Python Server Programming: Audio Processing with PyAudio
Python is a powerful programming language that can be used for everything from simple scripts to complex applications and servers. PyAudio is a popular audio processing library in Python that can be used to record, play and process audio data.
In this article, we will explore how to use PyAudio to develop a Python server for processing audio data. We will introduce the basic concepts and API of PyAudio and how to use it to process audio data. We will also demonstrate how to use PyAudio with a Python server framework.
Basic knowledge
Before starting to use PyAudio, we need to understand some basic knowledge.
Audio sampling rate
The audio sampling rate refers to the number of times a sound is sampled in one second. The higher the sample rate, the better the audio quality. Common audio sampling rates are 44.1kHz and 48kHz.
Audio bit depth
Audio bit depth refers to the precision with which each sample is stored. The higher the bit depth, the better the audio quality. Common bit depths are 16-bit and 24-bit.
Number of audio channels
The number of audio channels refers to the number of channels for recording audio signals. Single-channel (mono) audio has only one channel, two-channel (stereo) audio has two channels, and multi-channel audio has more than two channels.
PyAudio API
PyAudio's API defines a set of functions and constants that can be used to record, play and process audio data. The following are some important functions and constants:
pyaudio.PyAudio()
This is a constructor used to create a PyAudio instance. This instance can be used to access other PyAudio functions.
pyaudio.paInt16
This is a constant representing the 16-bit audio data type. You can use other constants to specify different audio data types.
pyaudio.paFloat32
This is a constant representing the 32-bit floating point audio data type. This data type is commonly used in audio signal processing.
PyAudio.open()
This function is used to open the audio stream. It returns a PyAudio stream object.
stream.read()
This function is used to read data from the audio stream.
stream.write()
This function is used to write data to the audio stream.
Example
The following is a simple Python program that uses PyAudio to record audio and save it to a file:
import pyaudio import wave chunk = 1024 FORMAT = pyaudio.paInt16 CHANNELS = 2 RATE = 44100 RECORD_SECONDS = 5 WAVE_OUTPUT_FILENAME = "output.wav" p = pyaudio.PyAudio() stream = p.open(format=FORMAT, channels=CHANNELS, rate=RATE, input=True, frames_per_buffer=chunk) print("* recording") frames = [] for i in range(0, int(RATE / chunk * RECORD_SECONDS)): data = stream.read(chunk) frames.append(data) print("* done recording") stream.stop_stream() stream.close() p.terminate() wf = wave.open(WAVE_OUTPUT_FILENAME, 'wb') wf.setnchannels(CHANNELS) wf.setsampwidth(p.get_sample_size(FORMAT)) wf.setframerate(RATE) wf.writeframes(b''.join(frames)) wf.close()
The above code uses PyAudio to open an audio stream and save it from the stream Read data in. It also uses the wave library to create a WAV file and writes the read data to the file.
Conclusion
In this article, we introduced how to use PyAudio for audio processing. We learned the basic concepts and API of PyAudio, and demonstrated how to create a Python server to process audio data. You should now be familiar with how to use PyAudio to develop Python applications and servers with audio processing capabilities.
The above is the detailed content of Python Server Programming: Audio Processing with PyAudio. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


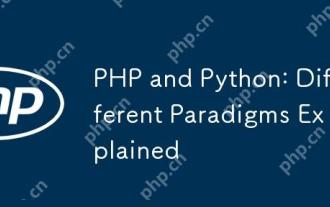
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
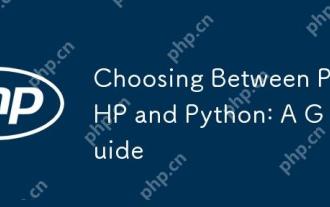
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
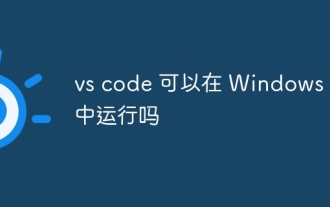
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
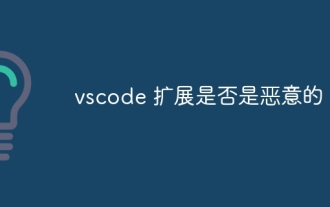
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
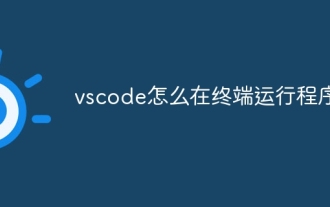
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
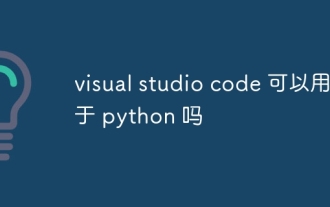
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
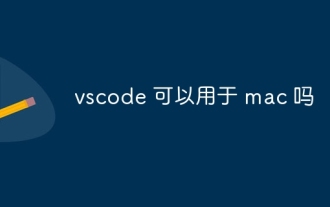
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
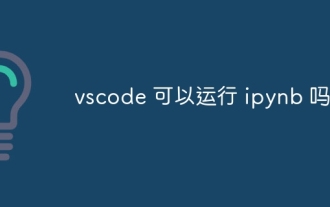
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
