PHP Notice: Undefined index: solution in solution
When using PHP language for program development, you often encounter such error message: "PHP Notice: Undefined index". This is a common error message in PHP language, which means that an undefined array subscript or key is operated on, causing the program to fail to execute normally. This article explains the causes and solutions to this error.
1. Cause of the error
Undefined array index or key usually refers to trying to access an element that does not exist in the array, so an "Undefined index" error will occur. This error often occurs when using array type variables in PHP. The program throws this error message due to accessing a non-existent key value.
For example, for the following PHP code:
<?php $arr = array( 'name' => 'Tom', 'age' => 18, 'sex' => 'male' ); echo $arr['hobby']; ?>
After running, you will find the error message: "PHP Notice: Undefined index: hobby". This is because the key value "hobby" is not defined in the array, so the system cannot find its corresponding value.
2. Solution
- Determine whether the element exists
To avoid accessing undefined array subscripts or key values, you can first determine whether the element exists exists. If it does not exist, the processing of the element is skipped. You can use the array_key_exists() function or isset() statement to make a judgment.
<?php $arr = array( 'name' => 'Tom', 'age' => 18, 'sex' => 'male' ); if (array_key_exists('hobby', $arr)) { echo $arr['hobby']; } ?>
If you use the isset() statement, the code is as follows:
<?php $arr = array( 'name' => 'Tom', 'age' => 18, 'sex' => 'male' ); if (isset($arr['hobby'])) { echo $arr['hobby']; } ?>
If "hobby" exists in the array, the corresponding value will be output; if it does not exist, there will be no output.
- Use default values
In order to avoid error messages, you can define default values for certain elements in the array. For example:
<?php $arr = array( 'name' => 'Tom', 'age' => 18, 'sex' => 'male', 'hobby' => '' ); echo $arr['hobby']; ?>
In the above code, the "hobby" element is defined as an empty string to avoid error messages.
- Use the @ symbol
In PHP, you can use the @ symbol to suppress the output of error messages. For example, the above code can be rewritten as follows:
<?php $arr = array( 'name' => 'Tom', 'age' => 18, 'sex' => 'male' ); echo @$arr['hobby']; ?>
If a non-existent key value is accessed, there will be no output.
- Modify the PHP.ini file
If a large number of codes have similar error messages, you can turn off the Notice error message by modifying the PHP.ini file. Find the error_reporting configuration item in the PHP.ini file and modify it to:
error_reporting = E_ALL & ~E_NOTICE
This can turn off Notice-level error messages globally, but it may also block other useful information, so special attention is required.
In short, in the process of program development in PHP language, we should always pay attention to such prompt information and handle it in the correct way to better ensure the normal operation of the program.
The above is the detailed content of PHP Notice: Undefined index: solution in solution. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


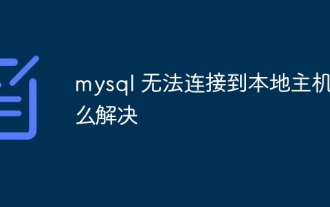
The MySQL connection may be due to the following reasons: MySQL service is not started, the firewall intercepts the connection, the port number is incorrect, the user name or password is incorrect, the listening address in my.cnf is improperly configured, etc. The troubleshooting steps include: 1. Check whether the MySQL service is running; 2. Adjust the firewall settings to allow MySQL to listen to port 3306; 3. Confirm that the port number is consistent with the actual port number; 4. Check whether the user name and password are correct; 5. Make sure the bind-address settings in my.cnf are correct.
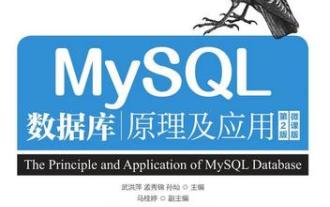
The solution to MySQL installation error is: 1. Carefully check the system environment to ensure that the MySQL dependency library requirements are met. Different operating systems and version requirements are different; 2. Carefully read the error message and take corresponding measures according to prompts (such as missing library files or insufficient permissions), such as installing dependencies or using sudo commands; 3. If necessary, try to install the source code and carefully check the compilation log, but this requires a certain amount of Linux knowledge and experience. The key to ultimately solving the problem is to carefully check the system environment and error information, and refer to the official documents.
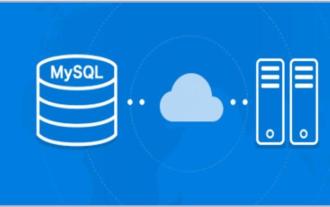
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
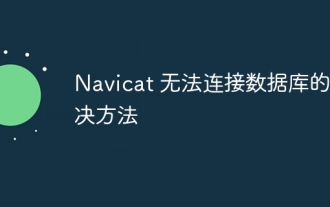
The following steps can be used to resolve the problem that Navicat cannot connect to the database: Check the server connection, make sure the server is running, address and port correctly, and the firewall allows connections. Verify the login information and confirm that the user name, password and permissions are correct. Check network connections and troubleshoot network problems such as router or firewall failures. Disable SSL connections, which may not be supported by some servers. Check the database version to make sure the Navicat version is compatible with the target database. Adjust the connection timeout, and for remote or slower connections, increase the connection timeout timeout. Other workarounds, if the above steps are not working, you can try restarting the software, using a different connection driver, or consulting the database administrator or official Navicat support.
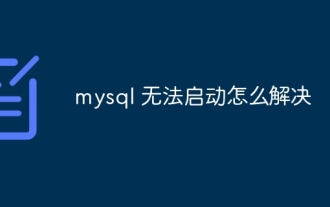
There are many reasons why MySQL startup fails, and it can be diagnosed by checking the error log. Common causes include port conflicts (check port occupancy and modify configuration), permission issues (check service running user permissions), configuration file errors (check parameter settings), data directory corruption (restore data or rebuild table space), InnoDB table space issues (check ibdata1 files), plug-in loading failure (check error log). When solving problems, you should analyze them based on the error log, find the root cause of the problem, and develop the habit of backing up data regularly to prevent and solve problems.
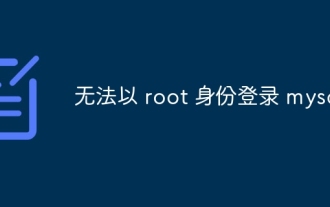
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
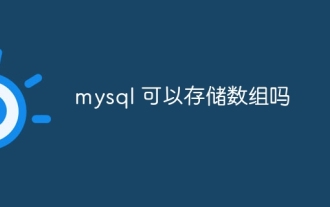
MySQL does not support array types in essence, but can save the country through the following methods: JSON array (constrained performance efficiency); multiple fields (poor scalability); and association tables (most flexible and conform to the design idea of relational databases).
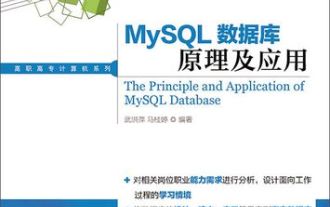
MySQL configuration file corruption can be repaired through the following solutions: 1. Simple fix: If there are only a small number of errors (such as missing semicolons), use a text editor to correct it, and be sure to back up before modifying; 2. Complete reconstruction: If the corruption is serious or the configuration file cannot be found, refer to the official document or copy the default configuration file of the same version, and then modify it according to the needs; 3. Use the installation program to provide repair function: Try to automatically repair the configuration file using the repair function provided by the installer. After selecting the appropriate solution to repair it, you need to restart the MySQL service and verify whether it is successful and develop good backup habits to prevent such problems.
