


PHP Warning: date() expects parameter 2 to be long, string given solution
When developing using PHP programs, you often encounter some warning or error messages. Among them, one error message that may appear is: PHP Warning: date() expects parameter 2 to be long, string given.
This error message means: the second parameter of the function date() is expected to be a long integer (long), but what is actually passed to it is a string (string). So, how should we solve this problem? Below, we will introduce several possible solutions.
1. Confirm whether the passed parameter type is correct
When using the date() function, the second parameter is usually used to represent the timestamp. A timestamp is a way of representing time in integer form, usually obtained using the time() function. Therefore, we first need to confirm whether the second parameter is indeed a long integer timestamp when calling the date() function.
For example, in the following code example, the second parameter of the date() function is a string, so the above error message will appear.
$dateStr = "2022-01-01"; echo date("Y年m月d日",$dateStr); //输出:PHP Warning: date() expects parameter 2 to be long, string given
If you need to convert the time in string form into a timestamp, you can use the strtotime() function to achieve this. For example:
$dateStr = "2022-01-01"; $date = strtotime($dateStr); echo date("Y年m月d日",$date); //输出:2022年01月01日
2. Check whether the parameter is NULL
If the second parameter is NULL when calling the date() function, the above error will occur. Therefore, when using the date() function, you should check whether the second parameter is NULL, for example:
$date = null; echo date("Y年m月d日",$date); //输出:PHP Warning: date() expects parameter 2 to be long, string given
You can change the above code to:
$date = time(); echo date("Y年m月d日",$date); //输出:当前时间的年月日格式
3. Check whether the parameter is Numerical string
When using the date() function, if the second parameter is a string of integer type, the above error message will also appear. Therefore, when using the date() function, you should convert the parameters to numeric types, such as:
$dateStr = "1640995200"; $date = intval($dateStr); echo date("Y年m月d日",$date); //输出:2022年01月01日
Or, directly use the type conversion operator for conversion, such as:
$dateStr = "1640995200"; $date = (int)$dateStr; echo date("Y年m月d日",$date); //输出:2022年01月01日
In summary As mentioned above, when the error message PHP Warning: date() expects parameter 2 to be long, string given appears, we can solve this problem by checking the passed parameter type, determining whether the parameter is NULL, or performing type conversion. When this error occurs, don't panic, just choose the appropriate solution according to the specific situation.
The above is the detailed content of PHP Warning: date() expects parameter 2 to be long, string given solution. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


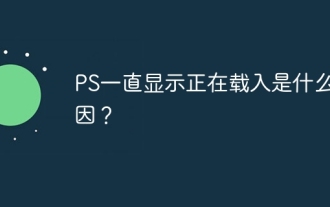
PS "Loading" problems are caused by resource access or processing problems: hard disk reading speed is slow or bad: Use CrystalDiskInfo to check the hard disk health and replace the problematic hard disk. Insufficient memory: Upgrade memory to meet PS's needs for high-resolution images and complex layer processing. Graphics card drivers are outdated or corrupted: Update the drivers to optimize communication between the PS and the graphics card. File paths are too long or file names have special characters: use short paths and avoid special characters. PS's own problem: Reinstall or repair the PS installer.

In PHP, you can effectively prevent CSRF attacks by using unpredictable tokens. Specific methods include: 1. Generate and embed CSRF tokens in the form; 2. Verify the validity of the token when processing the request.

In PHP, the final keyword is used to prevent classes from being inherited and methods being overwritten. 1) When marking the class as final, the class cannot be inherited. 2) When marking the method as final, the method cannot be rewritten by the subclass. Using final keywords ensures the stability and security of your code.
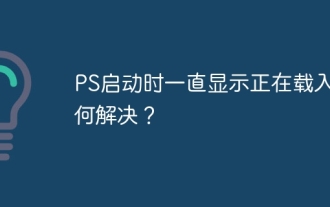
A PS stuck on "Loading" when booting can be caused by various reasons: Disable corrupt or conflicting plugins. Delete or rename a corrupted configuration file. Close unnecessary programs or upgrade memory to avoid insufficient memory. Upgrade to a solid-state drive to speed up hard drive reading. Reinstalling PS to repair corrupt system files or installation package issues. View error information during the startup process of error log analysis.
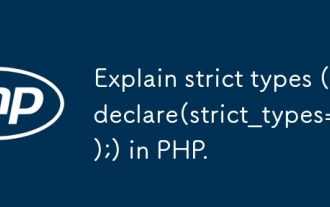
Strict types in PHP are enabled by adding declare(strict_types=1); at the top of the file. 1) It forces type checking of function parameters and return values to prevent implicit type conversion. 2) Using strict types can improve the reliability and predictability of the code, reduce bugs, and improve maintainability and readability.
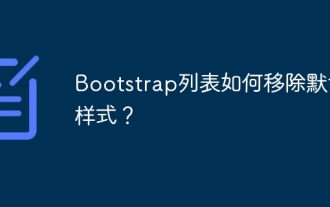
The default style of the Bootstrap list can be removed with CSS override. Use more specific CSS rules and selectors, follow the "proximity principle" and "weight principle", overriding the Bootstrap default style. To avoid style conflicts, more targeted selectors can be used. If the override is unsuccessful, adjust the weight of the custom CSS. At the same time, pay attention to performance optimization, avoid overuse of !important, and write concise and efficient CSS code.
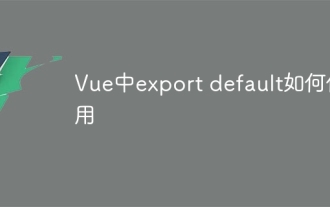
Export default in Vue reveals: Default export, import the entire module at one time, without specifying a name. Components are converted into modules at compile time, and available modules are packaged through the build tool. It can be combined with named exports and export other content, such as constants or functions. Frequently asked questions include circular dependencies, path errors, and build errors, requiring careful examination of the code and import statements. Best practices include code segmentation, readability, and component reuse.
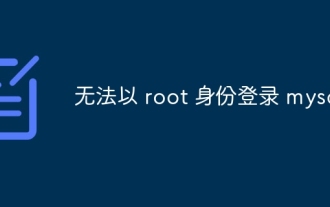
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
