Using JWT to implement authentication in Beego
With the rapid development of the Internet and mobile Internet, more and more applications require authentication and permission control, and JWT (JSON Web Token), as a lightweight authentication and authorization mechanism, is used in WEB applications is widely used in.
Beego is an MVC framework based on the Go language, which has the advantages of efficiency, simplicity, and scalability. This article will introduce how to use JWT to implement authentication in Beego.
1. Introduction to JWT
JSON Web Token (JWT) is an open standard (RFC 7519) for transmitting identity and claim information over the network. It can securely transfer information between various systems because it can encrypt and digitally sign information. A JWT consists of three parts: header, claim and signature. Where headers and claims are encoded using base64, the signature uses a key to encrypt the data.
2. Beego integrates JWT
1. Install dependencies
First we need to install two dependency packages:
go get github.com/dgrijalva/ jwt-go
go get github.com/astaxie/beego
2. Create a JWT tool class
We can create a JWT tool class by encapsulating the JWT operation for generating , verify JWT and other operations. These include methods such as issuing tokens, verifying tokens, and obtaining information stored in tokens. The code is as follows:
package utils import ( "errors" "github.com/dgrijalva/jwt-go" "time" ) // JWT构造体 type JWT struct { signingKey []byte } // 定义JWT参数 type CustomClaims struct { UserID string `json:"userId"` UserName string `json:"userName"` jwt.StandardClaims } // 构造函数 func NewJWT() *JWT { return &JWT{ []byte("jwt-secret-key"), } } // 生成token func (j *JWT) CreateToken(claims CustomClaims) (string, error) { token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims) return token.SignedString(j.signingKey) } // 解析token func (j *JWT) ParseToken(tokenString string) (*CustomClaims, error) { token, err := jwt.ParseWithClaims(tokenString, &CustomClaims{}, func(token *jwt.Token) (interface{}, error) { if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok { return nil, errors.New("签名方法不正确") } return j.signingKey, nil }) if err != nil { return nil, err } if claims, ok := token.Claims.(*CustomClaims); ok && token.Valid { return claims, nil } return nil, errors.New("无效的token") }
3. Use JWT for authentication
In Beego, we can use middleware to verify the user's identity, for example:
package controllers import ( "myProject/utils" "github.com/astaxie/beego" "github.com/dgrijalva/jwt-go" ) type BaseController struct { beego.Controller } type CustomClaims struct { UserID string `json:"userId"` UserName string `json:"userName"` jwt.StandardClaims } func (c *BaseController) Prepare() { // 获取请求头中的token tokenString := c.Ctx.Request.Header.Get("Authorization") // 创建JWT实例 jwt := utils.NewJWT() // 解析token,获取token中存储的用户信息 claims, err := jwt.ParseToken(tokenString) if err != nil { c.Data["json"] = "无效的token" c.ServeJSON() return } // 验证token中的用户信息 if claims.UserID != "123456" || claims.UserName != "test" { c.Data["json"] = "用户信息验证失败" c.ServeJSON() return } }
In In the above code, we first obtain the token in the request header, and then parse the token through JWT to obtain the user information stored in it. Finally, we verify the user information in the token with the user information stored in our database. Only after passing the verification can we access the relevant interfaces normally.
3. Summary
Through the above steps, we have successfully integrated the JWT authentication mechanism and implemented user identity verification, permission control and other operations in the Beego application. However, it should be noted that in actual applications, we need to ensure the security of the JWT key, and we also need to consider whether the information stored in the JWT is reasonable.
The above is the detailed content of Using JWT to implement authentication in Beego. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


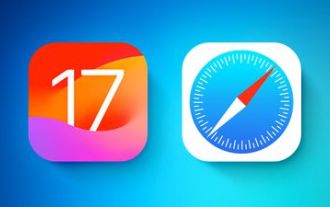
In iOS 17, Apple introduced several new privacy and security features to its mobile operating system, one of which is the ability to require two-step authentication for private browsing tabs in Safari. Here's how it works and how to turn it off. On an iPhone or iPad running iOS 17 or iPadOS 17, if you have any Private Browsing tab open in Safari and then exit the session or app, Apple's browser now requires Face ID/TouchID authentication or a passcode to access again they. In other words, if someone gets their hands on your iPhone or iPad while it's unlocked, they still won't be able to view it without knowing your passcode
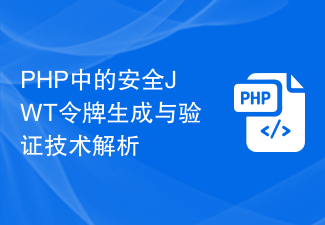
Analysis of Secure JWT Token Generation and Verification Technology in PHP With the development of network applications, user authentication and authorization are becoming more and more important. JsonWebToken (JWT) is an open standard (RFC7519) for securely transmitting information in web applications. In PHP development, it has become a common practice to use JWT tokens for user authentication and authorization. This article will introduce secure JWT token generation and verification technology in PHP. 1. Basic knowledge of JWT in understanding how to generate and
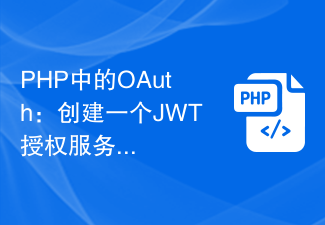
OAuth in PHP: Creating a JWT authorization server With the rise of mobile applications and the trend of separation of front-end and back-end, OAuth has become an indispensable part of modern web applications. OAuth is an authorization protocol that protects users' resources from unauthorized access by providing standardized processes and mechanisms. In this article, we will learn how to create a JWT (JSONWebTokens) based OAuth authorization server using PHP. JWT is a type of

Implementing user authentication using middleware in the Slim framework With the development of web applications, user authentication has become a crucial feature. In order to protect users' personal information and sensitive data, we need a reliable method to verify the user's identity. In this article, we will introduce how to implement user authentication using the Slim framework’s middleware. The Slim framework is a lightweight PHP framework that provides a simple and fast way to build web applications. One of the powerful features is the middle

In today's era of rapid technological development, programming languages are springing up like mushrooms after a rain. One of the languages that has attracted much attention is the Go language, which is loved by many developers for its simplicity, efficiency, concurrency safety and other features. The Go language is known for its strong ecosystem with many excellent open source projects. This article will introduce five selected Go language open source projects and lead readers to explore the world of Go language open source projects. KubernetesKubernetes is an open source container orchestration engine for automated
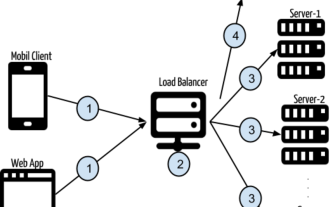
Authentication is one of the most important parts of any web application. This tutorial discusses token-based authentication systems and how they differ from traditional login systems. By the end of this tutorial, you will see a fully working demo written in Angular and Node.js. Traditional Authentication Systems Before moving on to token-based authentication systems, let’s take a look at traditional authentication systems. The user provides their username and password in the login form and clicks Login. After making the request, authenticate the user on the backend by querying the database. If the request is valid, a session is created using the user information obtained from the database, and the session information is returned in the response header so that the session ID is stored in the browser. Provides access to applications subject to

With the rapid development of the Internet, more and more enterprises have begun to migrate their applications to cloud platforms. Docker and Kubernetes have become two very popular and powerful tools for application deployment and management on cloud platforms. Beego is a web framework developed using Golang. It provides rich functions such as HTTP routing, MVC layering, logging, configuration management, Session management, etc. In this article we will cover how to use Docker and Kub

How to use JWT to implement authentication and authorization in PHP applications Introduction: With the rapid development of the Internet, authentication and authorization are becoming increasingly important in web applications. JSONWebToken (JWT) is a popular authentication and authorization mechanism that is widely used in PHP applications. This article will introduce how to use JWT to implement authentication and authorization in PHP applications, and provide code examples to help readers better understand the use of JWT. 1. Introduction to JWT JSONWebTo
