Create an online store using the Yii framework
As a web developer, learning and practicing using the PHP framework is undoubtedly essential. Among many PHP frameworks, the Yii framework is an efficient, elegant, and secure framework with a wide user base.
In this article, I will share how to use the Yii framework to create a basic online mall application. The application will have basic mall functions such as user management, product management and shopping cart functions. This application can be used as an introductory practice for beginners to learn the Yii framework.
Install the Yii framework
Before we start using the Yii framework, we need to install the framework first. The Yii framework provides multiple installation methods, the most commonly used method is to install using Composer. Before installation, we need to confirm that Composer is installed.
We can use the following command to install the Yii framework:
composer create-project --prefer-dist yiisoft/yii2-app-basic basic
The above command will create a basic application. You can confirm whether the Yii framework is installed successfully by visiting http://localhost/basic.
Data table design and data filling
Before creating an online mall application, we need to create a data table related to the mall and fill in the data. This article will use MySQL as the database and create the following data table:
- User table: stores user information, such as user name, password, and email.
- Product table: Stores product information, such as name, price and description.
- Order table: stores order information, such as user ID, product ID and order quantity.
- Cart table: Stores shopping cart information, such as user ID, product ID and quantity, etc.
Adding appropriate indexes to the above data tables can improve query efficiency.
The following is the SQL statement to create the above data table:
CREATE TABLE `user` ( `id` int(11) NOT NULL AUTO_INCREMENT, `username` varchar(255) NOT NULL, `password` varchar(255) NOT NULL, `email` varchar(255) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8; CREATE TABLE `product` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(255) NOT NULL, `price` float(10,2) NOT NULL, `description` varchar(255) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8; CREATE TABLE `order` ( `id` int(11) NOT NULL AUTO_INCREMENT, `user_id` int(11) NOT NULL, `product_id` int(11) NOT NULL, `quantity` int(11) NOT NULL, PRIMARY KEY (`id`), KEY `user_id` (`user_id`), KEY `product_id` (`product_id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8; CREATE TABLE `cart` ( `id` int(11) NOT NULL AUTO_INCREMENT, `user_id` int(11) NOT NULL, `product_id` int(11) NOT NULL, `quantity` int(11) NOT NULL, PRIMARY KEY (`id`), KEY `user_id` (`user_id`), KEY `product_id` (`product_id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Now, we need to fill in the data to test the application. Add at least one user record to the user table and at least one product record to the product table. We will use this user and product as test data for the remainder of this article.
Creating a User Controller
We will start by creating a User Controller. In the Yii framework, controllers are the core components that handle application requests. The controller is responsible for detecting the request type and calling the response operation method based on the request.
Now, we create the UserController controller and add basic action methods. We will use the yiiwebController extension to create the controller.
In Yii, the controller class name is usually added with the Controller suffix, which can better describe the functions implemented by the class. The following is the basic code of the UserController class:
<?php namespace appcontrollers; use yiiwebController; class UserController extends Controller { public function actionIndex() { return $this->render('index'); } public function actionLogin() { // code for login logic } public function actionRegister() { // code for registration logic } }
In the above code, we have added three basic operation methods. The actionIndex() method will render the index.php view file. This is the default action method name. The actionLogin() and actionRegister() methods will be used for user login and registration.
Now, we need to create the index.php view file. View files are the output of action methods in the controller. We will use the Yii::$app->view->render() method provided by the Yii framework to render the view.
Create the index.php file in the views/user folder and add the following code:
<?php use yiihelpersHtml; $this->title = 'User'; $this->params['breadcrumbs'][] = $this->title; ?> <h1><?= Html::encode($this->title) ?></h1> <p> Welcome to the user homepage. </p>
The above code will display a simple welcome message on the browser.
Creating the product controller and view
Now, we will move on to creating the product controller and view. We will use yiidataActiveDataProvider extension to get the product list data.
Create a ProductController controller and add the following action method:
<?php namespace appcontrollers; use yiiwebController; use appmodelsProduct; use yiidataActiveDataProvider; class ProductController extends Controller { public function actionIndex() { $dataProvider = new ActiveDataProvider([ 'query' => Product::find(), ]); return $this->render('index', [ 'dataProvider' => $dataProvider, ]); } public function actionAddtocart($id) { // code for add to cart logic } }
As shown in the code, we first use Product::find() to get all products. We then put the product list data in an ActiveDataProvider and pass it to the view. After this, we create the actionAddtocart() method for adding items to the shopping cart.
We need to create the product folder and create the index.php view file under this folder. We use a GridView widget that will render a list of products based on the data provided by the dataProvider. We also add a button for adding products to the cart.
The following is the code of the views/product/index.php file:
<?php use yiihelpersHtml; use yiigridGridView; $this->title = 'Product'; $this->params['breadcrumbs'][] = $this->title; ?> <h1><?= Html::encode($this->title) ?></h1> <?= GridView::widget([ 'dataProvider' => $dataProvider, 'columns' => [ 'id', 'name', 'price', 'description', [ 'class' => 'yiigridActionColumn', 'buttons' => [ 'addtocart' => function ($url, $model) { return Html::a('Add to cart', ['product/addtocart', 'id' => $model->id]); } ], 'template' => '{addtocart}', ], ], ]); ?>
Now, we can confirm the view by visiting http://localhost/basic/product/index in the browser is rendered correctly.
Create a shopping cart controller and view
We need a shopping cart controller and view to manage the items added to the shopping cart in the mall. We will use session to store shopping cart data.
We create the CartController controller and add the following operation method:
<?php namespace appcontrollers; use yiiwebController; use Yii; class CartController extends Controller { public function actionIndex() { $cart = Yii::$app->session->get('cart'); return $this->render('index', [ 'cart' => $cart, ]); } public function actionAdd($id) { // code for add product to cart logic } public function actionRemove($id) { // code for remove product from cart logic } }
In the above code, we first obtain the shopping cart data stored in the session. In the actionAdd($id) method, we will add the product with the specified ID number to the shopping cart. In the actionRemove($id) method, we will remove the item with the specified ID number from the shopping cart.
Next, we need to create the cart folder and create the index.php view file under this folder. We use the ListView widget which will render the shopping cart list based on the shopping cart data stored in the session. We also added some buttons for increasing or decreasing the number of items in the cart or removing items from the cart.
The following is the code of views/cart/index.php file:
<?php use yiihelpersHtml; use yiiwidgetsListView; $this->title = 'Shopping Cart'; $this->params['breadcrumbs'][] = $this->title; ?> <h1><?= Html::encode($this->title) ?></h1> <?= ListView::widget([ 'dataProvider' => $dataProvider, 'itemView' => '_cart_item', 'emptyText' => 'Your cart is empty.', ]) ?>
在views/cart文件夹下创建_cart_item.php视图文件,该文件将被用于渲染购物车列表中的每一行。以下是views/cart/_cart_item.php文件的代码:
<?php use yiihelpersHtml; use yiiwidgetsActiveForm; $form = ActiveForm::begin([ 'id' => 'cart-item-' . $model['id'], ]); ?> <?= Html::a('Remove', ['cart/remove', 'id' => $model['id']]) ?> <?= $model['name'] ?> <?= $form->field($model, 'quantity')->textInput(['value' => $model['quantity']]) ?> <?= Html::submitButton('Update') ?> <?php ActiveForm::end(); ?>
以上代码将会在浏览器中显示购物车列表,用户可以在该列表中执行增加或减少商品数量的操作,也可以删除购物车中已有商品。
完成在线商城应用程序
现在,我们已经完成了基础的在线商城应用程序,该应用程序拥有用户管理,产品管理和购物车等基础功能。该应用程序可以作为初学者学习和实践Yii框架的入门实践。当然,在实际应用中,我们仍需要添加更多功能来满足商城的需求。
The above is the detailed content of Create an online store using the Yii framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
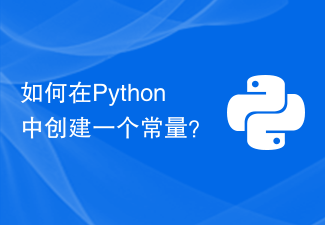
Constants and variables are used to store data values in programming. A variable usually refers to a value that can change over time. A constant is a type of variable whose value cannot be changed during program execution. There are only six built-in constants available in Python, they are False, True, None, NotImplemented, Ellipsis(...) and __debug__. Apart from these constants, Python does not have any built-in data types to store constant values. Example An example of a constant is demonstrated below - False=100 outputs SyntaxError:cannotassigntoFalseFalse is a built-in constant in Python that is used to store boolean values
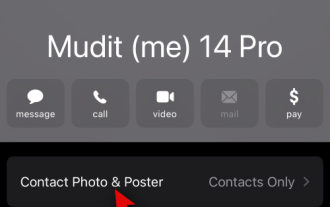
How to Personalize Calls on iPhone Apple’s iOS 17 introduces a new feature called Contact Posters that allows you to personalize the look of your call screen on your iPhone. This feature allows you to design a poster using selected photos, colors, fonts, and Memoji as contact cards. So when you make a call, your custom image will appear on the recipient's iPhone exactly as you envisioned. You can choose to share your unique contact poster with all your saved contacts, or choose who can see it. Likewise, during a call exchange, you will also see other people's contact posters. Additionally, Apple lets you set specific contact photos for individual contacts, making calls from those contacts
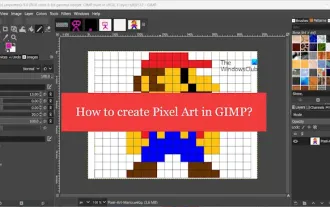
This article will interest you if you are interested in using GIMP for pixel art creation on Windows. GIMP is a well-known graphics editing software that is not only free and open source, but also helps users create beautiful images and designs easily. In addition to being suitable for beginners and professional designers alike, GIMP can also be used to create pixel art, a form of digital art that utilizes pixels as the only building blocks for drawing and creating. How to Create Pixel Art in GIMP Here are the main steps to create pixel pictures using GIMP on a Windows PC: Download and install GIMP, then launch the application. Create a new image. Resize width and height. Select the pencil tool. Set the brush type to pixels. set up
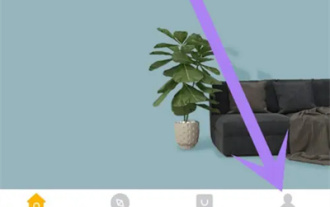
Many friends expressed that they want to know how to create a family in Gree+ software. Here is the operation method for you. Friends who want to know more, come and take a look with me. First, open the Gree+ software on your mobile phone and log in. Then, in the options bar at the bottom of the page, click the "My" option on the far right to enter the personal account page. 2. After coming to my page, there is a "Create Family" option under "Family". After finding it, click on it to enter. 3. Next jump to the page to create a family, enter the family name to be set in the input box according to the prompts, and click the "Save" button in the upper right corner after entering it. 4. Finally, a "save successfully" prompt will pop up at the bottom of the page, indicating that the family has been successfully created.
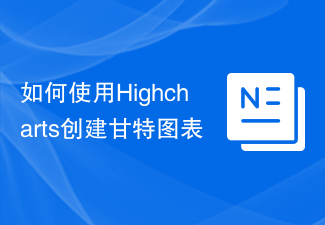
How to use Highcharts to create a Gantt chart requires specific code examples. Introduction: The Gantt chart is a chart form commonly used to display project progress and time management. It can visually display the start time, end time and progress of the task. Highcharts is a powerful JavaScript chart library that provides rich chart types and flexible configuration options. This article will introduce how to use Highcharts to create a Gantt chart and give specific code examples. 1. Highchart
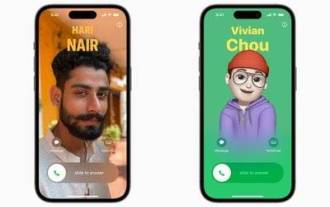
In iOS17, Apple has added a contact poster feature to its commonly used Phone and Contacts apps. This feature allows users to set personalized posters for each contact, making the address book more visual and personal. Contact posters can help users identify and locate specific contacts more quickly, improving user experience. Through this feature, users can add specific pictures or logos to each contact according to their preferences and needs, making the address book interface more vivid. Apple in iOS17 provides iPhone users with a novel way to express themselves, and added a personalizable contact poster. The Contact Poster feature allows you to display unique, personalized content when calling other iPhone users. you
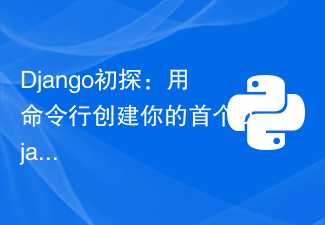
Start the journey of Django project: start from the command line and create your first Django project. Django is a powerful and flexible web application framework. It is based on Python and provides many tools and functions needed to develop web applications. This article will lead you to create your first Django project starting from the command line. Before starting, make sure you have Python and Django installed. Step 1: Create the project directory First, open the command line window and create a new directory
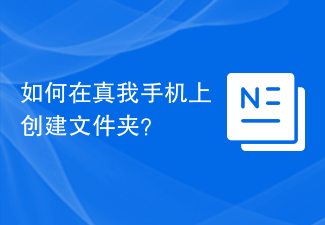
Title: Realme Phone Beginner’s Guide: How to Create Folders on Realme Phone? In today's society, mobile phones have become an indispensable tool in people's lives. As a popular smartphone brand, Realme Phone is loved by users for its simple and practical operating system. In the process of using Realme phones, many people may encounter situations where they need to organize files and applications on their phones, and creating folders is an effective way. This article will introduce how to create folders on Realme phones to help users better manage their phone content. No.
