Detailed explanation of asynchronous task processing in Redis
As web applications continue to develop, the need for asynchronous task processing is becoming more and more important, because we need to ensure that users can continue to use the application before completing the task. In this case, except for asynchronous task processing, multi-task parallel processing cannot be achieved, so it is often necessary to use some tools to handle asynchronous tasks, of which Redis is a very useful tool.
Redis is a high-performance in-memory database that can be used to quickly store, read and operate data. Its main use is to implement caching and messaging, however, it can also be used to handle asynchronous tasks. Redis has built-in queuing and publish/subscribe capabilities, which makes it a very useful tool for asynchronous task processing.
In this article, we will introduce how to use Redis to implement asynchronous task processing.
- Establishing a Redis connection
First, we need to use a Redis client to establish a connection with the Redis server. Any client that supports Redis connections can be used. Python's redis-py is a very good choice. Please make sure to install redis-py globally:
pip install redis
Next, you can use the following command to establish a Redis connection:
import redis redis_conn = redis.Redis(host='localhost', port=6379, db=0)
Here we have created a Redis connection instance named redis_conn, which will Connect to the local Redis server (host='localhost'), the port number is 6379 (port=6379), and use database No. 0 (db=0).
- Redis Queue
Redis Queue (RQ) is a Python library that uses Redis as the backend to implement a distributed task queue. RQ is built on Redis's lpush and rpop commands, so it has very good performance.
Install RQ and Redis:
pip install rq redis
- Synchronization task
In a synchronization task, the main thread will execute all code and wait for the task to complete. The following is a sample code for a synchronized task:
import time def task(): # 等待5秒 time.sleep(5) print('Task complete') print('Starting task') task() print('Task ended')
In the above example, we defined a function named task that waits for 5 seconds and then outputs "Task complete". Then we call this task in the main thread, output "Starting task", wait for 5 seconds, and output "Task ended".
This approach is feasible for short-lived tasks, but for long-running tasks it can leave users very unsatisfied because they cannot use the application.
Now, let's see how to convert this task into an asynchronous task.
- Asynchronous Task
The idea of converting a task into an asynchronous task is to execute the task in a separate thread or process and continue to execute other code in the main thread . This way, the user can continue to use the application while tasks are performed in the background.
In Python, you can use threads or processes to perform background tasks. But if multiple tasks are running, the number of threads and processes may increase, and they may also develop problems, such as deadlocks and synchronization issues.
Using Redis can solve this problem, because Redis has a built-in queue structure that allows us to avoid these problems. The basic idea of implementing asynchronous tasks in Redis is to create a task queue and add tasks to the queue. Then create a separate task executor to get the tasks in the queue and execute them.
Since Redis is an in-memory database, you can use it to store all queue data. This way we can store task status in Redis and don't need to use threads or processes to handle tasks.
The following is a sample code for an asynchronous task:
from rq import Queue from redis import Redis redis_conn = Redis() q = Queue(connection=redis_conn) def task(): # 等待5秒 time.sleep(5) print('Task complete') print('Starting task') job = q.enqueue(task) print('Task started')
In the above code, we first create a Redis queue named q, and then define a function named task. When calling a task in the main thread, we add the task to the queue using the enqueue method of the queue object. This method returns a task object named job, which represents the task in the queue. Then we output "Task started" and the queue executor will get the task in the background and execute it.
- Monitoring Tasks
In the previous example, we could use the job object to monitor the task status and retrieve the results. Here is the sample code on how to monitor a task:
from rq import Queue from redis import Redis redis_conn = Redis() q = Queue(connection=redis_conn) def task(): # 等待5秒 time.sleep(5) return 'Task complete' print('Starting task') job = q.enqueue(task) print('Task started') # 检查任务状态并获取结果 while job.result is None: print('Task still processing') time.sleep(1) print('Task complete: {}'.format(job.result))
In the above code, we check the result property of the task until it is not empty. We then output "Task complete:" plus the result of the task object.
- Using Publish/Subscribe
Redis also supports a publish/subscribe (pub/sub) model, which makes it a very useful messaging tool. In this model, a publisher publishes messages to a topic, and subscribers subscribe to the topic and receive all messages on the topic.
Let us take an asynchronous task as an example to illustrate the implementation using the publish/subscribe model.
First, we need to create a unique ID for each task and add the task to the queue. We then publish the task ID into the topic. The task executor subscribes to the topic and when a task ID is received, it gets the task and executes it.
The following is a sample code to implement an asynchronous task using the publish/subscribe model:
from rq import Queue from redis import Redis import uuid redis_conn = Redis() q = Queue(connection=redis_conn) # 订阅任务主题并执行任务 def worker(): while True: _, job_id = redis_conn.blpop('tasks') job = q.fetch_job(job_id.decode('utf-8')) job.perform() # 发布任务并将其ID添加到队列中 def enqueue_task(): job = q.enqueue(task) redis_conn.rpush('tasks', job.id) def task(): # 等待5秒 time.sleep(5) return 'Task complete' print('Starting workers') for i in range(3): # 创建3个工作线程 threading.Thread(target=worker).start() print('Enqueueing task') enqueue_task() print('Task enqueued')
In the above code, we first define a task executor named worker, which continuously Loop and cancel the scheduled task ID from the queue. When it gets the task ID, it uses the fetch_job method to get the task object and execute it.
We also define a function called enqueue_task, which creates an asynchronous task named job and adds its ID to the queue. We then call this function in the main thread and publish the task ID to a topic called "tasks". The task executor will get the task and execute it when it receives the task ID.
- Summary
In this article, we introduced how to use Redis to implement asynchronous task processing. We used queues, the publish/subscribe model, and the RQ library in python, while showing how to convert tasks into asynchronous mode and use asynchronous tasks to solve user experience problems. Redis is very useful when handling asynchronous tasks as it provides built-in queuing and publish/subscribe functionality with very good performance. If you want to make your web application responsive and implement asynchronous task processing, Redis is a good choice.
The above is the detailed content of Detailed explanation of asynchronous task processing in Redis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


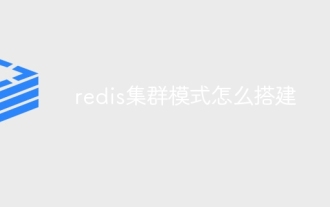
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make

Redis uses hash tables to store data and supports data structures such as strings, lists, hash tables, collections and ordered collections. Redis persists data through snapshots (RDB) and append write-only (AOF) mechanisms. Redis uses master-slave replication to improve data availability. Redis uses a single-threaded event loop to handle connections and commands to ensure data atomicity and consistency. Redis sets the expiration time for the key and uses the lazy delete mechanism to delete the expiration key.
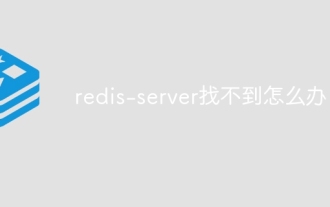
Steps to solve the problem that redis-server cannot find: Check the installation to make sure Redis is installed correctly; set the environment variables REDIS_HOST and REDIS_PORT; start the Redis server redis-server; check whether the server is running redis-cli ping.
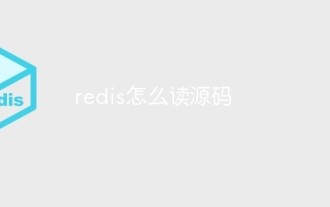
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.
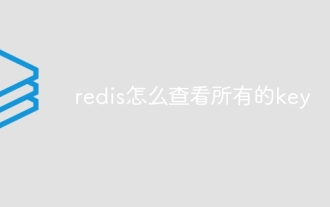
To view all keys in Redis, there are three ways: use the KEYS command to return all keys that match the specified pattern; use the SCAN command to iterate over the keys and return a set of keys; use the INFO command to get the total number of keys.
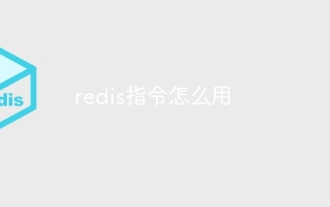
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
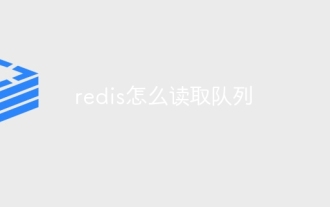
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
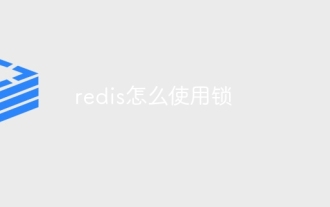
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
