


Detailed explanation of middleware of Gin framework and its application
The Gin framework is a lightweight web framework based on the Go language. It has the advantages of high efficiency, flexibility, and easy scalability, and is loved by many developers. The middleware mechanism is a highlight of the Gin framework. In this article, we will explore the middleware mechanism of the Gin framework and its application in detail.
1. What is middleware
Middleware refers to a plug-in that intercepts and rewrites the processing logic of requests and responses during the process of processing network requests. In Go language, middleware is usually implemented using function types. The middleware of the Gin framework is implemented by passing these functions as formal parameters to the functions that handle requests and responses.
In the Gin framework, middleware is divided into two types: global middleware and local middleware. Global middleware acts on all routes, while local middleware acts on a certain route or routing group.
2. The middleware mechanism of the Gin framework
The middleware mechanism of the Gin framework is very simple. You only need to pass the middleware as a function type to the function that handles the request and response.
For example, the following code is a simple middleware:
func MyMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // do something c.Next() // do something after } }
Among them, the MyMiddleware
function defines a middleware function, which returns a function type. The function type returned is the function that handles requests and responses, usually called HandlerFunc
.
HandlerFunc
is defined as follows:
type HandlerFunc func(*Context)
It accepts a parameter of type *Context
, which represents the context of the request. The *Context
type contains various information in the request, such as request headers, request bodies, request parameters, etc.
In the middleware function, we can operate on the context and call the c.Next()
method to transfer control to the next middleware or route processing function.
For example, if we want to add a request header to the middleware, we can do it in the following way:
func AddHeader() gin.HandlerFunc { return func(c *gin.Context) { c.Header("X-Request-Id", "123456") c.Next() } }
This middleware will add a X-Request-Id# to the request. ##Header, and then transfer control to the next handler function. In the routing processing function, we can obtain the value of this request header through the
c.GetHeader method.
Use,
GET,
POST,
PUT,
DELETE and other methods. Can. For example:
import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // 使用全局中间件 r.Use(MyGlobalMiddleware()) // 定义路由组,并使用局部中间件 v1 := r.Group("/v1") { v1.Use(AddHeader()) v1.GET("/hello", Hello) } r.Run(":8080") } func MyGlobalMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // do something c.Next() // do something after } } func AddHeader() gin.HandlerFunc { return func(c *gin.Context) { c.Header("X-Request-Id", "123456") c.Next() } } func Hello(c *gin.Context) { headers := c.Request.Header requestId := headers.Get("X-Request-Id") c.JSON(200, gin.H{ "message": "hello", "request_id": requestId, }) }
MyGlobalMiddleware(), which will act on all routes. We also use a local middleware
AddHeader(), which only acts before the
/v1/hello route. In the
Hello function, we obtain the value of the
X-Request-Id request header and return it to the caller.
- Logger middleware
r := gin.New() r.Use(gin.Logger())
- Recovery middleware
r := gin.Default() r.Use(gin.Recovery())
- Cors middleware
r := gin.Default() r.Use(cors.Default())
The above is the detailed content of Detailed explanation of middleware of Gin framework and its application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


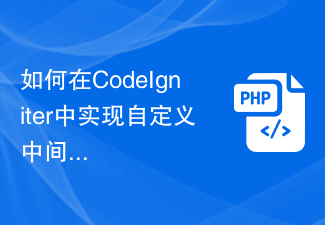
How to implement custom middleware in CodeIgniter Introduction: In modern web development, middleware plays a vital role in applications. They can be used to perform some shared processing logic before or after the request reaches the controller. CodeIgniter, as a popular PHP framework, also supports the use of middleware. This article will introduce how to implement custom middleware in CodeIgniter and provide a simple code example. Middleware overview: Middleware is a kind of request
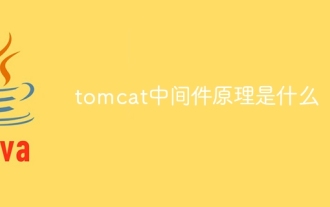
The principle of tomcat middleware is implemented based on Java Servlet and Java EE specifications. As a Servlet container, Tomcat is responsible for processing HTTP requests and responses and providing the running environment for Web applications. The principles of Tomcat middleware mainly involve: 1. Container model; 2. Component architecture; 3. Servlet processing mechanism; 4. Event listening and filters; 5. Configuration management; 6. Security; 7. Clustering and load balancing; 8. Connector technology; 9. Embedded mode, etc.

Implementing user authentication using middleware in the Slim framework With the development of web applications, user authentication has become a crucial feature. In order to protect users' personal information and sensitive data, we need a reliable method to verify the user's identity. In this article, we will introduce how to implement user authentication using the Slim framework’s middleware. The Slim framework is a lightweight PHP framework that provides a simple and fast way to build web applications. One of the powerful features is the middle
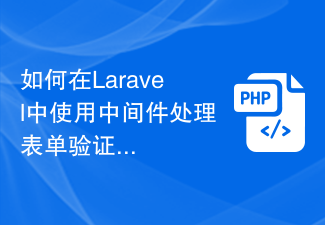
How to use middleware to handle form validation in Laravel, specific code examples are required Introduction: Form validation is a very common task in Laravel. In order to ensure the validity and security of the data entered by users, we usually verify the data submitted in the form. Laravel provides a convenient form validation function and also supports the use of middleware to handle form validation. This article will introduce in detail how to use middleware to handle form validation in Laravel and provide specific code examples.
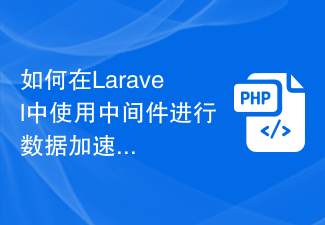
How to use middleware for data acceleration in Laravel Introduction: When developing web applications using the Laravel framework, data acceleration is the key to improving application performance. Middleware is an important feature provided by Laravel that handles requests before they reach the controller or before the response is returned. This article will focus on how to use middleware to achieve data acceleration in Laravel and provide specific code examples. 1. What is middleware? Middleware is a mechanism in the Laravel framework. It is used
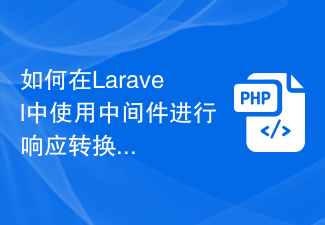
How to use middleware for response conversion in Laravel Middleware is one of the very powerful and practical features in the Laravel framework. It allows us to process requests and responses before the request enters the controller or before the response is sent to the client. In this article, I will demonstrate how to use middleware for response transformation in Laravel. Before starting, make sure you have Laravel installed and a new project created. Now we will follow these steps: Create a new middleware Open
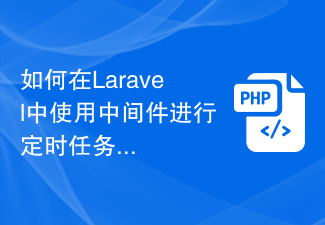
How to use middleware for scheduled task scheduling in Laravel Introduction: Laravel is a popular PHP open source framework that provides convenient and powerful tools to develop web applications. One of the important features is scheduled tasks, which allows developers to run specific tasks at specified intervals. In this article, we will introduce how to use middleware to implement Laravel's scheduled task scheduling, and provide specific code examples. Environment Preparation Before starting, we need to make sure
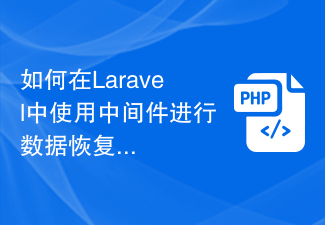
Laravel is a popular PHP web application framework that provides many fast and easy ways to build efficient, secure and scalable web applications. When developing Laravel applications, we often need to consider the issue of data recovery, that is, how to recover data and ensure the normal operation of the application in the event of data loss or damage. In this article, we will introduce how to use Laravel middleware to implement data recovery functions and provide specific code examples. 1. What is Lara?
