


Use the Gin framework to implement real-time monitoring and alarm functions
Gin is a lightweight Web framework that uses the coroutine and high-speed routing processing capabilities of the Go language to quickly develop high-performance Web applications. In this article, we will explore how to use the Gin framework to implement real-time monitoring and alarm functions.
Monitoring and alarming are important parts of modern software development. In a large system, there may be thousands of processes, hundreds of servers, and millions of users. The amount of data generated by these systems is often staggering, so a method that can quickly process this data and promptly alert system administrators is needed.
The following are the steps to use the Gin framework to implement real-time monitoring and alarm functions:
1. Set up routing
First, we need to set up a route to handle requests from the client. Using the Gin framework, we can easily define a route:
router := gin.Default() router.POST("/monitor", monitorHandler)
In the above code, we define a POST request whose path is "/monitor" and hand the request to the monitorHandler named Processor function to handle.
2. Processing requests
Next, we need to implement the monitorHandler function to handle the POST request sent to "/monitor". The main task of this processor function is to store the data sent from the client into the database.
func monitorHandler(c *gin.Context) { //从客户端获取数据 data := c.Request.Body //将数据存储到数据库中 err := saveDataToDatabase(data) if err != nil { log.Println(err) } }
In the above code, we first get the data from the requested Body and then store the data into the database. If the storage fails, we use the log package to print the error message to the console.
3. Real-time monitoring
In order to realize the real-time monitoring function, we need to read data from the database regularly and send alarm information to the administrator when abnormalities or errors are monitored. Goroutine can be used to implement periodic tasks:
func startMonitor() { for { //从数据库读取最新的数据 data, err := readDataFromDatabase() if err != nil { log.Println(err) continue } //检测是否有异常情况 if checkData(data) { //发送报警信息给管理员 err := sendAlertToAdmin() if err != nil { log.Println(err) } } //等待10秒钟再继续检测 time.Sleep(10 * time.Second) } }
In the above code, we define a startMonitor function and use the for loop and the Sleep function of the time package to execute the function regularly. In this function, we first read the latest data from the database and then detect whether there are any abnormalities. If so, we call the sendAlertToAdmin function to send alarm information to the administrator. Finally, we wait 10 seconds before continuing the detection.
4. Send alarm information
The main task of the sendAlertToAdmin function is to send alarm information to the administrator. In order to achieve this function, we can use the SMTP protocol to send emails:
func sendAlertToAdmin() error { //准备邮件内容 msg := []byte("To: admin@example.com " + "Subject: Alert " + "There is an error in the system!") //建立SMTP连接 auth := smtp.PlainAuth("", "user@example.com", "password", "smtp.example.com") err := smtp.SendMail("smtp.example.com:587", auth, "user@example.com", []string{"admin@example.com"}, msg) if err != nil { return err } return nil }
In the above code, we use the smtp package to establish the SMTP connection and send the alarm information to the specified administrator email.
Summary
In this article, we use the coroutine and high-speed routing processing capabilities of the Gin framework and Go language to implement real-time monitoring and alarm functions. We first set up a route and then implemented the handler function to handle POST requests from the client. We then use Goroutine to periodically read data from the database and detect if there are any anomalies. If so, we use the SMTP protocol to send alarm information to the designated administrator's email address. This example shows that the Gin framework is very suitable for quickly developing high-performance web applications, especially in terms of real-time monitoring and alarm functions.
The above is the detailed content of Use the Gin framework to implement real-time monitoring and alarm functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
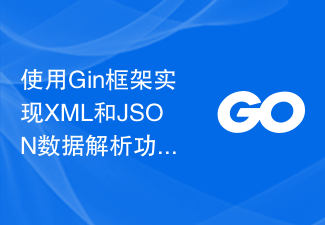
In the field of web development, XML and JSON, one of the data formats, are widely used, and the Gin framework is a lightweight Go language web framework that is simple, easy to use and has efficient performance. This article will introduce how to use the Gin framework to implement XML and JSON data parsing functions. Gin Framework Overview The Gin framework is a web framework based on the Go language, which can be used to build efficient and scalable web applications. The Gin framework is designed to be simple and easy to use. It provides a variety of middleware and plug-ins to make the development
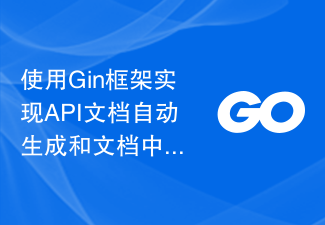
With the continuous development of Internet applications, the use of API interfaces is becoming more and more popular. During the development process, in order to facilitate the use and management of interfaces, the writing and maintenance of API documents has become increasingly important. The traditional way of writing documents requires manual maintenance, which is inefficient and error-prone. In order to solve these problems, many teams have begun to use automatic generation of API documents to improve development efficiency and code quality. In this article, we will introduce how to use the Gin framework to implement automatic generation of API documents and document center functions. Gin is one

With the rapid development of web applications, more and more enterprises tend to use Golang language for development. In Golang development, using the Gin framework is a very popular choice. The Gin framework is a high-performance web framework that uses fasthttp as the HTTP engine and has a lightweight and elegant API design. In this article, we will delve into the application of reverse proxy and request forwarding in the Gin framework. The concept of reverse proxy The concept of reverse proxy is to use the proxy server to make the client
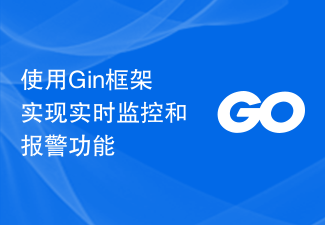
Gin is a lightweight Web framework that uses the coroutine and high-speed routing processing capabilities of the Go language to quickly develop high-performance Web applications. In this article, we will explore how to use the Gin framework to implement real-time monitoring and alarm functions. Monitoring and alarming are an important part of modern software development. In a large system, there may be thousands of processes, hundreds of servers, and millions of users. The amount of data generated by these systems is often staggering, so there is a need for a system that can quickly process this data and provide timely warnings.
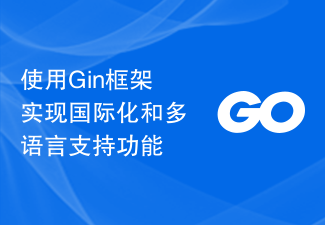
With the development of globalization and the popularity of the Internet, more and more websites and applications have begun to strive to achieve internationalization and multi-language support functions to meet the needs of different groups of people. In order to realize these functions, developers need to use some advanced technologies and frameworks. In this article, we will introduce how to use the Gin framework to implement internationalization and multi-language support capabilities. The Gin framework is a lightweight web framework written in Go language. It is efficient, easy to use and flexible, and has become the preferred framework for many developers. besides,
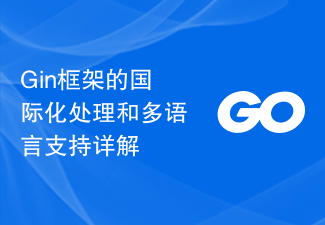
The Gin framework is a lightweight web framework that is characterized by speed and flexibility. For applications that need to support multiple languages, the Gin framework can easily perform internationalization processing and multi-language support. This article will elaborate on the internationalization processing and multi-language support of the Gin framework. Internationalization During the development process, in order to take into account users of different languages, it is necessary to internationalize the application. Simply put, internationalization processing means appropriately modifying and adapting the resource files, codes, texts, etc.
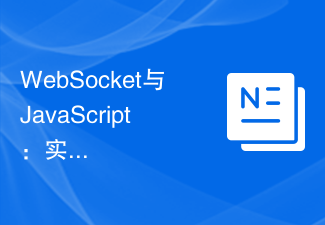
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
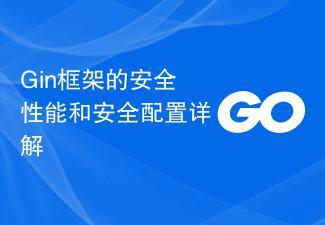
The Gin framework is a lightweight web development framework based on the Go language and provides excellent features such as powerful routing functions, middleware support, and scalability. However, security is a crucial factor for any web application. In this article, we will discuss the security performance and security configuration of the Gin framework to help users ensure the security of their web applications. 1. Security performance of Gin framework 1.1 XSS attack prevention Cross-site scripting (XSS) attack is the most common Web
