Forms in the Yii framework: building user interaction interfaces
Yii framework is a popular PHP framework that is widely used for web application development. The framework provides a wealth of features and tools, one of the most important features is forms. In this article, we will explore forms in the Yii framework, including how to build user interaction interfaces.
What is a form?
In web applications, a form is an interface element that allows users to enter or submit data. Typically, a form contains a series of input fields, such as text boxes, drop-down lists, radio buttons, and check boxes. When a user fills out a form and submits it, this data is typically sent to the server for processing.
Forms in Yii framework
Yii framework provides multiple ways to build forms. Among them, the most commonly used is to use Active Form provided by Yii. Active Form is a core class of Yii that can generate forms quickly and efficiently. When using Active Form, we can generate forms through Yii's Model class and Active Record model class.
Use Yii's Model class to generate a form
When using Yii's Model class to generate a form, we need to first create a new Model class, and then define the properties corresponding to each field of the form. For example:
class ContactForm extends yiiaseModel { public $name; public $email; public $subject; public $body; public function rules() { return [ [['name', 'email', 'subject', 'body'], 'required'], ['email', 'email'], ]; } }
In this example, we define four attributes: name, email address, subject, and body, and use the rules() method to define the rules. These rules can be used to validate user input data.
Next, we can use Active Form to generate a new form:
<?php $form = ActiveForm::begin(); ?> <?= $form->field($model, 'name') ?> <?= $form->field($model, 'email') ?> <?= $form->field($model, 'subject') ?> <?= $form->field($model, 'body')->textarea(['rows' => 6]) ?> <div class="form-group"> <?= Html::submitButton('Submit', ['class' => 'btn btn-primary']) ?> </div> <?php ActiveForm::end(); ?>
In this example, we use the begin() and end() methods of Active Form, and then use $ The field method generates input fields for each attribute. Finally, we added a submit button.
Use Active Record model classes to generate forms
Using Active Record model classes can also quickly build forms. Active Record is a class used by the Yii framework to operate the database. It can define a model attribute for each field in the table.
For example, we have a Users table, which contains id, username and email fields. We can use Yii's Active Record to generate a new model class:
class UserForm extends yiidbActiveRecord { public static function tableName() { return 'Users'; } public function rules() { return [ [['username', 'email'], 'required'], ['email', 'email'], ]; } }
In this example, we define a tableName() method to indicate which database table to use. We can then do any usual Active Record operations on this class.
To build a form using Active Form, we can use the following code:
<?php $form = ActiveForm::begin(); ?> <?= $form->field($model, 'username') ?> <?= $form->field($model, 'email') ?> <div class="form-group"> <?= Html::submitButton('Submit', ['class' => 'btn btn-primary']) ?> </div> <?php ActiveForm::end(); ?>
In this example, we use the begin() and end() methods of Active Form, and then use the $field method Generate input fields for each attribute. We also added a submit button.
Conclusion
The form in the Yii framework is an important tool for building user interaction interfaces. Whether you use Yii's Model class or Active Record model class, you can quickly and effectively generate rich forms. In addition to the input fields in the above examples, the Yii framework also provides various other input field options, such as file upload, date selection, password input boxes, etc. If you want to know more about the Yii framework and forms, you can check out the Yii documentation or official website.
The above is the detailed content of Forms in the Yii framework: building user interaction interfaces. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


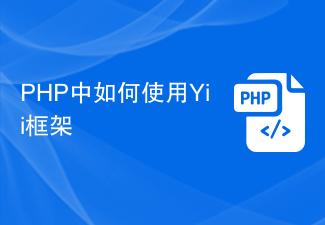
With the rapid development of web applications, modern web development has become an important skill. Many frameworks and tools are available for developing efficient web applications, among which the Yii framework is a very popular framework. Yii is a high-performance, component-based PHP framework that uses the latest design patterns and technologies, provides powerful tools and components, and is ideal for building complex web applications. In this article, we will discuss how to use Yii framework to build web applications. Install Yii framework first,
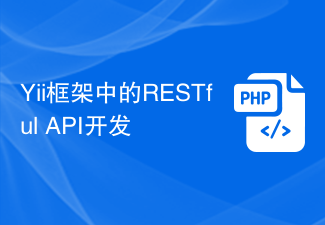
Yii is a high-performance MVC framework based on PHP. It provides a very rich set of tools and functions to support the rapid and efficient development of web applications. Among them, the RESTful API function of the Yii framework has attracted more and more attention and love from developers, because using the Yii framework can easily build high-performance and easily scalable RESTful interfaces, providing strong support for the development of web applications. . Introduction to RESTfulAPI RESTfulAPI is a
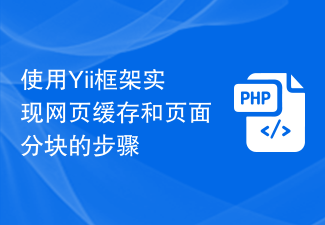
Steps to implement web page caching and page chunking using the Yii framework Introduction: During the web development process, in order to improve the performance and user experience of the website, it is often necessary to cache and chunk the page. The Yii framework provides powerful caching and layout functions, which can help developers quickly implement web page caching and page chunking. This article will introduce how to use the Yii framework to implement web page caching and page chunking. 1. Turn on web page caching. In the Yii framework, web page caching can be turned on through the configuration file. Open the main configuration file co
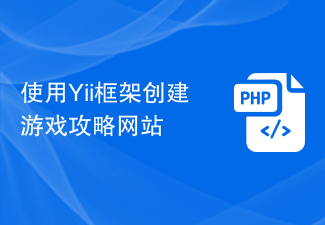
In recent years, with the rapid development of the game industry, more and more players have begun to look for game strategies to help them pass the game. Therefore, creating a game guide website can make it easier for players to obtain game guides, and at the same time, it can also provide players with a better gaming experience. When creating such a website, we can use the Yii framework for development. The Yii framework is a web application development framework based on the PHP programming language. It has the characteristics of high efficiency, security, and strong scalability, and can help us create a game guide more quickly and efficiently.
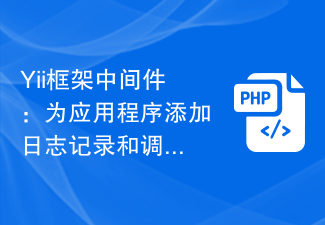
Yii framework middleware: Add logging and debugging capabilities to applications [Introduction] When developing web applications, we usually need to add some additional features to improve the performance and stability of the application. The Yii framework provides the concept of middleware that enables us to perform some additional tasks before and after the application handles the request. This article will introduce how to use the middleware function of the Yii framework to implement logging and debugging functions. [What is middleware] Middleware refers to the processing of requests and responses before and after the application processes the request.
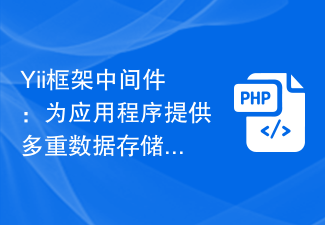
Yii framework middleware: providing multiple data storage support for applications Introduction Middleware (middleware) is an important concept in the Yii framework, which provides multiple data storage support for applications. Middleware acts like a filter, inserting custom code between an application's requests and responses. Through middleware, we can process, verify, filter requests, and then pass the processed results to the next middleware or final handler. Middleware in the Yii framework is very easy to use
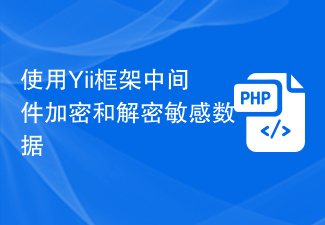
Encrypting and decrypting sensitive data using Yii framework middleware Introduction: In modern Internet applications, privacy and data security are very important issues. To ensure that users' sensitive data is not accessible to unauthorized visitors, we need to encrypt this data. The Yii framework provides us with a simple and effective way to implement the functions of encrypting and decrypting sensitive data. In this article, we’ll cover how to achieve this using the Yii framework’s middleware. Introduction to Yii framework Yii framework is a high-performance PHP framework.
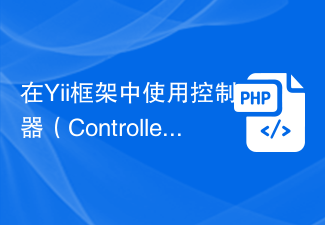
In the Yii framework, controllers play an important role in processing requests. In addition to handling regular page requests, controllers can also be used to handle Ajax requests. This article will introduce how to handle Ajax requests in the Yii framework and provide code examples. In the Yii framework, processing Ajax requests can be carried out through the following steps: The first step is to create a controller (Controller) class. You can inherit the basic controller class yiiwebCo provided by the Yii framework
