Data analysis using Pig and Hive in Beego
With the continuous advancement of data collection and storage technology, enterprises have more and more data resources. But how to efficiently perform data analysis and mining is still a problem worthy of study. In this article, we will introduce how to combine Pig and Hive for data analysis in the Beego framework.
- Introduction to Beego framework
Beego is a framework for rapid development of web applications. It is developed using the MVC pattern and Go language. Beego framework is lightweight, efficient, easy to use, and rapid development. It is currently one of the mainstream frameworks for developing web applications in Go language. Beego framework has built-in ORM, Session, Cache and other functions, and also supports the use of third-party libraries.
- Pig Introduction
Pig is a data stream processing framework that can efficiently process data in Hadoop. Pig provides a SQL-like language that can easily query, filter and transform data. Pig also supports custom functions and MapReduce operations, which can meet various complex data processing needs.
- Hive Introduction
Hive is a data warehouse tool that can store structured data in Hadoop and provide a SQL-like query language for query and analysis . Hive supports multiple data sources, including HDFS, HBase and local file systems. Hive's query language uses SQL-like HiveQL, which can facilitate data analysis and mining.
- Steps to use Pig and Hive for data analysis in Beego
(1) Install and configure Hadoop, Hive and Pig
First you need to configure the server Installing and configuring Hadoop, Hive and Pig on the Internet will not be introduced here.
(2) Connect to Hive
Beego has a built-in go-hive library, which can easily connect to Hive. When using the go-hive library, you need to introduce the following package into the code:
import ( "github.com/ziutek/mymysql/autorc" "hive" "time" )
Among them, the hive package provides related functions and structures for Hive connection. The sample code for using Hive connection is as follows:
cfg := hive.NewConfig() cfg.Addr = "127.0.0.1:10000" cfg.Timeout = 5 * time.Second cfg.User = "hive" cfg.Passwd = "" cfg.Database = "default" db, err := hive.Open(cfg) if err != nil { log.Fatal(err) } defer db.Close() //查询操作 rows, _, err := db.Query("select * from tablename limit 1000") if err != nil { log.Fatal(err) } for _, row := range rows { //输出查询结果 fmt.Println(row) }
(3) Using Pig for data processing
Beego has a built-in exec package, which can easily execute Pig scripts. When using the exec package, you need to introduce the following package into the code:
import ( "exec" "os" )
The sample code for using the exec package to execute the Pig script is as follows:
//打开Pig脚本文件 file, err := os.Open("pigscript.pig") if err != nil { log.Fatal(err) } defer file.Close() //执行Pig脚本 cmd := exec.Command("pig") cmd.Stdin = file err = cmd.Run() if err != nil { log.Fatal(err) }
(4) Combine Pig and Hive for data processing
Pig and Hive are both tools for data processing on Hadoop, and they can easily interact with each other. Data interaction between Pig and Hive can be easily achieved using Beego. For example, we can use Pig for data cleaning and transformation, and then store the results into Hive for analysis and mining. The sample code is as follows:
//执行Pig脚本 cmd := exec.Command("pig", "-param", "input=input.csv", "-param", "output=output", "pigscript.pig") err := cmd.Run() if err != nil { log.Fatal(err) } //连接Hive cfg := hive.NewConfig() cfg.Addr = "127.0.0.1:10000" cfg.Timeout = 5 * time.Second cfg.User = "hive" cfg.Passwd = "" cfg.Database = "default" db, err := hive.Open(cfg) if err != nil { log.Fatal(err) } defer db.Close() //查询Pig处理结果 rows, _, err := db.Query("select * from output") if err != nil { log.Fatal(err) } for _, row := range rows { //输出查询结果 fmt.Println(row) }
- Summary
Combining Pig and Hive for data analysis in the Beego framework can easily process and analyze massive data resources and give full play to the data. value. At the same time, the efficiency and ease of use of the Beego framework also provide good support and guarantee for data analysis.
The above is the detailed content of Data analysis using Pig and Hive in Beego. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




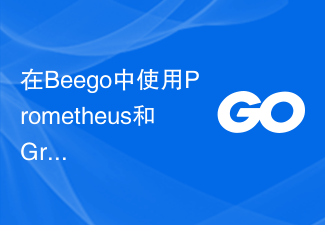
With the rise of cloud computing and microservices, application complexity has increased. Therefore, monitoring and diagnostics become one of the important development tasks. In this regard, Prometheus and Grafana are two popular open source monitoring and visualization tools that can help developers better monitor and analyze applications. This article will explore how to use Prometheus and Grafana to implement monitoring and alarming in the Beego framework. 1. Introduction Beego is an open source rapid development web application.
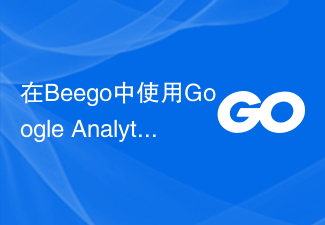
With the rapid development of the Internet, the use of Web applications is becoming more and more common. How to monitor and analyze the usage of Web applications has become a focus of developers and website operators. Google Analytics is a powerful website analytics tool that can track and analyze the behavior of website visitors. This article will introduce how to use Google Analytics in Beego to collect website data. 1. To register a Google Analytics account, you first need to
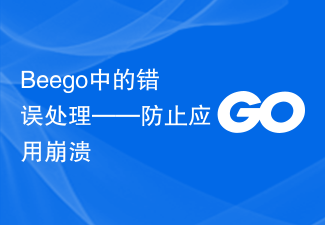
In the Beego framework, error handling is a very important part, because if the application does not have a correct and complete error handling mechanism, it may cause the application to crash or not run properly, which is both for our projects and users. A very serious problem. The Beego framework provides a series of mechanisms to help us avoid these problems and make our code more robust and maintainable. In this article, we will introduce the error handling mechanisms in the Beego framework and discuss how they can help us avoid
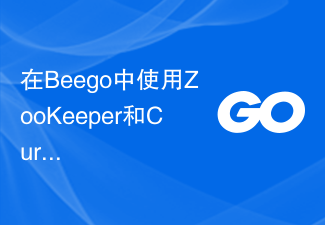
With the rapid development of the Internet, distributed systems have become one of the infrastructures in many enterprises and organizations. For a distributed system to function properly, it needs to be coordinated and managed. In this regard, ZooKeeper and Curator are two tools worth using. ZooKeeper is a very popular distributed coordination service that can help us coordinate the status and data between nodes in a cluster. Curator is an encapsulation of ZooKeeper

In today's era of rapid technological development, programming languages are springing up like mushrooms after a rain. One of the languages that has attracted much attention is the Go language, which is loved by many developers for its simplicity, efficiency, concurrency safety and other features. The Go language is known for its strong ecosystem with many excellent open source projects. This article will introduce five selected Go language open source projects and lead readers to explore the world of Go language open source projects. KubernetesKubernetes is an open source container orchestration engine for automated

With the rapid development of the Internet, more and more enterprises have begun to migrate their applications to cloud platforms. Docker and Kubernetes have become two very popular and powerful tools for application deployment and management on cloud platforms. Beego is a web framework developed using Golang. It provides rich functions such as HTTP routing, MVC layering, logging, configuration management, Session management, etc. In this article we will cover how to use Docker and Kub
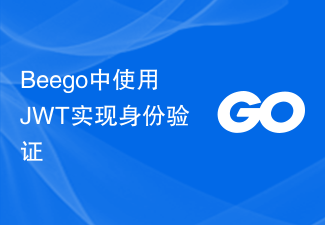
With the rapid development of the Internet and mobile Internet, more and more applications require authentication and permission control, and JWT (JSON Web Token), as a lightweight authentication and authorization mechanism, is widely used in WEB applications. Beego is an MVC framework based on the Go language, which has the advantages of efficiency, simplicity, and scalability. This article will introduce how to use JWT to implement authentication in Beego. 1. Introduction to JWT JSONWebToken (JWT) is a
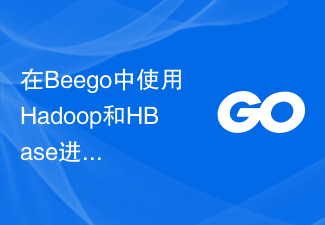
With the advent of the big data era, data processing and storage have become more and more important, and how to efficiently manage and analyze large amounts of data has become a challenge for enterprises. Hadoop and HBase, two projects of the Apache Foundation, provide a solution for big data storage and analysis. This article will introduce how to use Hadoop and HBase in Beego for big data storage and query. 1. Introduction to Hadoop and HBase Hadoop is an open source distributed storage and computing system that can
