


Detailed explanation of the configuration file parser of the Gin framework and its application
Gin is a lightweight web application framework based on Go language, which is very popular among developers in web development. One of the important reasons is that Gin provides rich middleware, making developing web applications more efficient and convenient. In Gin, the use of configuration files is also very common. This article will introduce the configuration file parser of the Gin framework in detail and discuss its applications.
1. The role of configuration files
In web applications, configuration files are very important. They store various settings of the application, including database connections, logging, security settings, etc. . Configuration files usually exist in the form of text files. You can change the settings of the application by modifying the configuration file without recompiling the application.
2. Configuration file of Gin framework
In Gin framework, if you need to use the configuration file, you need to manually parse it yourself. The Gin framework has a built-in simple configuration file parser that can be used to parse common configuration file formats, such as JSON, TOML, YAML, etc. We can load the configuration file through the following code:
import ( "github.com/gin-gonic/gin" "github.com/spf13/viper" ) func main() { router := gin.Default() // 读取配置文件 viper.SetConfigFile("config.yaml") err := viper.ReadInConfig() if err != nil { panic(fmt.Errorf("Fatal error config file: %s ", err)) } // 设置路由组 api := router.Group("/api") { api.GET("/user", getUser) } }
In the above code, we can see that the configuration file is stored in the config.yaml file in YAML format. Configuration files are loaded and parsed through the SetConfigFile() and ReadInConfig() methods of the viper library. If there is an error loading the configuration file, an exception will be thrown and the program will stop running.
3. Configuration file parser of Gin framework
The Gin framework has a built-in configuration file parser that can be used to parse various common configuration file formats. The parser uses the spf13/viper library, which supports configuration files in multiple formats and provides a rich API to facilitate configuration reading in applications.
In the Gin framework, we can use the viper library to read and parse configuration files. For details, please refer to the following code:
import ( "github.com/spf13/viper" ) // 读取配置文件 viper.SetConfigFile("config.yaml") err := viper.ReadInConfig() if err != nil { panic(fmt.Errorf("Fatal error config file: %s ", err)) } // 读取配置项 databaseURL := viper.GetString("database.url") maxConnections := viper.GetInt("database.maxConnections")
In the above code, we can see that the viper library provides many methods to read and parse configuration files. We first specify the location of the configuration file through the SetConfigFile() method, and then call the ReadInConfig() method to read and parse the configuration file. If parsing fails, an exception occurs and the application stops running.
4. Application of Gin framework configuration file parser
- Set the log level through the configuration file
In the Gin framework, we can use the configuration file to set the log level to flexibly control the detail of the log output. The following is an example:
import ( "github.com/gin-gonic/gin" "github.com/spf13/viper" ) func main() { router := gin.Default() // 读取配置文件 viper.SetConfigFile("config.yaml") err := viper.ReadInConfig() if err != nil { panic(fmt.Errorf("Fatal error config file: %s ", err)) } // 设置日志级别 gin.SetMode(viper.GetString("log.level")) // 设置路由组 api := router.Group("/api") { api.GET("/user", getUser) } }
In the above code, we first configure the log level into the config.yaml file and read it through the viper library. Then, use the gin.SetMode() method to set the log level.
- Set the database connection through the configuration file
In the Gin framework, we can set the database connection through the configuration file, making it more convenient to manage the database connection. The following is an example:
import ( "database/sql" "fmt" "github.com/gin-gonic/gin" "github.com/spf13/viper" _ "github.com/go-sql-driver/mysql" ) func main() { router := gin.Default() // 读取配置文件 viper.SetConfigFile("config.yaml") err := viper.ReadInConfig() if err != nil { panic(fmt.Errorf("Fatal error config file: %s ", err)) } // 获取配置项 dbURL := viper.GetString("database.url") // 连接数据库 db, err := sql.Open("mysql", dbURL) if err != nil { panic(fmt.Errorf("Fatal error Connection String: %s ", err)) } // 设置路由组 api := router.Group("/api") { api.GET("/user", getUser) } }
In the above code, we can see that we first configure the database connection into the config.yaml file and read it through the viper library. Then, connect to the database through the sql.Open() method. If the connection fails, the program will stop running.
5. Summary
This article introduces the configuration file parser and its application of the Gin framework. Configuration files can be easily read and parsed through the viper library, which can make our web applications more flexible, efficient and convenient. Therefore, in actual development, we should make full use of the configuration file parser of the Gin framework and centralize the application settings and configuration in the configuration file to facilitate our unified management of the application.
The above is the detailed content of Detailed explanation of the configuration file parser of the Gin framework and its application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


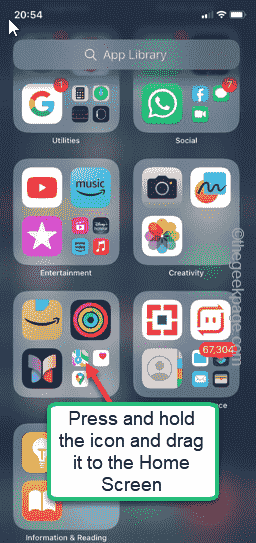
Deleted something important from your home screen and trying to get it back? You can put app icons back on the screen in a variety of ways. We have discussed all the methods you can follow and put the app icon back on the home screen. How to Undo Remove from Home Screen in iPhone As we mentioned before, there are several ways to restore this change on iPhone. Method 1 – Replace App Icon in App Library You can place an app icon on your home screen directly from the App Library. Step 1 – Swipe sideways to find all apps in the app library. Step 2 – Find the app icon you deleted earlier. Step 3 – Simply drag the app icon from the main library to the correct location on the home screen. This is the application diagram
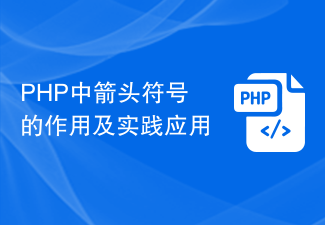
The role and practical application of arrow symbols in PHP In PHP, the arrow symbol (->) is usually used to access the properties and methods of objects. Objects are one of the basic concepts of object-oriented programming (OOP) in PHP. In actual development, arrow symbols play an important role in operating objects. This article will introduce the role and practical application of arrow symbols, and provide specific code examples to help readers better understand. 1. The role of the arrow symbol to access the properties of an object. The arrow symbol can be used to access the properties of an object. When we instantiate a pair
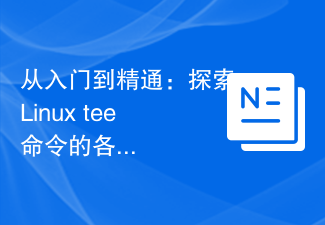
The Linuxtee command is a very useful command line tool that can write output to a file or send output to another command without affecting existing output. In this article, we will explore in depth the various application scenarios of the Linuxtee command, from entry to proficiency. 1. Basic usage First, let’s take a look at the basic usage of the tee command. The syntax of tee command is as follows: tee[OPTION]...[FILE]...This command will read data from standard input and save the data to
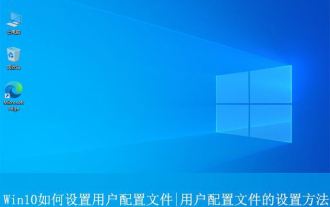
Recently, many Win10 system users want to change the user profile, but they don’t know how to do it. This article will show you how to set the user profile in Win10 system! How to set up user profile in Win10 1. First, press the "Win+I" keys to open the settings interface, and click to enter the "System" settings. 2. Then, in the opened interface, click "About" on the left, then find and click "Advanced System Settings". 3. Then, in the pop-up window, switch to the "" option bar and click "User Configuration" below.

Helm is an important component of Kubernetes that simplifies the deployment of Kubernetes applications by bundling configuration files into a package called HelmChart. This approach makes updating a single configuration file more convenient than modifying multiple files. With Helm, users can easily deploy Kubernetes applications, simplifying the entire deployment process and improving efficiency. In this guide, I'll cover different ways to implement Helm on Ubuntu. Please note: The commands in the following guide apply to Ubuntu 22.04 as well as all Ubuntu versions and Debian-based distributions. These commands are tested and should work correctly on your system. in U
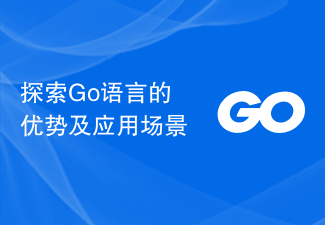
The Go language is an open source programming language developed by Google and first released in 2007. It is designed to be a simple, easy-to-learn, efficient, and highly concurrency language, and is favored by more and more developers. This article will explore the advantages of Go language, introduce some application scenarios suitable for Go language, and give specific code examples. Advantages: Strong concurrency: Go language has built-in support for lightweight threads-goroutine, which can easily implement concurrent programming. Goroutin can be started by using the go keyword
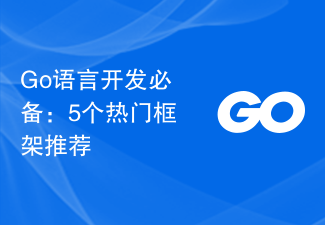
"Go Language Development Essentials: 5 Popular Framework Recommendations" As a fast and efficient programming language, Go language is favored by more and more developers. In order to improve development efficiency and optimize code structure, many developers choose to use frameworks to quickly build applications. In the world of Go language, there are many excellent frameworks to choose from. This article will introduce 5 popular Go language frameworks and provide specific code examples to help readers better understand and use these frameworks. 1.GinGin is a lightweight web framework with fast
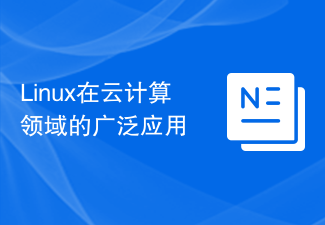
The wide application of Linux in the field of cloud computing With the continuous development and popularization of cloud computing technology, Linux, as an open source operating system, plays an important role in the field of cloud computing. Due to its stability, security and flexibility, Linux systems are widely used in various cloud computing platforms and services, providing a solid foundation for the development of cloud computing technology. This article will introduce the wide range of applications of Linux in the field of cloud computing and give specific code examples. 1. Application virtualization technology of Linux in cloud computing platform Virtualization technology
