


PHP Regular Expression: How to match all textarea tags in HTML
HTML is a commonly used page markup language used to display content on web pages. In HTML, the textarea tag is used to create text boxes that allow users to enter or edit text.
When you need to extract all textarea tags and their contents from the page, PHP regular expressions can provide a simple and effective solution. In this article, we will learn how to match all textarea tags in HTML using PHP regular expressions.
- Understanding regular expressions
A regular expression is an expression used to match text patterns. In PHP, they are often used for things like searching for strings, replacing strings, or validating input.
Regular expressions consist of various characters, special characters and metacharacters. Among them, special characters include characters used to match specific patterns in text, such as the period (.) used to match any single character. Metacharacters describe how to match a pattern. For example, quantifier metacharacters describe whether to match one or more characters.
- Use PHP DOM parser
In PHP, you can use the DOM parser (Document Object Model) to parse the HTML document and find the required elements in the document element. The DOM parser abstracts HTML into a tree structure (DOM object), allowing programs to easily retrieve and modify element content in web documents.
Using the DOM parser, you can load an HTML document containing a textarea tag using the following code:
$html = file_get_contents('example.html'); $dom = new DOMDocument; $dom->loadHTML($html);
In the code, we first get the HTML file using the file_get_contents()
function content and pass it to the DOM parser. We then use the loadHTML()
method to convert the HTML file into a DOM object.
Next, we can use the getElementsByTagName()
method on the DOM object to get all textarea tags:
$textarea_list = $dom->getElementsByTagName('textarea');
- Matching the content of the textarea tag
Although the DOM parser can easily obtain the textarea tag in the HTML file, it does not provide an easy way to obtain the content of the tag. Therefore, we need to further match the content of the textarea tag using PHP regular expressions.
textarea tags usually take the following form:
<textarea cols="50" rows="10">this is a text area</textarea>
You can use PHP regular expressions to match all textarea tags and their contents. In regular expressions, you can use the preg_match_all()
function to pass an HTML string and related parameters. Here is the JavaScript regular expression that matches all textarea tags:
$pattern = '/<textarea[^>]*>(.*?)</textarea>/si'; preg_match_all($pattern, $html, $matches);
In the code, we use /
to wrap the regular expression and add si## after the expression # represents the search identifier, and adds a greedy checkbox (
*?) to ensure that all textarea tags are matched. If the regular expression matches successfully, all textarea tags and their contents will be returned in the form of an array.
$html = file_get_contents('example.html'); $dom = new DOMDocument; $dom->loadHTML($html); $textarea_list = $dom->getElementsByTagName('textarea'); foreach($textarea_list as $textarea) { $content = $textarea->nodeValue; // 获取 textarea 的内容 echo "textarea content: $content "; }
foreach to loop through each tag and use
$textarea->nodeValue to get the content of the textarea.
- Conclusion
The above is the detailed content of PHP Regular Expression: How to match all textarea tags in HTML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


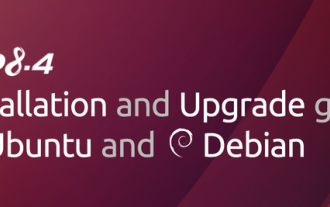
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
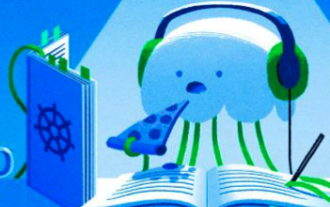
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
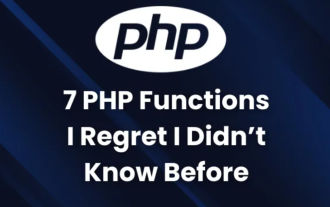
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
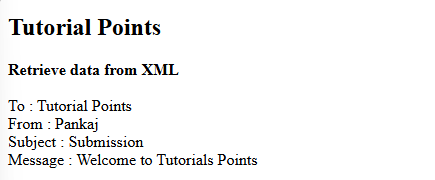
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
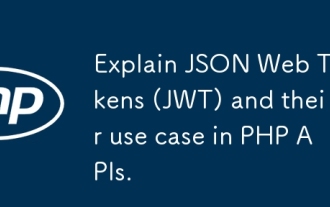
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
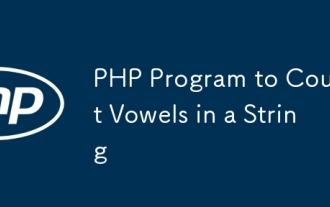
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
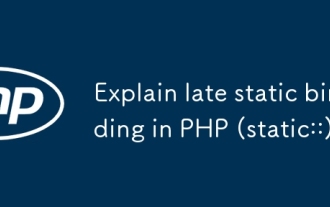
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
