Python Server Programming: Computer Vision with OpenCV
With the rapid development of computer technology, the field of computer vision has become an area of concern for more and more Python programmers. This article will introduce how to use Python and the OpenCV framework to build a computer vision server to implement some basic image processing functions.
- Installing OpenCV
To use OpenCV for computer vision development, you first need to install the OpenCV library in Python. There are many installation methods. Here is a relatively simple method:
Enter the following command on the command line:
pip install opencv-python
In addition, you can also choose to install other OpenCV modules, such as:
pip install opencv-contrib-python
- Implementing image processing functions
Next, we will use OpenCV to implement some basic image processing functions, such as:
- Read Image file
- Display image
- Convert image format
- Crop image
- Scale image
- Filter image
- Image Convert to grayscale
The following is the code implementation:
import cv2 # 读取图像文件 img = cv2.imread("test.jpg") # 显示图像 cv2.imshow("Original Image", img) # 转换图像格式 gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 显示灰度图像 cv2.imshow("Gray Image", gray_img) # 裁剪图像 cropped_img = img[100:400, 200:500] # 显示裁剪后的图像 cv2.imshow("Cropped Image", cropped_img) # 缩放图像 resized_img = cv2.resize(img, (800, 600)) # 显示缩放后的图像 cv2.imshow("Resized Image", resized_img) # 滤波图像 blur_img = cv2.GaussianBlur(img, (5, 5), 0) # 显示滤波后的图像 cv2.imshow("Blurred Image", blur_img) cv2.waitKey(0) cv2.destroyAllWindows()
This code will read the image file named "test.jpg" and implement the above basic image processing functions.
- Building a computer vision server based on Flask
Next, we will use Flask, the Python web framework, to build a simple computer vision server to integrate the above image processing functions Encapsulated in the form of API interface.
The following is the code implementation:
from flask import Flask, jsonify, request import cv2 app = Flask(__name__) @app.route('/') def index(): return "Welcome to the Computer Vision Server!" @app.route('/api/gray', methods=['POST']) def gray(): # 读取上传的图像文件 img_file = request.files['image'] img = cv2.imdecode(np.fromstring(img_file.read(), np.uint8), cv2.IMREAD_COLOR) # 转换图像格式为灰度 gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 将灰度图像转换为PNG格式,便于在web上显示 _, encoded_img = cv2.imencode('.png', gray_img) response = {'image': encoded_img.tobytes()} return jsonify(response) @app.route('/api/resize', methods=['POST']) def resize(): # 读取上传的图像文件 img_file = request.files['image'] img = cv2.imdecode(np.fromstring(img_file.read(), np.uint8), cv2.IMREAD_COLOR) # 获取传递的参数 width = int(request.form['width']) height = int(request.form['height']) # 缩放图像 resized_img = cv2.resize(img, (width, height)) # 将缩放后的图像转换为PNG格式,便于在web上显示 _, encoded_img = cv2.imencode('.png', resized_img) response = {'image': encoded_img.tobytes()} return jsonify(response) if __name__ == "__main__": app.run(debug=True, host='0.0.0.0')
Run the code and start the server.
- Test Server
We can use POST requests to test the API interface we just built on the server.
For example, we can use Postman to send an image to the server and call the "/api/gray" interface to convert the image to grayscale.
Similarly, we can also call the "/api/resize" interface to scale the image.
Through the above method, we can easily test the API interface of the computer vision server and realize the image processing function on the server side.
- Summary
This article introduces how to build a computer vision server using Python and the OpenCV framework. By encapsulating basic image processing functions into API interfaces, users can easily call these functions on the web. At the same time, computer vision technology has a wide range of application scenarios, and we can apply these technologies in many fields, such as image recognition, intelligent monitoring, etc.
The above is the detailed content of Python Server Programming: Computer Vision with OpenCV. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Object detection is an important task in the field of computer vision, used to identify objects in images or videos and locate their locations. This task is usually divided into two categories of algorithms, single-stage and two-stage, which differ in terms of accuracy and robustness. Single-stage target detection algorithm The single-stage target detection algorithm converts target detection into a classification problem. Its advantage is that it is fast and can complete the detection in just one step. However, due to oversimplification, the accuracy is usually not as good as the two-stage object detection algorithm. Common single-stage target detection algorithms include YOLO, SSD and FasterR-CNN. These algorithms generally take the entire image as input and run a classifier to identify the target object. Unlike traditional two-stage target detection algorithms, they do not need to define areas in advance, but directly predict
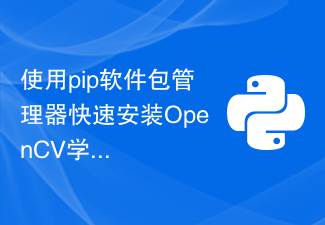
Use the pip command to easily install OpenCV tutorial, which requires specific code examples. OpenCV (OpenSource Computer Vision Library) is an open source computer vision library. It contains a large number of computer vision algorithms and functions, which can help developers quickly build image and video processing related applications. Before using OpenCV, we need to install it first. Fortunately, Python provides a powerful tool pip to manage third-party libraries
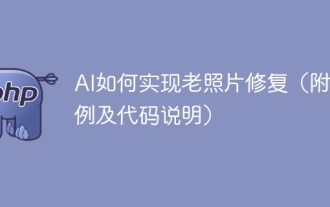
Old photo restoration is a method of using artificial intelligence technology to repair, enhance and improve old photos. Using computer vision and machine learning algorithms, the technology can automatically identify and repair damage and flaws in old photos, making them look clearer, more natural and more realistic. The technical principles of old photo restoration mainly include the following aspects: 1. Image denoising and enhancement. When restoring old photos, they need to be denoised and enhanced first. Image processing algorithms and filters, such as mean filtering, Gaussian filtering, bilateral filtering, etc., can be used to solve noise and color spots problems, thereby improving the quality of photos. 2. Image restoration and repair In old photos, there may be some defects and damage, such as scratches, cracks, fading, etc. These problems can be solved by image restoration and repair algorithms
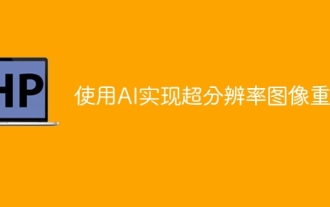
Super-resolution image reconstruction is the process of generating high-resolution images from low-resolution images using deep learning techniques, such as convolutional neural networks (CNN) and generative adversarial networks (GAN). The goal of this method is to improve the quality and detail of images by converting low-resolution images into high-resolution images. This technology has wide applications in many fields, such as medical imaging, surveillance cameras, satellite images, etc. Through super-resolution image reconstruction, we can obtain clearer and more detailed images, which helps to more accurately analyze and identify targets and features in images. Reconstruction methods Super-resolution image reconstruction methods can generally be divided into two categories: interpolation-based methods and deep learning-based methods. 1) Interpolation-based method Super-resolution image reconstruction based on interpolation
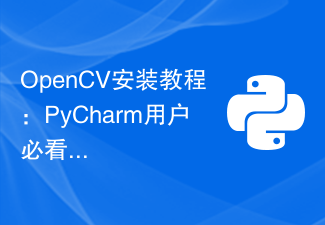
OpenCV is an open source library for computer vision and image processing, which is widely used in machine learning, image recognition, video processing and other fields. When developing using OpenCV, in order to better debug and run programs, many developers choose to use PyCharm, a powerful Python integrated development environment. This article will provide PyCharm users with an installation tutorial for OpenCV, with specific code examples. Step One: Install Python First, make sure you have Python installed

The Scale Invariant Feature Transform (SIFT) algorithm is a feature extraction algorithm used in the fields of image processing and computer vision. This algorithm was proposed in 1999 to improve object recognition and matching performance in computer vision systems. The SIFT algorithm is robust and accurate and is widely used in image recognition, three-dimensional reconstruction, target detection, video tracking and other fields. It achieves scale invariance by detecting key points in multiple scale spaces and extracting local feature descriptors around the key points. The main steps of the SIFT algorithm include scale space construction, key point detection, key point positioning, direction assignment and feature descriptor generation. Through these steps, the SIFT algorithm can extract robust and unique features, thereby achieving efficient image processing.
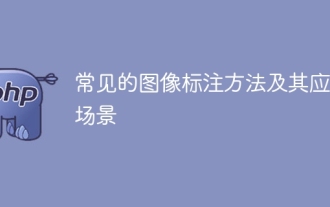
In the fields of machine learning and computer vision, image annotation is the process of applying human annotations to image data sets. Image annotation methods can be mainly divided into two categories: manual annotation and automatic annotation. Manual annotation means that human annotators annotate images through manual operations. This method requires human annotators to have professional knowledge and experience and be able to accurately identify and annotate target objects, scenes, or features in images. The advantage of manual annotation is that the annotation results are reliable and accurate, but the disadvantage is that it is time-consuming and costly. Automatic annotation refers to the method of using computer programs to automatically annotate images. This method uses machine learning and computer vision technology to achieve automatic annotation by training models. The advantages of automatic labeling are fast speed and low cost, but the disadvantage is that the labeling results may not be accurate.
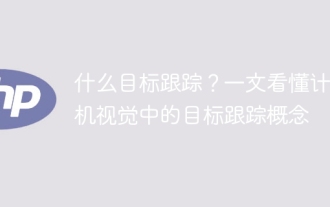
Object tracking is an important task in computer vision and is widely used in traffic monitoring, robotics, medical imaging, automatic vehicle tracking and other fields. It uses deep learning methods to predict or estimate the position of the target object in each consecutive frame in the video after determining the initial position of the target object. Object tracking has a wide range of applications in real life and is of great significance in the field of computer vision. Object tracking usually involves the process of object detection. The following is a brief overview of the object tracking steps: 1. Object detection, where the algorithm classifies and detects objects by creating bounding boxes around them. 2. Assign a unique identification (ID) to each object. 3. Track the movement of detected objects in frames while storing relevant information. Types of Target Tracking Targets
