


Practical tips on using cache to improve file system performance in Golang.
In recent years, with the continuous development of cloud computing, big data, artificial intelligence and other fields, the explosive growth of data volume has become an indisputable fact. Therefore, how to improve the access speed and performance of file systems has become a Problems that must be solved. In this context, the emergence of the Golang language has provided developers with more convenient and efficient tools to a certain extent. This article will combine practical experience to introduce some techniques for using cache to improve file system performance in Golang.
1. What is file system cache?
Before we explain the file system cache in depth, we must first understand what cache is. Caching is usually a technical means used to improve system performance. It stores frequently accessed data in high-speed memory so that the next time the data is needed, it can be read quickly, thereby reducing the need for slow memory ( Such as disk, network, etc.) to improve the response speed and efficiency of the system.
In the file system, there is also a caching mechanism, which focuses on improving the speed of file reading and writing. There are two main ways to implement file system caching: read caching and write caching.
Read cache: For read operations, the file system can use read cache to cache the read data blocks in the memory so that the data can be obtained directly from the memory during the next access without having to retrieve it from the disk. Read. This can reduce disk IO operations and thereby increase file access speed.
Write cache: For write operations, the file system can also use write cache to cache data in memory. Cached writes increase response time less significantly for applications and users, making writes to the file system faster and more efficient. Asynchronous flushing of the cache will reduce blocking in applications, increase throughput, and reduce the overhead of disk IO operations, thereby further improving file access speeds.
2. Implementation of Golang file system cache
In Golang’s standard library, the os package and bufio package have been provided to operate the file system, among which the bufio package implements caching IO, caching can be used to improve file system performance. However, for a large number of small files, or file operations with less frequent reads and writes, a more efficient cache implementation is required.
- Use sync.Map
sync.Map is a concurrent and safe map provided in Golang. Through the Range or Load, Store, Delete and other methods it provides, you can Perform cached read and write operations more efficiently. It can avoid problems such as data race conditions during read and write operations, thereby improving performance and security. Therefore, sync.Map is a good choice for implementing file system caching.
The following is a simple example code that uses sync.Map to implement file system caching:
package main import ( "fmt" "io/ioutil" "sync" ) var cache sync.Map func main() { data, _ := readData("test.txt") fmt.Println("Data:", string(data)) } func readData(path string) ([]byte, error) { // 先从缓存中查找 c, ok := cache.Load(path) if ok { return c.([]byte), nil } // 缓存中没有,则从磁盘中读取 data, err := ioutil.ReadFile(path) if err != nil { return nil, err } // 保存到缓存中 cache.Store(path, data) return data, nil }
In the above code, the readData function first looks up the data from the cache. If it exists in the cache, then Return directly; otherwise read data from disk and save it to cache.
- Use LRU cache
Although the above example uses cache, it does not consider the limit of cache capacity, resulting in all files being saved in the cache, which may occupy A lot of memory. Therefore, in order to avoid this situation, we can use the LRU (Least Recently Used) algorithm to implement a caching mechanism with capacity limitations. In the LRU algorithm, when the cache is full and a new data block needs to be inserted, the least recently used data will be eliminated first to ensure that the data in the cache are frequently accessed recently.
The following is a sample code using the LRU cache implementation:
package main import ( "fmt" "io/ioutil" "github.com/hashicorp/golang-lru" ) func main() { // 新建一个缓存,容量为50个文件 cache, _ := lru.New(50) // 从文件系统中读取数据 data, _ := readData("test.txt", cache) fmt.Println("Data:", string(data)) } func readData(path string, cache *lru.Cache) ([]byte, error) { // 先从缓存中查找 if c, ok := cache.Get(path); ok { return c.([]byte), nil } // 缓存中没有,则从磁盘中读取 data, err := ioutil.ReadFile(path) if err != nil { return nil, err } // 保存到缓存中 if cache.Len() >= cache.MaxLen() { cache.RemoveOldest() } cache.Add(path, data) return data, nil }
In the above sample code, we use the LRU implementation provided by the github.com/hashicorp/golang-lru library to save the cache . The cache capacity can be specified through the New method, and the Get, Add, and RemoveOldest methods can be used to implement cache reading, insertion, and elimination.
3. Conclusion
Through the above practices, we can see that using cache can effectively improve the speed and performance of file system access. In Golang, we can use sync.Map or LRU cache mechanism to achieve the effect of concurrency security and capacity limitation. Different scenarios can choose different implementation methods according to the actual situation. It is worth mentioning that the caching mechanism is not unique to Golang. Other languages also provide corresponding caching implementations. These general mechanisms and methods can be reused in multiple projects, improving development efficiency and code reuse. .
The above is the detailed content of Practical tips on using cache to improve file system performance in Golang.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
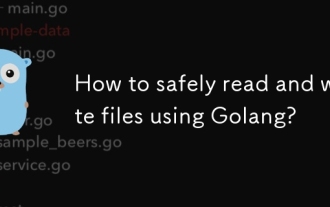
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
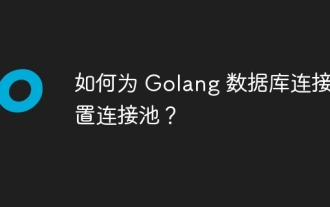
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
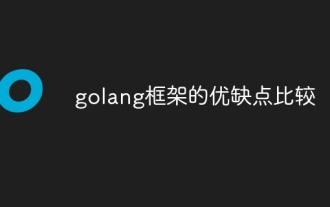
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
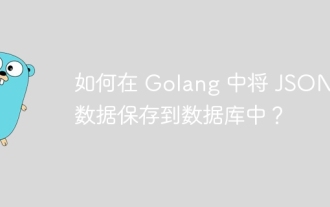
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
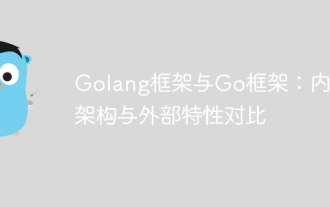
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
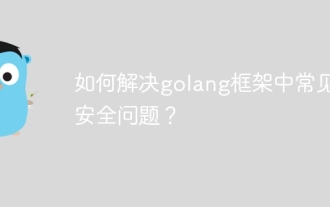
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
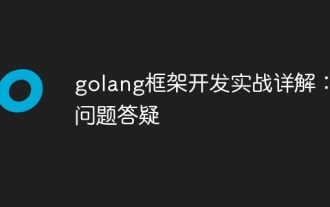
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
