Implementation principle of data response function in Vue documentation
Vue is a popular open source front-end framework that provides many functions that facilitate development, the most important of which is the data response mechanism. The data response mechanism is the core of Vue's two-way data binding and an important concept for developing Vue applications. This article will delve into the internal mechanism of Vue to implement data response.
Vue’s data response mechanism is implemented through Object.defineProperty. Object.defineProperty is a built-in function in JavaScript, mainly used to change the characteristics of properties. Different from the set and get functions in JavaScript, it can directly add properties to objects and assign some special properties to these properties. Vue uses Object.defineProperty to add getter and setter functions to objects to achieve data response.
At the same time, Vue treats the real data as data and the observer as watcher. In order to facilitate management, it also introduces an attribute dep. When an attribute in data is introduced into the view and a watcher instance is generated, the watcher will be added to dep, thus establishing the association between watcher and dep. When the data in data is changed, dep will be notified to call the watcher instance associated with it, and then call the callback function set before the watcher instance to achieve responsive updating of the data.
A simple code example is given below:
let data = { name: 'Vue' }; Object.defineProperty(data, 'name', { get() { console.log('get', data.name); return data.name; }, set(newValue) { console.log('set', newValue); data.name = newValue; } }); console.log(data.name); data.name = 'React'; console.log(data.name);
In the above code, we added a property named name to the data object through Object.defineProperty and defined the property The get and set functions.
When console.log(data.name) is executed, the get function of the name attribute will be called and "get Vue" will be output.
When data.name = 'React' is executed, the set function of the name attribute will be called, "set React" will be output, and then "get React" will be output.
The above is the implementation principle of Vue to implement data response. Through the association between Object.defineProperty and dep, watcher, and data, as well as the implementation of getter and setter functions, Vue implements effective responsive data updates, making it more convenient and efficient for developers to process data.
The above is the detailed content of Implementation principle of data response function in Vue documentation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
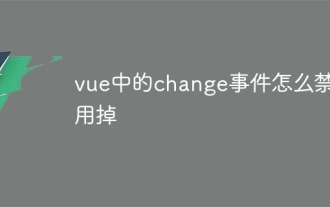
In Vue, the change event can be disabled in the following five ways: use the .disabled modifier to set the disabled element attribute using the v-on directive and preventDefault using the methods attribute and disableChange using the v-bind directive and :disabled
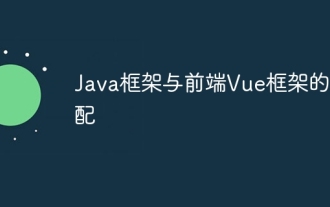
The Java framework and Vue front-end adaptation implement communication through the middle layer (such as SpringBoot), and convert the back-end API into a JSON format that Vue can recognize. Adaptation methods include: using the Axios library to send requests to the backend and using the VueResource plug-in to send simplified API requests.
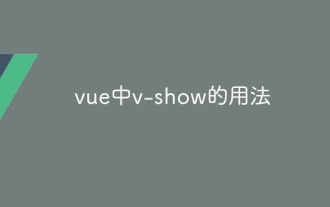
The v-show directive is used to dynamically hide or show elements in Vue.js. Its usage is as follows: The syntax of the v-show directive: v-show="booleanExpression", booleanExpression is a Boolean expression that determines whether the element is displayed. The difference with v-if: v-show only hides/shows elements through the CSS display property, which optimizes performance; while v-if conditionally renders elements and recreates them after destruction.
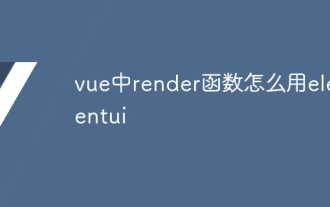
The render function is used to create the virtual DOM in a Vue.js application. In Element UI, you can integrate Element UI components into the render function by rendering the component directly, using JSX syntax, or using scopedSlots. When integrating, you need to import the Element UI library, set properties in kebab-case mode, and use scopedSlots to render slot content (if the component has slots).
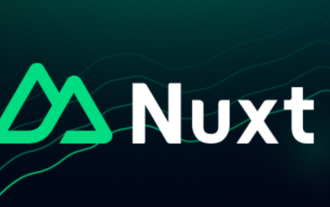
Nuxt is an opinionated Vue framework that makes it easier to build high-performance full-stack applications. It handles most of the complex configuration involved in routing, handling asynchronous data, middleware, and others. An opinionated director
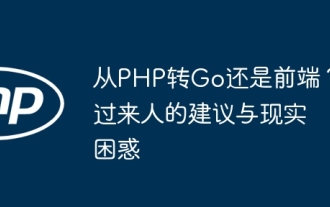
Confusion and the cause of choosing from PHP to Go Recently, I accidentally learned about the salary of colleagues in other positions such as Android and Embedded C in the company, and found that they are more...
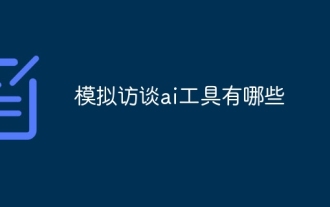
Mock interview AI tools are valuable tools for efficient candidate screening, saving recruiters time and effort. These tools include HireVue, Talview, Interviewed, iCIMS Video, and Eightfold AI. They provide automated, session-based assessments with benefits including efficiency, consistency, objectivity and scalability. When choosing a tool, recruiters should consider integrations, user-friendliness, accuracy, pricing, and support. Mock interviewing AI tools improve hiring speed, decision quality, and candidate experience.
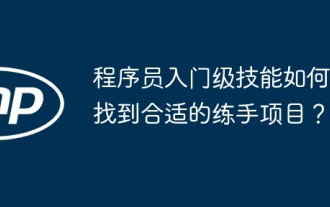
Programmers' "tickling" needs: From leisure to practice, this programmer friend has been a little idle recently and wants to improve his skills and achieve success through some small projects...
