How to use ThinkPHP6 to achieve no login required
ThinkPHP6 is a popular PHP framework that provides powerful tools and features to help developers quickly build web applications. Among them, implementing the login-free function is very important in many applications.
This article will introduce how to use ThinkPHP6 to implement the login-free function, and explore some best practices and techniques.
1. Understand the basic principles of login-free
In the process of login-free, we need to understand some basic principles.
First of all, login-free is usually implemented based on cookie or session mechanism. When a user logs into an application for the first time, the server assigns them a unique identifier (such as a session ID or token). This identifier can then be sent back to the server on subsequent requests by the application to prove that the user has been authenticated. This eliminates the need to enter your username and password again to access protected pages of the application.
Secondly, we need to understand how to store the identifier in a cookie or session. In ThinkPHP6, you can use the session function to easily achieve this operation.
2. Use ThinkPHP6 to realize login-free function
Below, we will introduce step by step how to use ThinkPHP6 to realize login-free function.
- Create login interface and logic
First, you need to create a login interface and logic. We can do this using a simple HTML form. When processing a login request, the username and password need to be verified, and a session ID or token is created for the user upon successful login. If login fails, corresponding error information needs to be returned.
This can be done using the following code:
public function login() { $data = $this->request->param(); $user = UserModel::where('username', $data['username'])->find(); if (!$user || $user['password'] != md5($data['password'])) { return ['code' => -1, 'msg' => '用户名或密码错误']; } // 登录成功,在session中保存用户信息 session('user', $user); return ['code' => 0, 'msg' => '登录成功']; }
In the above code, we first retrieve whether the username and password entered by the user are valid. If valid, a session ID or token is created for the user and saved on the server. If login fails, an error message is returned.
- Creating protected pages
Next, we need to create one or more protected pages. These pages can only be accessed if the user is logged in and has a valid session ID or token. Otherwise, the user will be redirected to the login page. This can be achieved using the following code:
public function index() { // 检查用户是否已登录 $user = session('user'); if (!$user) { return redirect('user/login'); } return $this->fetch(); }
In the above code, we first check if the user is logged in. If the user is logged in, relevant content is displayed. If the user is not logged in, redirect them to the login page.
- Realize login-free
Now, we can implement basic login and access control of protected pages. However, on this basis, we need to implement the login-free function.
The way to achieve login-free is very simple: store the user's session ID or token in a cookie. Then, when the user visits the application again, this cookie value can be sent back to the server to prove that the user has been authenticated.
This can be achieved using the following code:
public function login() { // 检查cookie中是否存在session ID或token $user = session('user'); if ($user) { return redirect('user/index'); } $data = $this->request->param(); $user = UserModel::where('username', $data['username'])->find(); if (!$user || $user['password'] != md5($data['password'])) { return ['code' => -1, 'msg' => '用户名或密码错误']; } // 登录成功,在session和cookie中保存用户信息 session('user', $user); cookie('user_id', $user['id'], 3600 * 24 * 7); return ['code' => 0, 'msg' => '登录成功']; }
In the above code, we first check whether a valid session ID or token already exists. If present, redirect the user to the protected page. Otherwise, we verify the username and password and on successful login create a session ID or token for the user and save it on the server. Additionally, we store the user ID in a cookie for verification on future requests.
We can then use the following code in the protected page to check the user ID stored in the cookie and retrieve the corresponding user information accordingly:
public function index() { // 检查cookie中是否存在用户ID $user_id = cookie('user_id'); if (!$user_id) { return redirect('user/login'); } // 检索用户信息 $user = UserModel::get($user_id); if (!$user) { return redirect('user/login'); } return $this->fetch(); }
In the above code, we First check if a valid user ID cookie exists. If present, the user information is retrieved using that ID. If the user is not found, redirect the user to the login page.
3. Best practices and techniques
When using ThinkPHP6 to achieve login-free, the following are some best practices and techniques:
- Use secure hash function Store password. In the sample code, we use the md5 function for password hashing. However, this is not secure as md5 can be easily brute-forced. It is recommended to use more secure hash functions such as bcrypt or scrypt.
- Set an unguessable key for the cookie. The secret can be set using secret_key in the config/app.php file.
- The shorter the cookie expiration time is set, the safer it is. Otherwise, if the cookie is stolen, an attacker can use it for authentication for a long time.
- You can consider using the remember me function. This feature will keep the user's cookie valid for a long time, but will only expire when the user actively logs out.
In general, it is very simple to use ThinkPHP6 to achieve login-free. Follow best practices and tips to ensure your application remains secure while protecting users.
The above is the detailed content of How to use ThinkPHP6 to achieve no login required. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
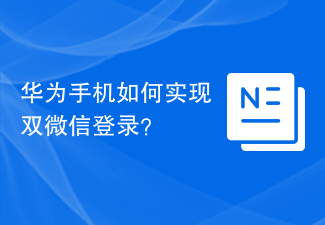
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
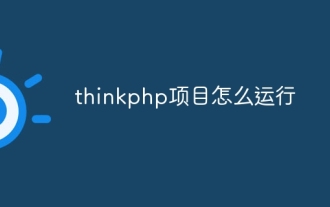
To run the ThinkPHP project, you need to: install Composer; use Composer to create the project; enter the project directory and execute php bin/console serve; visit http://localhost:8000 to view the welcome page.
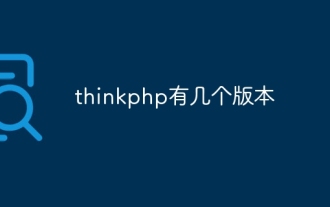
ThinkPHP has multiple versions designed for different PHP versions. Major versions include 3.2, 5.0, 5.1, and 6.0, while minor versions are used to fix bugs and provide new features. The latest stable version is ThinkPHP 6.0.16. When choosing a version, consider the PHP version, feature requirements, and community support. It is recommended to use the latest stable version for best performance and support.
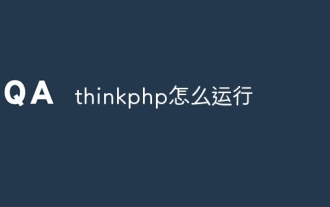
Steps to run ThinkPHP Framework locally: Download and unzip ThinkPHP Framework to a local directory. Create a virtual host (optional) pointing to the ThinkPHP root directory. Configure database connection parameters. Start the web server. Initialize the ThinkPHP application. Access the ThinkPHP application URL and run it.
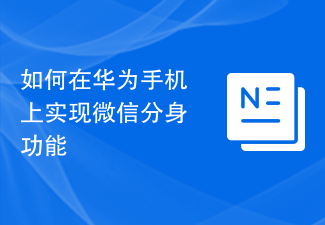
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
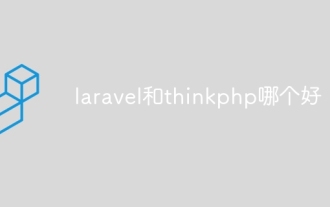
Performance comparison of Laravel and ThinkPHP frameworks: ThinkPHP generally performs better than Laravel, focusing on optimization and caching. Laravel performs well, but for complex applications, ThinkPHP may be a better fit.
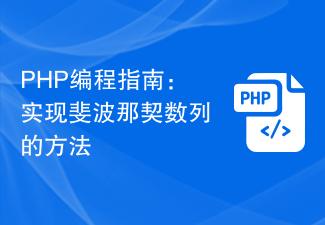
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
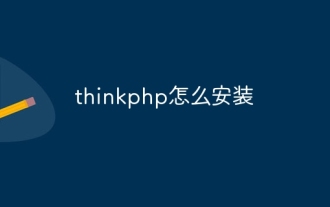
ThinkPHP installation steps: Prepare PHP, Composer, and MySQL environments. Create projects using Composer. Install the ThinkPHP framework and dependencies. Configure database connection. Generate application code. Launch the application and visit http://localhost:8000.
