


Database query result volume and reasonable processing of result sets: application in PHP programming
With the development of Internet technology, databases play an increasingly important role in website development. In data query, sometimes the query results are very large or even exceed the server memory limit. This requires us to process the query results reasonably, not only to ensure query speed and accuracy, but also to avoid server crashes. So, in PHP programming, how can we better handle result sets?
1. Control and optimization of result sets
Generally, in PHP, we can optimize the result set through some means. Such as LIMIT keyword, its function is to limit the number of query result sets. Its syntax is as follows:
SELECT * FROM table_name LIMIT start, length
where start indicates the starting row number of the query, and length indicates the number of records to be returned. This statement is very useful for paginated display to ensure that the server is not burdened by too many queries.
In addition to LIMIT, we can also reduce the size of the result set by optimizing query statements. Indexes can be used to improve query speed, avoid full table scans, and reduce server pressure.
2. Memory control of result set
In PHP, query results are stored in memory by default. Even a huge result set will be loaded into memory at once. This It is easy to cause the server's memory to overflow. Therefore, we need to process the result set in chunks, read a certain amount of data at a time, and load it dynamically to ensure that the program does not occupy all the memory.
For this, in PHP, we can use the fetch method in the PDO class. This method returns an associative array by default, representing all columns of the current row. We can specify the returned data structure by modifying the parameter type of fetch. For example, PDO::FETCH_OBJ means returning a result set in the form of an object, and PDO::FETCH_NUM means returning a result set with a numerical index. Among them, PDO::FETCH_BOTH means returning a mixed result set of two result sets.
If we want to load the result set in chunks, we can use the following method:
$limit = 1000; // 每次查询的记录数 $sql = "SELECT * FROM table_name"; $stmt = $pdo->prepare($sql); $stmt->execute(); while($row = $stmt->fetch(PDO::FETCH_OBJ, PDO::FETCH_ORI_NEXT, $limit)) { // 处理当前行的数据 }
The above code can ensure that the result set obtained each time has only $limit records at most.
3. Caching of result sets
In large websites, the result set usually does not expire immediately. Therefore, we can avoid repeated queries and improve performance through result set caching.
In PHP, you can use Memcached as a cache storage method to store the query result set in memory, and you can obtain it directly from the memory the next time you query.
To do this, we need to install and start the Memcached service, and then store the result set in Memcached. The specific implementation is as follows:
// 首先判断缓存是否存在 if($cache->has('result_cache')) { // 从缓存中加载结果集 $result = unserialize($cache->get('result_cache')); } else { // 执行查询 $sql = "SELECT * FROM table_name"; $stmt = $pdo->prepare($sql); $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); // 将结果集存入缓存 $cache->set('result_cache', serialize($result), 60); // 缓存生存时间60秒 } // 处理结果集 foreach($result as $row) { // 处理当前行的数据 }
In the above code, we first try to obtain the result set from the Memcached cache. If the cache exists, the cached data is read directly. If the cache does not exist, the query operation is executed and the result set is stored in the cache.
To sum up, in PHP programming, we can use the above methods to control and optimize the result set, dynamically control the memory, and use caching to improve query efficiency and ensure the stability and reliability of the server.
The above is the detailed content of Database query result volume and reasonable processing of result sets: application in PHP programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


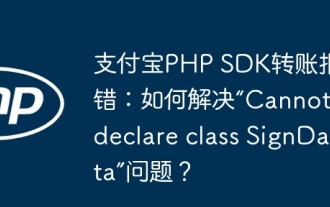
Alipay PHP...
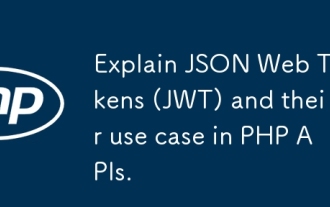
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
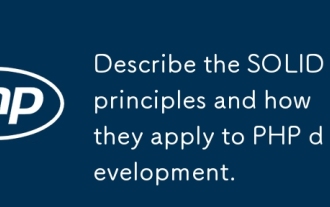
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
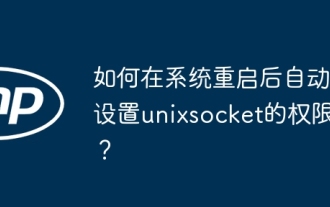
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
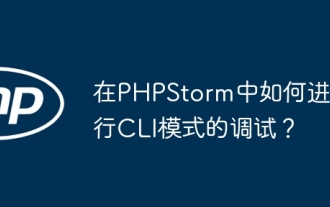
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
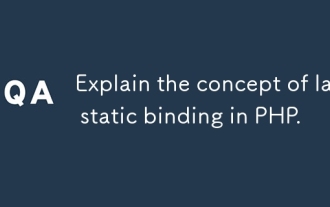
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
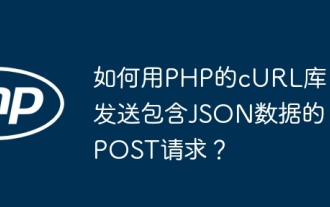
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
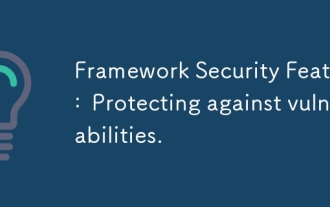
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
