Computed property functions in Vue3: Let you write more elegant code
In Vue.js, we often use computed property functions to help us manage data and render specific content in templates. In Vue.js version 3, the functions of computed property functions have become more powerful and flexible, allowing us to write more expressive and elegant code.
Computed properties are properties calculated based on one or more reactive dependencies in the Vue instance. When a reactive dependency is updated, a computed property recalculates its value and is therefore reactive. The syntax of a computed property is as follows:
computed: { propName: function() { // return value based on reactive dependencies } }
In Vue.js 3, the computed property function can be used in the following two ways:
- Through the
computed
method Defining computed property functions
Defining computed property functions is easier using the computed
function in Vue.js 3. It can receive a function as a parameter and return a reactive reference that automatically calculates and updates its value.
For example, suppose we have a component that needs to define a computed property by calculating the sum of two variables. In Vue.js 3, we can write like this:
<template> <div>{{ sum }}</div> </template> <script> import { computed } from 'vue'; export default { setup() { const num1 = 2; const num2 = 3; const sum = computed(() => { return num1 + num2; }); return { sum }; } } </script>
In the above example, we used the computed
function in Vue.js 3 to define the calculated property sum
. computed
The function can receive a function as a parameter, which uses Vue.js 3's reactive API to calculate the value of the calculated property. We define two variables num1
and num2
, and then use arrow functions to return their sum. Finally, we return the sum
computed property in the component options.
- Use
ref
andwatch
functions to define computed property functions
Another common use in Vue.js 3 The way to calculate attribute functions is to use the ref
and watch
functions. In this case, we can first use the ref
function to define the starting value of the calculated property function, and then use the watch
function to define the calculation logic and its responsive dependencies of the calculated property function. .
<template> <div>{{ sum }}</div> </template> <script> import { ref, watch } from 'vue'; export default { setup() { const num1 = ref(2); const num2 = ref(3); const sum = ref(num1.value + num2.value); watch([num1, num2], () => { sum.value = num1.value + num2.value; }); return { sum }; } } </script>
In the above example, we first use the ref
function to define the responsive variables num1
and num2
, assigning values to 2 and 2 respectively. 3. Then, we define the reactive variable sum
again using the ref
function and set its initial value to num1.value num2.value
.
Next, we use the watch
function of Vue.js 3 to monitor changes in num1
and num2
. When num1# When the values of ## and
num2 change, the
watch function will automatically update the value of
sum. We use
sum.value to update the value of the calculated property to ensure it updates responsively into our template.
computed function or the
ref and
watch functions to define computed property functions, which allows us to write more elegant and expressive code. Using Vue.js 3’s computed property functions allows us to take fuller advantage of Vue.js’s reactive system and improve the maintainability and reusability of our applications.
The above is the detailed content of Computed property functions in Vue3: Let you write more elegant code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
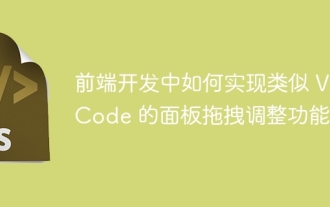
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
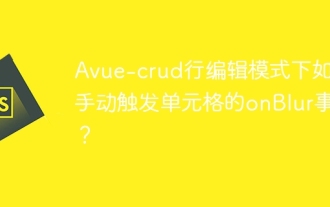
The onBlur event that implements Avue-crud row editing in the Avue component library manually triggers the Avue-crud component. It provides convenient in-line editing functions, but sometimes we need to...
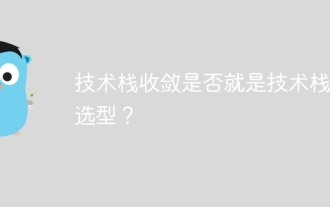
Title: The relationship between technology stack convergence and selection: Does technology stack convergence refer to the selection of technology stack? I saw an article that has a convergence technology stack...
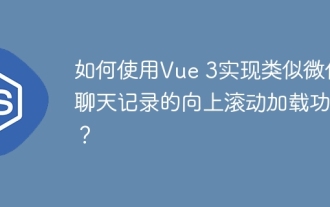
How to achieve upward scrolling loading similar to WeChat chat records? When developing applications similar to WeChat chat records, a common question is how to...
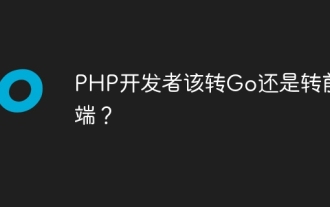
Career choices for PHP developers: to switch to Go or to front-end? In the modern software development industry, the selection of technology stacks and the planning of career development paths are for...
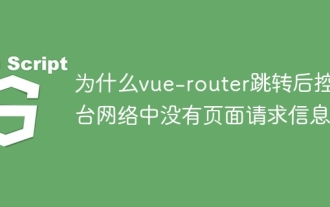
Why is there no page request information on the console network after vue-router jump? When using vue-router for page redirection, you may notice a...
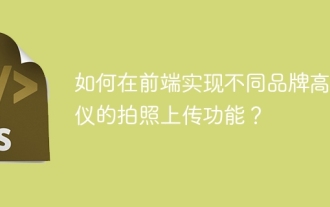
How to implement the photo upload function of different brands of high-photographers on the front end When developing front-end projects, you often encounter the need to integrate hardware equipment. for...
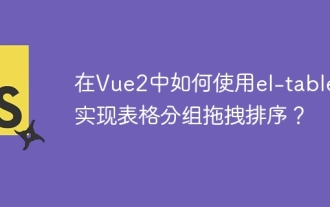
Implementing el-table table group drag and drop sorting in Vue2. Using el-table tables to implement group drag and drop sorting in Vue2 is a common requirement. Suppose we have a...
