


Python server programming: Using Paramiko to implement SSH remote operation
With the development of cloud computing and the Internet of Things, remote operation of servers has become more and more important. In Python, we can use the Paramiko module to easily implement SSH remote operations. In this article, we will introduce the basic usage of Paramiko and how to use Paramiko in Python to remotely manage servers.
What is Paramiko
Paramiko is a Python module for SSHv1 and SSHv2 that can be used to connect and control SSH clients and SSH servers. Using the Paramiko module, you can easily write Python scripts to remotely log in and operate the server.
Basic usage of Paramiko
The basic usage of Paramiko module is very simple. We can use Paramiko's SSHClient class to create an SSH client object and connect to the SSH server. Here is a basic example:
import paramiko ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('hostname', username='username', password='password')
In this example, we first create an SSHClient object and use the set_missing_host_key_policy method to allow missing SSH keys to be added automatically. We then connect to the SSH server using the connect method, passing in the hostname, username, and password as parameters.
Now, we have successfully connected to the SSH server. Next, we can use the SSHClient object to execute remote commands, such as obtaining the server's operating system information:
stdin, stdout, stderr = ssh.exec_command('uname -a') print(stdout.read())
In this example, we use the exec_command method to execute the uname -a command and obtain the output. We also used stdout.read() to read the output and print it.
In addition to executing remote commands, we can also use the SSHClient object to upload and download files. The following is an example of uploading files:
sftp = ssh.open_sftp() local_file = '/path/to/local/file.txt' remote_file = '/path/to/remote/file.txt' sftp.put(local_file, remote_file) sftp.close()
In this example, we first use the open_sftp method to open an SFTP session, and use the put method to upload local files to the remote server. We also used the sftp.close() method to close the SFTP session.
Use Paramiko to implement SSH remote operation
Now that we have understood the basic usage of Paramiko, next, we will use Paramiko to remotely operate the server. We will implement the following functionality:
- Connect to an SSH server and execute some basic commands
- Create and delete folders
- Upload and download files
First, we need to install the Paramiko module:
pip install paramiko
Next, we write a Python script. First, we import the necessary modules:
import os import paramiko
Then, we define some constants, including the hostname, username, and password of the SSH server:
HOST = 'hostname' USER = 'username' PASSWORD = 'password'
Next, we can use paramiko.SSHClient Class to connect to an SSH server:
ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(HOST, username=USER, password=PASSWORD)
In this example, we use the set_missing_host_key_policy() method to automatically add missing SSH keys.
Next, we will define some functions to perform different tasks. The first is the function that executes the command:
def run_command(command): stdin, stdout, stderr = ssh.exec_command(command) output = stdout.read().decode('utf-8') return output
This function receives a command parameter, uses the exec_command method of the SSHClient object to execute the command, and returns the output as a string.
Next, we define a function to create a directory:
def create_directory(path): sftp = ssh.open_sftp() try: sftp.mkdir(path) except IOError: pass sftp.close()
This function receives a path parameter and creates a directory using an SFTP session.
We also define functions to upload and download files:
def upload_file(local_path, remote_path): sftp = ssh.open_sftp() sftp.put(local_path, remote_path) sftp.close() def download_file(remote_path, local_path): sftp = ssh.open_sftp() sftp.get(remote_path, local_path) sftp.close()
These functions receive a local path and remote path (for uploading) or a remote path and local path (for downloading) respectively ), and use an SFTP session to upload or download the corresponding files.
Finally, we will define a main function to perform all tasks:
def main(): # Run a command output = run_command('ls -la') print(output) # Create a directory create_directory('/home/username/new_folder') # Upload a file upload_file('/path/to/local/file.txt', '/home/username/new_folder/file.txt') # Download a file download_file('/home/username/new_folder/file.txt', '/path/to/local/file.txt') # Close the SSH connection ssh.close()
This main function performs the following tasks:
- Execute the ls -la command and print Output
- Created a new directory
- Uploaded a file
- Downloaded the same file
- Closed SSH connection
Now, we can run this script and view the output:
python ssh.py
The result should be something like the following:
total 20 drwxr-xr-x 1 username username 4096 Aug 15 02:24 . drwxr-xr-x 1 username username 4096 Aug 15 02:20 .. -rw------- 1 username username 1675 Aug 15 02:21 .bash_history -rw-r--r-- 1 username username 220 Aug 15 02:20 .bash_logout -rw-r--r-- 1 username username 3771 Aug 15 02:20 .bashrc drwx------ 1 username username 4096 Aug 15 02:20 .cache drwxr-xr-x 1 username username 4096 Aug 15 02:20 .config drwx------ 1 username username 4096 Aug 15 02:20 .gnupg -rw-r--r-- 1 username username 807 Aug 15 02:20 .profile -rw-r--r-- 1 username username 0 Aug 15 02:21 test.txt
Summary
SSH remote can be easily achieved using the Paramiko module operate. In this article, we introduced the basic usage of Paramiko and demonstrated how to use Paramiko in Python to remotely manage servers. Whether in the field of cloud computing or the Internet of Things, Paramiko is a very useful tool that can help us automate server management.
The above is the detailed content of Python server programming: Using Paramiko to implement SSH remote operation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


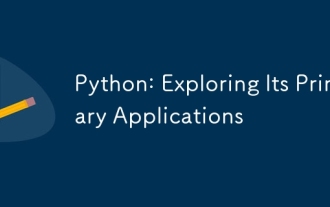
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
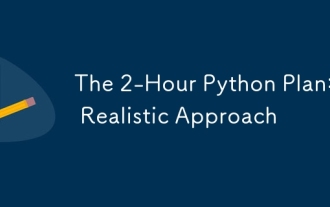
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
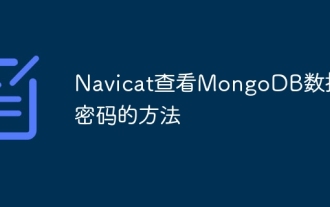
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
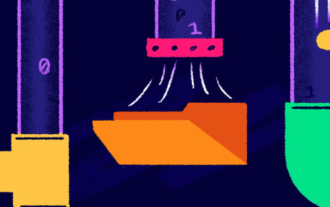
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
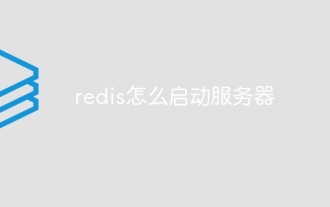
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
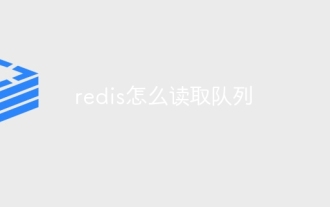
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
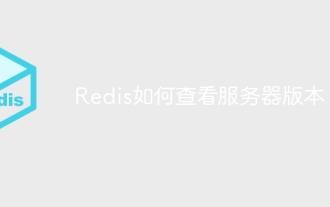
Question: How to view the Redis server version? Use the command line tool redis-cli --version to view the version of the connected server. Use the INFO server command to view the server's internal version and need to parse and return information. In a cluster environment, check the version consistency of each node and can be automatically checked using scripts. Use scripts to automate viewing versions, such as connecting with Python scripts and printing version information.
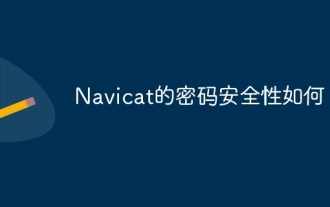
Navicat's password security relies on the combination of symmetric encryption, password strength and security measures. Specific measures include: using SSL connections (provided that the database server supports and correctly configures the certificate), regularly updating Navicat, using more secure methods (such as SSH tunnels), restricting access rights, and most importantly, never record passwords.
