PHP application function application: find strings in files
With the rapid development of the Internet, Web application development has become more and more common. As a widely used server-side programming language, PHP plays a vital role in web application development. The PHP language is very flexible and is widely used due to its ease of learning and deployment.
In the PHP language, functions are a very important concept. Functions allow us to encapsulate code, improve code reusability, simplify code structure, and improve code readability. In this article, we will discuss an application based on PHP function: Find a string in a file.
In web application development, we often need to find text or strings in files. In PHP, we can use its built-in functions to perform this operation. First, we need to open the file.
$file = fopen('example.txt', 'r');
After opening the file, we can read each line of the file and look for specific strings during the reading process. We can use the PHP built-in function fgets()
to get each line in the file. fgets()
The function reads one line from the file at a time and returns it.
while (!feof($file)) { $line = fgets($file); // 在每行中查找字符串 }
Next, we need to use the strpos()
function to find the string. strpos()
The function returns the position of the first occurrence of a given string in another string. If the string is not found, false
is returned. Using strpos()
function we can easily find the specified string in each line.
$search_str = 'example'; while (!feof($file)) { $line = fgets($file); if (strpos($line, $search_str) !== false) { // 找到了字符串,这里可以处理相应的逻辑。 } }
Finally, we need to close the open file after the program is executed:
fclose($file);
The above code demonstrates the basic process of finding strings in files through PHP functions. Using functions to encapsulate these operations can make the entire process more concise and improve the readability of the code.
function find_string_in_file($filename, $search_str) { $file = fopen($filename, 'r'); $result = ''; while (!feof($file)) { $line = fgets($file); if (strpos($line, $search_str) !== false) { $result .= $line; } } fclose($file); return $result; }
At this point, we only need to call this function to find a specific string in the file:
$result = find_string_in_file('example.txt', 'example');
In short, functions in PHP are very powerful and flexible tools. In this article, we demonstrated how to use a function to find a specified string in a file. Through this example, we can see that functions can not only improve the reusability and readability of the code, but also greatly simplify the structure of the code. Therefore, rational use of functions is very important in web application development.
The above is the detailed content of PHP application function application: find strings in files. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


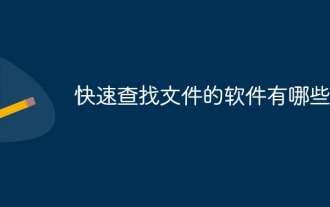
Software for quickly finding files: 1. Xiaozhi Soso; 2. Everything; 3. Listary; 4. AnyTXT Searcher.
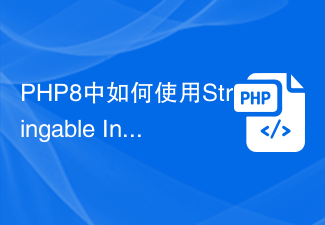
How to use StringableInterface to handle string operations more conveniently in PHP8? PHP8 is the latest version of the PHP language and brings many new features and improvements. One of the improvements that developers are excited about is the addition of StringableInterface. StringableInterface is an interface for handling string operations, which provides a more convenient way to handle and operate strings. This article will detail how to use
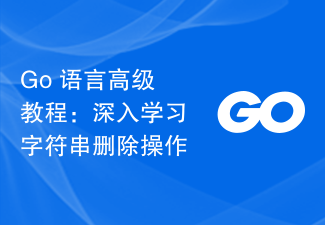
Go language is a very popular programming language, and its powerful features make it favored by many developers. String operations are one of the most common operations in programming, and in the Go language, string deletion operations are also very common. This article will delve into the string deletion operation in the Go language and use specific code examples to help you better understand and master this knowledge point. String deletion operation In the Go language, we usually use the strings package to perform string operations, including deletion operations.
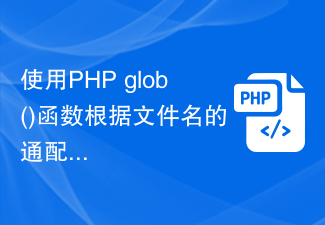
When processing files, we often encounter situations where we need to find a specified file. If the number of files is small, manual search can be used. However, if a large number of files are involved, manual search becomes overwhelming. At this time, a useful function comes in handy - the glob() function. The glob() function is a very useful function in PHP, which allows us to find files and directories by specifying wildcard characters. The glob() function can also sort and sort the found files through various parameters.
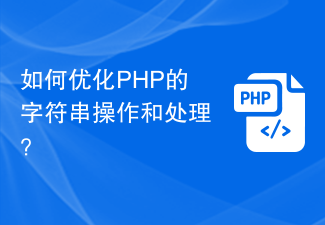
How to optimize PHP's string manipulation and processing? In web development, string manipulation and processing are very common and important parts. For PHP, string optimization can improve program performance and response speed. This article will introduce some methods to optimize PHP string manipulation and processing. Avoid unnecessary string concatenation operations String concatenation operations (using the "." operator) can lead to poor performance, especially within loops. For example, the following code: $str="";for($
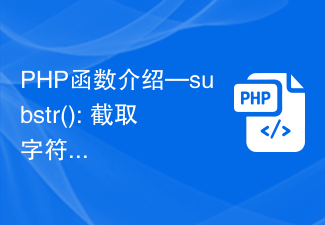
PHP function introduction—substr(): intercepting part of a string In PHP, string processing is a very common operation. For a string, sometimes we need to intercept part of the content for processing. At this time, PHP provides a very practical function-substr(). The substr() function can intercept a part of a string. The specific usage is as follows: stringsubstr(string$string,int
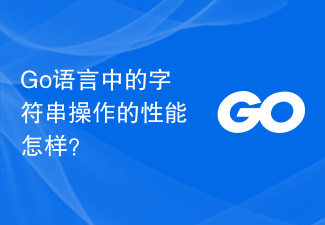
What is the performance of string operations in Go language? In program development, string processing is inevitable, especially in Web development, string processing occurs frequently. Therefore, the performance of string operations is obviously a matter of great concern to developers. So, what is the performance of string operations in Go language? This article will discuss the performance of string operations in Go language from the following aspects. Basic operations Strings in Go language are immutable, that is, once created, the characters in them cannot be modified.
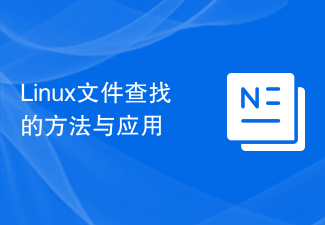
Finding files in Linux is one of the techniques we often use in daily operation and maintenance work. By searching for files, we can quickly locate specific files and perform corresponding operations. This article will introduce the techniques and practices commonly used to find files under Linux, with specific code examples. I hope it will be helpful to everyone. 1. Use the find command. The find command is a very powerful file search tool in Linux systems. It can recursively search for files in a specified path based on specified conditions. Here are some common find commands
