MySQL, we can also use some techniques to implement non-relational data processing functions.
This article will introduce the methods and techniques of using MySQL to implement non-relational data processing in Go language, including how to create and design a non-relational table, and demonstrate how to read data through examples. , write and update. In this process, we will use one of these libraries: github.com/go-sql-driver/mysql.
1. Create non-relational tables
For MySQL database, we usually think of it as a relational database, so we need to design the table and maintain the relationship between the tables . But sometimes, we need to store data in a non-relational structure, such as JSON format data.
In MySQL, we can use JSON type fields to implement non-relational storage. For example, we create a table to store user information, which contains a JSON type field "info" to store the user's personal information:
CREATE TABLE users ( id INT(11) NOT NULL AUTO_INCREMENT, name VARCHAR(50) NOT NULL, info JSON NOT NULL, PRIMARY KEY (id) );
In the above code, we created a table named "users ” table, which contains three fields: id, name and info. The type of the info field is JSON, which is used to store the user's personal information. In practical applications, we can also use other non-relational types, such as BLOB, TEXT, etc.
2. Use Go language to connect to MySQL database
Connecting to MySQL database in Go language requires the use of a third-party library, the most commonly used of which is github.com/go-sql-driver/mysql. First, you need to install this library, which can be installed through the following command:
go get github.com/go-sql-driver/mysql
This library provides a sql.DB type that can be used for database connections and operations. Before connecting to the database, we need to provide some configuration information, as follows:
dsn := "root:password@(127.0.0.1:3306)/database_name?charset=utf8mb4&parseTime=True&loc=Local" db, err := sql.Open("mysql", dsn) if err != nil { panic(err) } defer db.Close()
dsn is a data source name used to connect to the database. This includes user name, password, host address, port number, database name, encoding method and other information. In the above code, we used the local host address and the default 3306 port number, and replaced username and password with the corresponding values. If the connection is successful, the db variable will contain a reference to the MySQL database that you have connected to.
3. Go language to read and write non-relational data
After connecting to the MySQL database, we can use the sql.DB type to execute SQL queries and obtain related data. For non-relational data, we can use MySQL's JSON function to read and write JSON type fields.
- Write non-relational data
The following code shows how to write a new user information to the users table:
info := `{ "age": 23, "gender": "male", "email": "john@example.com" }` result, err := db.Exec("INSERT INTO users(name, info) VALUES(?, ?)", "John", info) if err != nil { panic(err) } fmt.Printf("New user id: %d ", result.LastInsertId())
In this example, we first define a JSON string containing user information. We then use the Exec function to execute the insert statement and pass the user information as parameters into the SQL statement. After the insertion is successful, we obtain and output the new user's ID.
- Reading non-relational data
Next, we will demonstrate how to read JSON data stored in non-relational fields. You can simply obtain a key-value pair in JSON using MySQL's JSON_EXTRACT function. The following code shows how to read all user information in the users table:
rows, err := db.Query("SELECT * FROM users") if err != nil { panic(err) } defer rows.Close() for rows.Next() { var id int var name string var info []byte err := rows.Scan(&id, &name, &info) if err != nil { panic(err) } var user map[string]interface{} err = json.Unmarshal(info, &user) if err != nil { panic(err) } fmt.Printf("ID: %d, Name: %s, Age: %f, Gender: %s, Email: %s ", id, name, user["age"], user["gender"], user["email"]) }
In this example, we first use the db.Query function to query all data in the users table. Then, we use the rows.Scan function to read the ID, name, and info fields of each row of data. Next, we convert the value of the info field into a user variable of type map[string]interface{} and use the json.Unmarshal function for parsing. Finally, we output the ID, Name, Age, Gender and Email of each user.
- Updating non-relational data
Finally, we demonstrate how to update data stored in non-relational fields. MySQL's JSON_SET function can be used to dynamically update a key-value pair in a JSON field. The following code shows how to update the user's email address to a new value:
result, err := db.Exec("UPDATE users SET info=JSON_SET(info, '$.email', ?) WHERE name=?", "john_doe@example.com", "John") if err != nil { panic(err) } rowsAffected, err := result.RowsAffected() if err != nil { panic(err) } fmt.Printf("Rows affected: %d ", rowsAffected)
In this example, we use the Exec function to execute the update statement and pass the new email address as a parameter to the SQL statement JSON_SET function. We use the WHERE clause to specify the name of the user to update. After the update is successful, we check and output the number of rows affected.
4. Summary
In the Go language, we can use the MySQL database to store non-relational data. By using JSON type fields, we can easily store and process data in JSON format. This article introduces methods and techniques for creating non-relational tables, connecting to MySQL databases, reading and writing non-relational data, as well as examples of using the JSON_EXTRACT and JSON_SET functions for data operations. They can help you better use MySQL to process non-relational data in practical applications.
The above is the detailed content of Use MySQL in Go language to implement non-relational data processing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
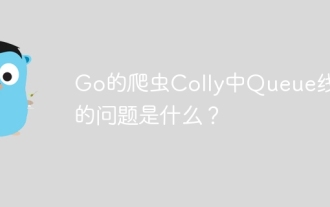
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
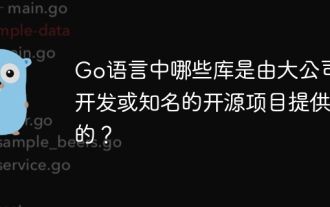
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
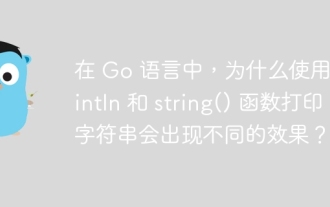
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
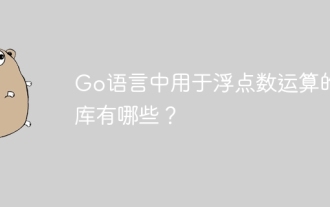
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
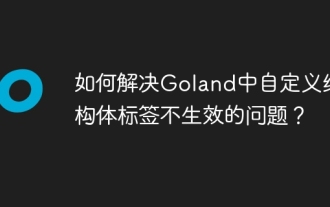
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
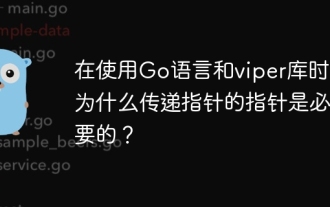
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
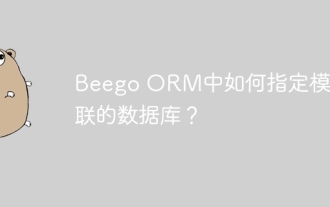
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
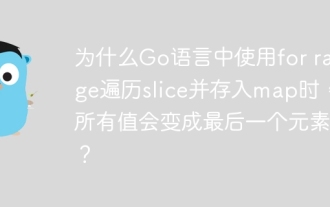
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
