


How to use regular expressions to match English sentences in PHP
Regular expression is a very powerful tool in PHP, which can help us quickly match various text patterns. In the fields of English learning and natural language processing, regular expressions can help us match various English sentences. In this article, we'll show you how to use regular expressions to match English sentences in PHP, and provide some practical example code.
First, let us understand the basic structure of English sentences. An English sentence usually consists of a subject, a predicate and an object. For example, "I ate an apple" is a simple English sentence.
In PHP, we use the preg_match function to match regular expressions. This function takes two parameters, the first parameter is the regular expression and the second parameter is the text string to match. When the preg_match function matches a pattern, the return value is 1, otherwise the return value is 0.
Here is a basic example showing how to use a regular expression to match a simple English sentence:
$pattern = "/^([A-Z][a-z]+)s([a-z]+)s([a-z]+)$/"; // 匹配一个简单的英语句子 $string = "I ate an apple"; if (preg_match($pattern, $string)) { echo "匹配成功!"; } else { echo "匹配失败!"; }
The regular expression here^([A-Z][a-z] )s([a-z] )s([a-z] )$
matches a simple English sentence. It contains three sub-patterns, which are used to match the subject, predicate and object of the sentence. We use s
to match spaces.
Next, we introduce some more advanced examples. First, let's look at a regular expression that matches complex English sentences:
$pattern = "/^(([A-Z][a-z]+)+s?)+(was|is|had|hassbeen|havesbeen|willsbe|are|am|wasn't|isn't|haven't|hasn't|won'tsbe|aren't|ain't|hadn't|wouldn'tsbe|won't|weren't)s(([A-Z][a-z]+)+s?)+((is|wass|shassbeens|shavesbeens|sares|swillsbes|swasn'ts|sisn'ts|shaven'ts|shasn'ts|swon'tsbes|saren'ts|sain'ts|shadn'ts|swouldn'tsbes|swon'ts|sweren'ts)+)+((an?s|sthes|s[d]*s)?([A-Z][a-z]+)+s?)+(.|,|?|!)?$/"; // 匹配复杂的英语句子 $string = "She is a beautiful girl, who has been living in Paris for three years."; if (preg_match($pattern, $string)) { echo "匹配成功!"; } else { echo "匹配失败!"; }
The regular expression here/^(( [A-Z][a-z] ) s?) ( was |is|had|hassbeen|havesbeen|willsbe|are|am|wasn't|isn't|haven't|hasn't|won'tsbe|aren't|ain't|hadn't|wouldn'tsbe |won't|weren't )s(( [A-Z][a-z] ) s?) (( is|wass|shassbeens|shavesbeens|sares|swillsbes|swasn'ts|sisn'ts|shaven' ts|shasn'ts|swon'tsbes|saren'ts|sain'ts|shadn'ts|swouldn'tsbes|swon'ts|sweren'ts) ) (( an?s|sthes|s[d]*s )?( [A-Z][a-z] ) s?) (.|,|?|!)?$/
Matches complex English sentences. This regular expression contains multiple subpatterns that match different types of words, punctuation, and spaces. This regex is readable because we split it into multiple lines.
Finally, let’s introduce some other useful regular expressions. Here is some sample code:
Matches English sentences that start with a capital letter and end with a period:
$pattern = "/^[A-Z].*.$/"; $string = "Mary has a little lamb."; if (preg_match($pattern, $string)) { echo "匹配成功!"; } else { echo "匹配失败!"; }
Matches English text that contains dates:
$pattern = "/(0?[1-9]|[12][0-9]|3[01])[-/.]([0]?[1-9]|[1][012])[-/.]d{4}/"; $string = "Today is 2021/12/31"; if (preg_match($pattern, $string)) { echo "匹配成功!"; } else { echo "匹配失败!"; }
Above are some examples Code, I hope it can help you better apply regular expressions to match English sentences. Using regular expressions can help us quickly and accurately identify patterns in English text, thereby facilitating subsequent natural language processing.
The above is the detailed content of How to use regular expressions to match English sentences in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


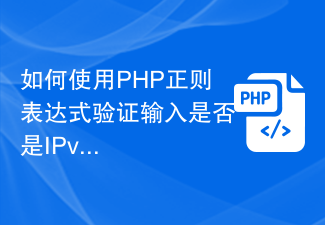
IPv6 refers to InternetProtocolVersion6, which is an IP address protocol used for Internet communication. An IPv6 address is a number composed of 128 bits, usually represented by eight hexadecimal number groups. In PHP, you can use regular expressions to verify whether the input is an IPv6 address. Here's how to use PHP regular expressions to verify IPv6 addresses. Step 1: Understand the format of the IPv6 address. The IPv6 address consists of 8 hexadecimal blocks, each

In PHP, we can use regular expressions to verify whether a string is empty. Cases where the string is empty include the following: The string contains only spaces. The string length is 0. String is null or undefined. Next, we'll cover how to use regular expressions in PHP to validate these situations. Regular expression: s+ This regular expression can be used to match strings containing only spaces. Where s means matching spaces, + means matching one or more. Code example: functionisEmptySt
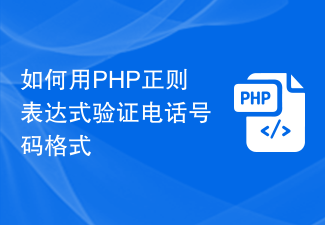
When writing web applications, you often need to verify phone numbers. A common method in PHP is to use regular expressions to determine whether the phone number is in the correct format. Regular expressions are a powerful tool that can help you identify certain patterns in concise statements. Below is an example of using regular expressions in PHP to validate phone number format. First, let's define the common format for phone numbers. Phone numbers can contain numbers, parentheses, hyphens, and spaces. A standard phone number should contain 10 digits, preceded by
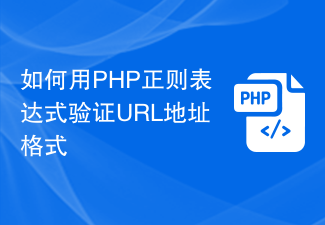
With the rapid development of the Internet, URL addresses have become an indispensable part of people's daily lives. In web development, in order to ensure that the URL address entered by the user can be correctly recognized and used by the system, we need to perform format verification on it. This article will introduce how to use PHP regular expressions to verify URL address format. 1. Basic components of URL addresses Before understanding how to verify the URL address format, we first need to understand the basic components of URL addresses. Usually, a standard URL address consists of
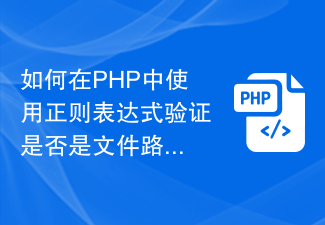
In PHP, regular expressions are a commonly used string matching and validation tool. During the development process, the input file path needs to be frequently verified to ensure that it is in the correct format. This article will introduce how to use regular expressions to verify whether a string is a file path. First, we need to determine the basic format of a file path. In Windows systems, a typical file path is in a format similar to "C:ProgramFilesPHPphp.exe". The path is divided into the following parts:

ID number and passport number are common document numbers in people's lives. When implementing functions involving these document numbers, it is often necessary to perform format verification on the entered numbers to ensure their correctness. In PHP, regular expressions can be used to achieve this function. This article will introduce how to use PHP regular expressions to verify whether the input string is in the format of an ID number or passport number. 1. ID card number verification The ID card number is composed of 18 digits and the last digit may be a letter (check code). Its format is as follows: the first 6
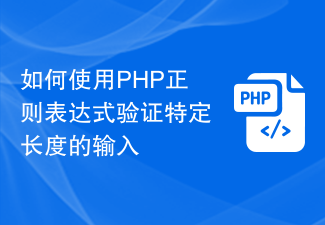
When developing web applications, it is often necessary to verify that user input conforms to specific format and length requirements. PHP regular expressions provide a powerful method for validation. This article will introduce how to use PHP regular expressions to validate input of a specific length. Determine the input length requirement Before you start writing a regular expression, you need to determine the input length requirement. For example, if the user is asked to enter a password of length 8, then the regular expression should match 8 characters instead of matching a string of 8 characters or more. Write regex
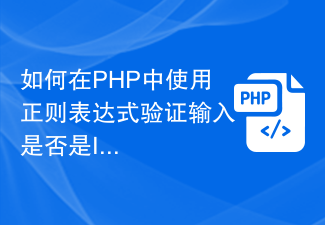
PHP, as a popular server-side programming language, provides some powerful tools to verify the correctness of input data. In this article, we will focus on how to use regular expressions to verify whether the input is an IPv4 address. First, what is an IPv4 address? An IPv4 address refers to a 32-bit binary number, which is usually divided into four 8-bit binary numbers, separated by ".", and expressed in decimal form. For example, 127.0.0.1 is an IPv4 address. Now, let's see how to use regular expressions to
