


Implementation method of component parent-child value transfer function in Vue document
Vue is a popular JavaScript framework. Its component development can help us improve the degree of modularity when developing complex applications and improve the maintainability and scalability of the code. In Vue, data transfer between components is a very common requirement, and the most common scenario is data transfer between parent and child components. In order to implement this kind of data transfer in Vue, we need to understand the implementation method of value transfer between parent and child components.
In Vue components, a parent component can have multiple child components at the same time. The parent component can pass data to the child components. These data can be the data of the parent component or the return of the function of the parent component calling the child component. value. Specifically, in Vue, there are several ways to implement component parent-child value transfer:
- Props transfer data
In Vue, we can declare the props attribute in the child component, and then Data is passed from the parent component to the child component in the form of properties. Child components can receive this data using properties declared in the props attribute.
Sample code:
Parent component:
<template> <div> <child-component :msg="helloWorld"></child-component> </div> </template> <script> import ChildComponent from './ChildComponent.vue' export default { data() { return { helloWorld: 'Hello World!' } }, components: { ChildComponent } } </script>
Child component:
<template> <div> {{msg}} </div> </template> <script> export default { props: { msg: String } } </script>
In this example, we declared in the child component A props attribute, its name is msg and its type is String. When using a child component in a parent component, we use the v-bind directive to bind the helloWorld attribute in the parent component to the msg attribute of the child component.
- The child component passes data to the parent component through the $emit event
In Vue, we can pass data to the parent component by triggering events. The child component can trigger a custom event by calling the $emit function, and the data that needs to be passed can be passed to the parent component as event parameters.
Sample code:
Parent component:
<template> <div> <child-component @message-sent="showMessage"></child-component> </div> </template> <script> import ChildComponent from './ChildComponent.vue' export default { methods: { showMessage(msg) { console.log(msg) } }, components: { ChildComponent } } </script>
Child component:
<template> <div> <button @click="sendMessage">Click Me</button> </div> </template> <script> export default { methods: { sendMessage() { this.$emit('message-sent', 'Hello World!') } } } </script>
In this example, we add to the child component A button. When the button is clicked, the child component calls the sendMessage function, triggers a custom event named message-sent, and passes the parameter 'Hello World!' to the parent component.
- Get the subcomponent instance through the ref attribute
In Vue, we can get the instance of the subcomponent by adding the ref attribute to it, and then directly call the methods and properties on this instance.
Sample code:
Parent component:
<template> <div> <button @click="showMessage">Click Me</button> <child-component ref="child"></child-component> </div> </template> <script> import ChildComponent from './ChildComponent.vue' export default { methods: { showMessage() { console.log(this.$refs.child.message) } }, components: { ChildComponent } } </script>
Child component:
<template> <div> {{message}} </div> </template> <script> export default { data() { return { message: 'Hello World!' } } } </script>
In this example, we define in the child component A data attribute message, and the instance of the child component is obtained through the ref attribute in the parent component. When the button is clicked, the parent component obtains the instance of the child component through this.$refs.child, and then directly accesses the message property on it.
Summary:
The above are some common ways to implement component parent-child value transfer in Vue. Among them, Props is the most commonly used method of transmitting data. It can make the data transfer type between components clear and has better readability and maintainability; while the method of transmitting data to the parent component through the $emit event is applicable It is suitable for scenarios where operations or data need to be performed within sub-components but cannot be achieved through props. The method of obtaining sub-component instances through ref attributes is suitable for situations where the parent component needs to directly operate sub-component data or functions.
The above is the detailed content of Implementation method of component parent-child value transfer function in Vue document. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


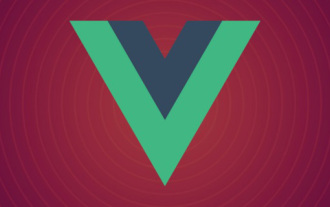
Value passing method: 1. Use props to pass from parent to child; 2. From child to parent, you need to customize the event. Use "this.$emit('event name')" to trigger it in the child component, and use "@event" in the parent. name" monitoring; 3. Between brothers, use the public parent element as a bridge, combining parent and child props to pass parameters, and child and parent custom events; 4. Use routing to pass values; 5. Use $ref to pass values; 6. Use dependency injection to pass Descendants and great-grandchildren; 7. Use $attrs; 8. Use $listeners intermediate events; 9. Use $parent to pass, etc.
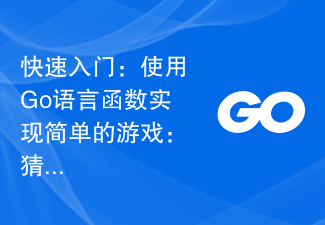
Quick Start: Use Go language functions to implement a simple game: guess numbers. With the popularity and development of computer programming, learning a programming language has become the goal of many people. As a simple, easy-to-learn and powerful programming language, Go language is favored by more and more developers. This article will introduce how to use Go language functions to implement a simple game: guess the number. By writing this small game, we can better understand and learn the basic syntax of Go language and the use of functions. Step 1: Import the necessary packages before starting to compile
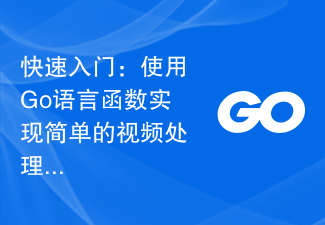
Quick Start: Use Go language functions to implement simple video processing functions Introduction: In today's digital era, video has become an indispensable part of our lives. However, processing videos requires efficient algorithms and powerful computing power. As a simple and efficient programming language, Go language also shows its unique advantages in video processing. This article will introduce how to use Go language functions to implement simple video processing functions, and use code examples to let everyone understand the process more intuitively. Step 1: Import related packages for video processing
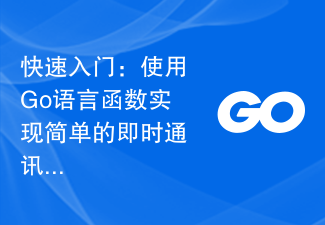
Quick Start: Using Go language functions to implement a simple instant messaging chat function Introduction: In today's highly interconnected society, instant messaging has become an indispensable part of people's daily lives. This article will introduce how to use Go language functions to implement a simple instant messaging chat function. Using Go language functions can simplify code writing and improve programming efficiency, allowing us to quickly get started and develop a powerful instant messaging system. I. Preparation work Before starting to write code, we need to understand some things about Go language
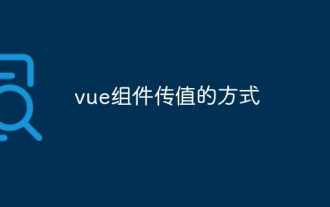
The way to pass value in vue components: 1. Pass from parent to son; 2. Pass from son to father; 3. Pass value from brother; 4. Pass value with question mark, colon and parent-child component; 5. Use "$ref" to pass value; 6. Use "inject" to inject the data of the parent component into the current instance; 7. Pass from ancestor to grandson; 8. Pass from grandson to ancestor; 9. $parent; 10. Pass value to sessionStorage; 11. vuex.
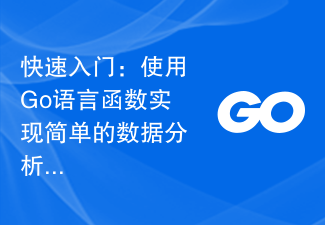
Quick Start: Use Go language functions to implement simple data analysis functions Overview: Data analysis is one of the important skills in modern society. With the advent of the big data era, it has become increasingly important to effectively analyze and extract value from data. As an efficient and concise programming language, Go language has the ability to handle data analysis tasks. This article will introduce how to use Go language functions to implement simple data analysis functions. Data import Before performing data analysis, you first need to import the data into the Go program. Various methods can be used, such as reading

Quick Start: Use Go language functions to implement simple data visualization histogram display In the field of modern data science and analysis, data visualization is a very important skill. Through visualization, we can better understand and communicate data and discover meaningful patterns and trends. In this article, we will use Go language functions to implement a simple data visualization histogram display. First, we need to install the Go language development environment. You can find it on the Go official website (https://golang.org/)
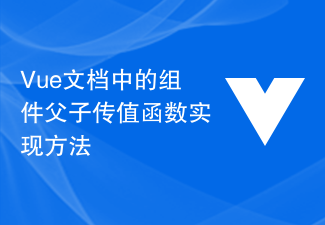
Vue is a popular JavaScript framework. Its component development can help us improve the degree of modularity when developing complex applications, and improve the maintainability and scalability of the code. In Vue, data transfer between components is a very common requirement, and the most common scenario is data transfer between parent and child components. In order to implement this kind of data transfer in Vue, we need to understand the implementation method of value transfer between parent and child components. In Vue components, a parent component can have multiple child components at the same time, and the parent component can
