


Caching algorithms: Detailed explanation of LRU, LFU, and FIFO algorithms in Java caching technology
In Java development, caching is a very important concept. Caching can improve the efficiency of data reading and writing, thereby improving the overall performance of the application. There are many caching algorithms, common ones include LRU, LFU and FIFO. The following is a detailed introduction to these three caching algorithms and their application scenarios.
1. LRU algorithm
The LRU algorithm is the least used recently. This algorithm means that if a piece of data has not been used in the recent period, the probability of it being used in the future period is very small. Therefore, when cache space is insufficient, the least recently used data should be deleted to free up space. The core of the LRU algorithm is to maintain a table of usage time, which can be implemented using a linked list or an array.
The following is a simple code implementation using the LRU algorithm in Java:
public class LRUCache<K, V> extends LinkedHashMap<K, V> { private final int CACHE_SIZE; public LRUCache(int cacheSize) { super((int)Math.ceil(cacheSize / 0.75f) + 1, 0.75f, true); CACHE_SIZE = cacheSize; } @Override protected boolean removeEldestEntry(Map.Entry eldest) { return size() > CACHE_SIZE; } }
2. LFU algorithm
The LFU algorithm is the least frequently used. LFU determines which data should be cached based on the historical access frequency of the data. In the LFU algorithm, each data has a counter to record the number of times it has been accessed. When the cache space is insufficient, the data with the lowest access frequency should be deleted to free up space. The core of the LFU algorithm is to maintain a counter table that records the number of accesses to each data.
The following is a simple code implementation using the LFU algorithm in Java:
public class LFUCache<K, V> extends LinkedHashMap<K, V> { private final int CACHE_SIZE; private Map<K, Integer> countMap; public LFUCache(int cacheSize) { super((int)Math.ceil(cacheSize / 0.75f) + 1, 0.75f, true); CACHE_SIZE = cacheSize; countMap = new HashMap<>(); } @Override public V put(K key, V value) { V oldValue = super.put(key, value); if (size() > CACHE_SIZE) { K leastUsedKey = getLeastUsedKey(); super.remove(leastUsedKey); countMap.remove(leastUsedKey); } countMap.put(key, countMap.getOrDefault(key, 0) + 1); return oldValue; } private K getLeastUsedKey() { K leastUsedKey = null; int leastUsedCount = Integer.MAX_VALUE; for (Map.Entry<K, Integer> entry : countMap.entrySet()) { if (entry.getValue() < leastUsedCount) { leastUsedCount = entry.getValue(); leastUsedKey = entry.getKey(); } } return leastUsedKey; } }
3. FIFO algorithm
The FIFO algorithm is first in, first out. This algorithm means that the data placed first in the cache is deleted first. When the cache space is insufficient, the data that is first put into the cache should be deleted and the newly arrived data should be placed at the end. The core of the FIFO algorithm is to maintain a queue, which records the insertion time of each data.
The following is a simple code implementation using the FIFO algorithm in Java:
public class FIFOCache<K, V> extends LinkedHashMap<K, V> { private final int CACHE_SIZE; public FIFOCache(int cacheSize) { super((int)Math.ceil(cacheSize / 0.75f) + 1, 0.75f, true); CACHE_SIZE = cacheSize; } @Override protected boolean removeEldestEntry(Map.Entry eldest) { return size() > CACHE_SIZE; } }
The above three caching algorithms have their own advantages and disadvantages. The disadvantage of the LRU algorithm is that if a piece of data is accessed only once in a long period of time, it will also be cached. The disadvantage of the LFU algorithm is that it requires maintaining a counter table, which adds additional overhead. The disadvantage of the FIFO algorithm is that the data in the cache is not necessarily the most commonly used.
In practical applications, the appropriate algorithm should be selected according to the specific scenario. For example, for some frequently accessed data, you can use the LRU algorithm; for less frequently accessed data, you can use the LFU algorithm; for application scenarios where cache efficiency is more important, you can use the FIFO algorithm.
The above is the detailed content of Caching algorithms: Detailed explanation of LRU, LFU, and FIFO algorithms in Java caching technology. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
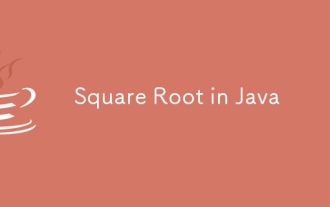
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
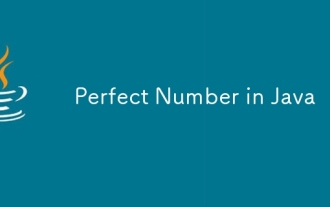
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
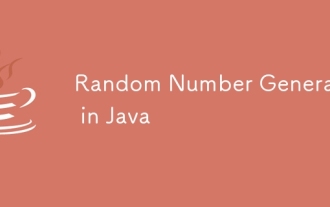
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
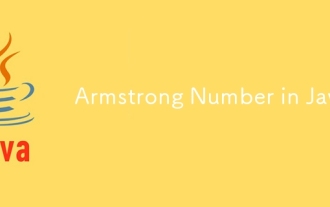
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
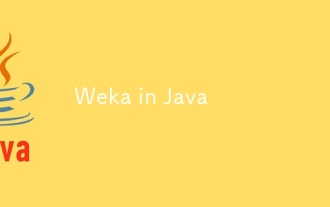
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
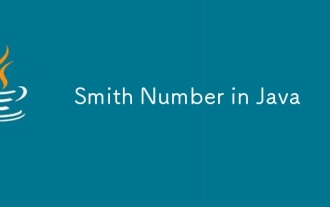
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
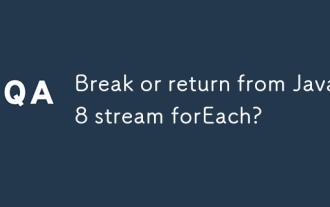
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
