Using Gin framework to implement two-factor authentication function
Two-factor authentication has become an essential feature of network security, which can greatly enhance the security of your account. In this article, we will introduce how to use the Gin framework to implement two-factor authentication functionality.
The Gin framework is a lightweight web framework that has the advantages of high performance, ease of use and flexibility. It supports RESTful API, middleware, routing groups, template rendering and other functions, and has good documentation and examples, making it one of the most popular Go language web frameworks.
Before you start, please make sure you have installed the Go language development environment and configured the corresponding GOPATH and PATH environment variables.
First, we need to create a new Gin project. Enter the following command on the command line:
$ mkdir gin-auth $ cd gin-auth $ go mod init gin-auth
Next, we need to install the Gin framework and other dependent packages. Type the following command in the console:
$ go get -u github.com/gin-gonic/gin $ go get -u github.com/gin-contrib/sessions $ go get -u github.com/google/uuid
gin
is the Gin framework itself.gin-contrib/sessions
is the Session middleware of the Gin framework, used to handle Session-related tasks.uuid
is a Go language library developed by Google for generating UUID. Used in this article to generate two-factor authentication verification codes.
Now, we can start implementing our two-factor authentication feature.
We first need to write the login page and the code to handle the login request, as shown below:
package main import ( "net/http" "github.com/gin-contrib/sessions" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // 使用sessions中间件 store := sessions.NewCookieStore([]byte("secret")) router.Use(sessions.Sessions("mysession", store)) router.GET("/", func(c *gin.Context) { c.HTML(http.StatusOK, "login.html", nil) }) router.POST("/", func(c *gin.Context) { username := c.PostForm("username") password := c.PostForm("password") // TODO: 验证用户名和密码是否正确 // 将用户名保存到Session中 session := sessions.Default(c) session.Set("username", username) session.Save() c.Redirect(http.StatusFound, "/second-factor") }) router.Run(":8080") }
In the above code, we use the Gin framework's gin.Default()
Function creates a basic router. Then, we use the sessions.NewCookieStore
function to create a Cookie Store that stores Session to store the user's Session information. We used Session middleware in the router middleware and named it mysession
.
In the home page routing, we will render the login page through the c.HTML
function. In the login route, we get the username and password entered by the user and then validate them in a function we implement later. If the authentication is successful, we save the username in the Session and redirect the user to the second authentication page.
Next, we will write the page and function for the second authentication. Here, we verify whether we have logged in through Session. If logged in, the secondary authentication page is displayed and a random 6-digit verification code is generated. The verification code will be saved in the Session and sent to the user via SMS, email, or security token.
// 定义一个中间件,用于检查Session中是否保存了该用户的用户名 func AuthRequired() gin.HandlerFunc { return func(c *gin.Context) { session := sessions.Default(c) username := session.Get("username") if username == nil { c.Redirect(http.StatusFound, "/") return } c.Next() } } func generateCode() string { code := uuid.New().String() code = strings.ReplaceAll(code, "-", "") code = code[:6] return code } func sendCode(username string, code string) error { // TODO: 将验证码发送给用户 return nil } func main() { router := gin.Default() // ... router.GET("/second-factor", AuthRequired(), func(c *gin.Context) { session := sessions.Default(c) username := session.Get("username").(string) code := generateCode() // 将二次认证验证码保存到Session session.Set("second-factor-code", code) session.Save() // 发送二次认证验证码 err := sendCode(username, code) if err != nil { c.String(http.StatusInternalServerError, "发送二次认证验证码失败") } // 渲染二次认证视图 c.HTML(http.StatusOK, "second-factor.html", nil) }) router.POST("/second-factor", AuthRequired(), func(c *gin.Context) { session := sessions.Default(c) code := session.Get("second-factor-code").(string) inputCode := c.PostForm("code") // 验证二次认证验证码是否正确 if code != inputCode { c.String(http.StatusBadRequest, "验证码不正确") return } c.Redirect(http.StatusFound, "/dashboard") }) router.Run(":8080") }
In the above code, we define a middleware named AuthRequired
to check whether a logged-in user exists in the Session. In our second route, we use this middleware to redirect the user to the login page if the logged in user is not found in the Session.
We use a function called generateCode
to generate a 6-digit verification code and use the sendCode
function to send that verification code to the user. In our actual application, this verification code can be sent using SMS, email, or security token.
We use a POST request and the token in the second route to verify whether the user's secondary authentication code is correct. If the authentication code is correct, the user is redirected to the control panel page.
代码已经完成了,现在可以创建一些模板文件来呈现登录、二次验证和控制面板页面了。下面是示例模板文件,你可以根据自身需求对其进行修改。
<meta charset="UTF-8"> <title>Login</title>
<form method="post" action="/"> <label> 用户名: <input type="text" name="username" /> </label> <br /> <label> 密码: <input type="password" name="password" /> </label> <br /> <button type="submit">登录</button> </form>
<meta charset="UTF-8"> <title>Second Factor Authentication</title>
head>
<form method="post" action="/second-factor"> <p> 请验证您的身份。 </p> <p> 一个6位数字的验证码已经发送到您的邮件或手机上。 <br /> 请输入该验证码以完成二次认证: </p> <label> 验证码: <input type="text" name="code" /> </label> <br /> <button type="submit">验证</button> </form>
<meta charset="UTF-8"> <title>Dashboard</title>
<h1>欢迎访问控制面板</h1>
现在,我们的Gin应用程序就已经完成了。它使用Session中间件实现了用户认证机制,并使用了二次认证功能来增强安全性。
The above is the detailed content of Using Gin framework to implement two-factor authentication function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


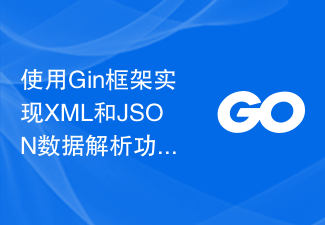
In the field of web development, XML and JSON, one of the data formats, are widely used, and the Gin framework is a lightweight Go language web framework that is simple, easy to use and has efficient performance. This article will introduce how to use the Gin framework to implement XML and JSON data parsing functions. Gin Framework Overview The Gin framework is a web framework based on the Go language, which can be used to build efficient and scalable web applications. The Gin framework is designed to be simple and easy to use. It provides a variety of middleware and plug-ins to make the development
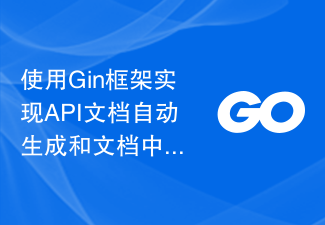
With the continuous development of Internet applications, the use of API interfaces is becoming more and more popular. During the development process, in order to facilitate the use and management of interfaces, the writing and maintenance of API documents has become increasingly important. The traditional way of writing documents requires manual maintenance, which is inefficient and error-prone. In order to solve these problems, many teams have begun to use automatic generation of API documents to improve development efficiency and code quality. In this article, we will introduce how to use the Gin framework to implement automatic generation of API documents and document center functions. Gin is one

With the rapid development of web applications, more and more enterprises tend to use Golang language for development. In Golang development, using the Gin framework is a very popular choice. The Gin framework is a high-performance web framework that uses fasthttp as the HTTP engine and has a lightweight and elegant API design. In this article, we will delve into the application of reverse proxy and request forwarding in the Gin framework. The concept of reverse proxy The concept of reverse proxy is to use the proxy server to make the client

How to use Laravel to implement image processing functions requires specific code examples. Nowadays, with the development of the Internet, image processing has become an indispensable part of website development. Laravel is a popular PHP framework that provides us with many convenient tools to process images. This article will introduce how to use Laravel to implement image processing functions, and give specific code examples. Install LaravelInterventionImageInterven
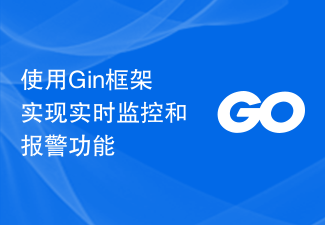
Gin is a lightweight Web framework that uses the coroutine and high-speed routing processing capabilities of the Go language to quickly develop high-performance Web applications. In this article, we will explore how to use the Gin framework to implement real-time monitoring and alarm functions. Monitoring and alarming are an important part of modern software development. In a large system, there may be thousands of processes, hundreds of servers, and millions of users. The amount of data generated by these systems is often staggering, so there is a need for a system that can quickly process this data and provide timely warnings.
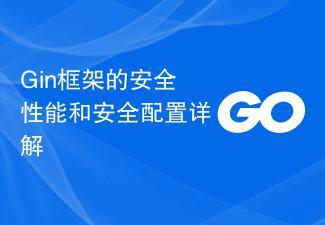
The Gin framework is a lightweight web development framework based on the Go language and provides excellent features such as powerful routing functions, middleware support, and scalability. However, security is a crucial factor for any web application. In this article, we will discuss the security performance and security configuration of the Gin framework to help users ensure the security of their web applications. 1. Security performance of Gin framework 1.1 XSS attack prevention Cross-site scripting (XSS) attack is the most common Web
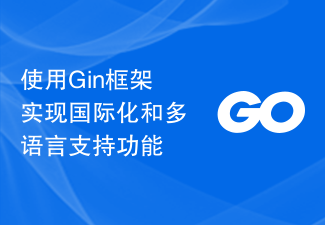
With the development of globalization and the popularity of the Internet, more and more websites and applications have begun to strive to achieve internationalization and multi-language support functions to meet the needs of different groups of people. In order to realize these functions, developers need to use some advanced technologies and frameworks. In this article, we will introduce how to use the Gin framework to implement internationalization and multi-language support capabilities. The Gin framework is a lightweight web framework written in Go language. It is efficient, easy to use and flexible, and has become the preferred framework for many developers. besides,
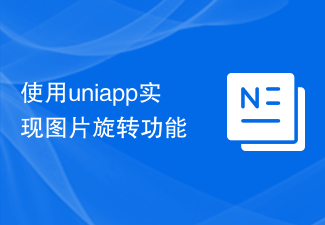
Using uniapp to implement image rotation function In mobile application development, we often encounter scenarios where images need to be rotated. For example, the angle needs to be adjusted after taking a photo, or an effect similar to the rotation of a camera after taking a photo is achieved. This article will introduce how to use the uniapp framework to implement the image rotation function and provide specific code examples. uniapp is a cross-platform development framework based on Vue.js, which can simultaneously develop and publish applications for iOS, Android, H5 and other platforms. Implemented in uniapp
