Reflection mechanism and its applications in PHP
With the development of software engineering, the complexity of code gradually increases, and we need higher-level tools to help us understand and manage code. In PHP, the reflection mechanism is a powerful tool that allows us to query and manipulate various aspects of the code at runtime. In this article, we will learn the basics of PHP reflection mechanism and its uses in practical applications.
1. Basic knowledge of reflection mechanism
1.1 What is reflection mechanism?
The reflection mechanism is the ability to obtain and manipulate program elements at runtime. In PHP, this means that we can query and adjust information about classes, functions, methods, and properties at runtime without needing to access them directly.
1.2 Reflection class
In PHP, we can use reflection classes to implement the reflection mechanism. Reflection classes contain a large number of methods and properties that allow us to query and manipulate various aspects of PHP code. In PHP, there are three main reflection classes:
- ReflectionClass: used to query class information;
- ReflectionMethod: used to query method information in a class;
- ReflectionProperty: used to query property information in a class.
1.3 Create a reflection object
To use the reflection class, we need to create a reflection object first. The method of creating a reflection object is as follows:
$reflectionObj = new ReflectionClass('类名/函数名/方法名');
Here we can pass a class name, function name or method name to create a reflection object, and then we can use the methods in the reflection object to query and operate these program elements. .
2. Application of reflection mechanism
2.1 Class reflection
Class reflection is one of the most commonly used applications of the reflection mechanism. With ReflectionClass, we can query all information of a class, including constants, properties, methods, etc. Here is a simple example:
class MyClass { const MY_CONST = 1; private $myProperty; public function __construct() { $this->myProperty = 2; } public function myMethod($arg1, $arg2) { echo "arg1: ".$arg1." arg2: ".$arg2." "; } } $reflectionObj = new ReflectionClass('MyClass'); // 查询类的名称、命名空间、父类等信息 echo "类名:".$reflectionObj->getName()." "; echo "命名空间:".$reflectionObj->getNamespaceName()." "; echo "父类:".$reflectionObj->getParentClass()." "; // 查询类的常量 echo "常量MY_CONST:".$reflectionObj->getConstant('MY_CONST')." "; // 查询类的属性 $properties = $reflectionObj->getProperties(); foreach ($properties as $property) { echo $property->getName()." "; } // 查询类的方法 $methods = $reflectionObj->getMethods(); foreach ($methods as $method) { echo $method->getName()." "; }
Using ReflectionClass, we can easily query various aspects of a class. For example, we can dynamically create instances of a class at runtime, or understand the structure of a class while analyzing and debugging code.
2.2 Method reflection
Method reflection is another important application of the reflection mechanism. The ReflectionMethod class allows us to query methods in a class and analyze information such as its parameters, return type, and access modifiers. Here is a simple example:
class MyClass { private function myMethod($arg1, $arg2) { echo "arg1: ".$arg1." arg2: ".$arg2." "; } } $reflectionObj = new ReflectionClass('MyClass'); $method = $reflectionObj->getMethod('myMethod'); // 查询方法名称、参数数目、可变参数等信息 echo "方法名称:".$method->getName()." "; echo "参数数目:".$method->getNumberOfParameters()." "; echo "可变参数?:".($method->isVariadic() ? '是' : '否')." "; // 查询参数类型、默认值等信息 $params = $method->getParameters(); foreach ($params as $param) { echo "参数名称:".$param->getName()." "; echo "参数类型:".$param->getType()->getName()." "; echo "默认值:".($param->isDefaultValueAvailable() ? $param->getDefaultValue() : '无')." "; } // 查询方法的访问修饰符 echo "访问修饰符:".$method->getModifiers()." "; echo "public?:".($method->isPublic() ? '是' : '否')." "; echo "protected?:".($method->isProtected() ? '是' : '否')." "; echo "private?:".($method->isPrivate() ? '是' : '否')." ";
Method reflection is an effective way to dynamically query and operate methods in a class. For example, we can use ReflectionMethod to modify the access modifier of a method and dynamically call the method at runtime.
2.3 Attribute reflection
Attribute reflection is another important application of the reflection mechanism. The ReflectionProperty class allows us to query and modify properties in a class, including access to modifiers, default values, annotations and other information. Here is a simple example:
class MyClass { private $myProperty = 1; } $reflectionObj = new ReflectionClass('MyClass'); $property = $reflectionObj->getProperty('myProperty'); // 查询属性名称、访问修饰符等信息 echo "属性名称:".$property->getName()." "; echo "访问修饰符:".$property->getModifiers()." "; echo "public?:".($property->isPublic() ? '是' : '否')." "; echo "protected?:".($property->isProtected() ? '是' : '否')." "; echo "private?:".($property->isPrivate() ? '是' : '否')." "; // 查询属性默认值 echo "默认值:".($property->isInitialized() ? $property->getValue(new MyClass) : '无')." "; // 修改属性的值 $myObj = new MyClass; $property->setValue($myObj, 2); echo "修改后的值:".$myObj->myProperty." ";
Property reflection allows us to dynamically query and modify properties in a class, allowing us to adapt to different runtime environments.
Conclusion
The reflection mechanism is a powerful and flexible tool in PHP, which can help us query and manipulate various aspects of the code at runtime. This article introduces the basic knowledge of the reflection mechanism and the uses of three reflection classes, namely ReflectionClass, ReflectionMethod and ReflectionProperty. The reflection mechanism is widely used, for example, it can play an important role in dynamically creating objects, analyzing and debugging code, and implementing reflection injection.
The above is the detailed content of Reflection mechanism and its applications in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


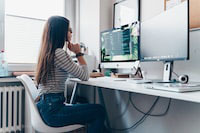
This article will explain in detail how PHP formats rows into CSV and writes file pointers. I think it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Format rows to CSV and write to file pointer Step 1: Open file pointer $file=fopen("path/to/file.csv","w"); Step 2: Convert rows to CSV string using fputcsv( ) function converts rows to CSV strings. The function accepts the following parameters: $file: file pointer $fields: CSV fields as an array $delimiter: field delimiter (optional) $enclosure: field quotes (
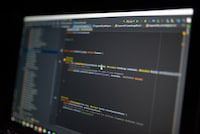
This article will explain in detail about changing the current umask in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Overview of PHP changing current umask umask is a php function used to set the default file permissions for newly created files and directories. It accepts one argument, which is an octal number representing the permission to block. For example, to prevent write permission on newly created files, you would use 002. Methods of changing umask There are two ways to change the current umask in PHP: Using the umask() function: The umask() function directly changes the current umask. Its syntax is: intumas
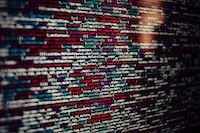
This article will explain in detail how to create a file with a unique file name in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Creating files with unique file names in PHP Introduction Creating files with unique file names in PHP is essential for organizing and managing your file system. Unique file names ensure that existing files are not overwritten and make it easier to find and retrieve specific files. This guide will cover several ways to generate unique filenames in PHP. Method 1: Use the uniqid() function The uniqid() function generates a unique string based on the current time and microseconds. This string can be used as the basis for the file name.
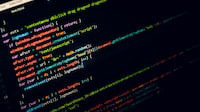
This article will explain in detail about PHP calculating the MD5 hash of files. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP calculates the MD5 hash of a file MD5 (MessageDigest5) is a one-way encryption algorithm that converts messages of arbitrary length into a fixed-length 128-bit hash value. It is widely used to ensure file integrity, verify data authenticity and create digital signatures. Calculating the MD5 hash of a file in PHP PHP provides multiple methods to calculate the MD5 hash of a file: Use the md5_file() function. The md5_file() function directly calculates the MD5 hash value of the file and returns a 32-character
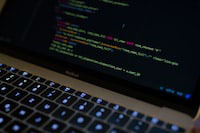
This article will explain in detail how PHP returns an array after key value flipping. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Key Value Flip Array Key value flip is an operation on an array that swaps the keys and values in the array to generate a new array with the original key as the value and the original value as the key. Implementation method In PHP, you can perform key-value flipping of an array through the following methods: array_flip() function: The array_flip() function is specially used for key-value flipping operations. It receives an array as argument and returns a new array with the keys and values swapped. $original_array=[
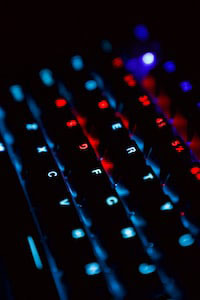
This article will explain in detail how PHP truncates files to a given length. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Introduction to PHP file truncation The file_put_contents() function in PHP can be used to truncate files to a specified length. Truncation means removing part of the end of a file, thereby shortening the file length. Syntax file_put_contents($filename,$data,SEEK_SET,$offset);$filename: the file path to be truncated. $data: Empty string to be written to the file. SEEK_SET: designated as the beginning of the file
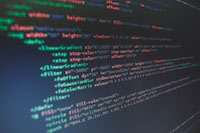
This article will explain in detail how PHP determines whether a specified key exists in an array. The editor thinks it is very practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP determines whether a specified key exists in an array: In PHP, there are many ways to determine whether a specified key exists in an array: 1. Use the isset() function: isset($array["key"]) This function returns a Boolean value, true if the specified key exists, false otherwise. 2. Use array_key_exists() function: array_key_exists("key",$arr

This article will explain in detail the numerical encoding of the error message returned by PHP in the previous Mysql operation. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. . Using PHP to return MySQL error information Numeric Encoding Introduction When processing mysql queries, you may encounter errors. In order to handle these errors effectively, it is crucial to understand the numerical encoding of error messages. This article will guide you to use php to obtain the numerical encoding of Mysql error messages. Method of obtaining the numerical encoding of error information 1. mysqli_errno() The mysqli_errno() function returns the most recent error number of the current MySQL connection. The syntax is as follows: $erro
