


Use Gin framework to implement distributed deployment and management functions
With the development and application of the Internet, distributed systems have attracted more and more attention and attention. In distributed systems, how to achieve rapid deployment and convenient management has become a necessary technology. This article will introduce how to use the Gin framework to implement the deployment and management functions of distributed systems.
1. Distributed system deployment
The deployment of distributed systems mainly includes code deployment, environment deployment, configuration management and service registration. These aspects will be introduced one by one below.
- Code deployment
In a distributed system, code deployment is an important link. Because in a distributed system, different nodes may need to run different codes, and the running environments may also be different. Therefore, we need to package and compile the code differently and then deploy it on different nodes.
Using the Gin framework, we can easily package and compile code. First, add the following code to the code:
func main() { gin.SetMode(gin.ReleaseMode)// 设置环境 router := gin.Default() // 以下添加路由 router.Run(":8080") // 启动服务 }
Then, use the following command to compile:
CGO_ENABLED=0 GOOS=linux GOARCH=amd64 go build -o main main.go
This completes the compilation of the code. Then, we can transfer the compiled main file to different nodes for deployment.
- Environment deployment
Distributed systems usually need to run on different nodes, so environment deployment is also an essential link. Different nodes may need to run in different environments, so we need to determine the running environment of each node and configure it on the node.
Using the Gin framework, we can easily implement environment deployment. This can be done through containerization technologies such as Docker, which allows for rapid deployment of different environments.
- Configuration Management
In a distributed system, configuration management is also an important link. The configuration of the system may require different configurations on different nodes. Therefore, we need to manage the configuration to facilitate quick configuration updates and management.
In the Gin framework, we can perform configuration management through configuration files. Specifically, viper can be used to implement it, as shown below:
import ( "github.com/spf13/viper" ) // 读取配置文件 viper.SetConfigName("config") // 设置文件名(不带后缀) viper.SetConfigType("yaml") // 设置文件类型 viper.AddConfigPath(".") // 设置文件路径 viper.ReadInConfig() // 读取配置文件
- Service Registration
In a distributed system, service registration is a very important link. Service registration can realize dynamic discovery and management of services, and facilitate service invocation and management.
In the Gin framework, we can use consul and other registration center technologies to implement service registration. Specifically, it can be implemented using consul-api, as shown below:
import ( "github.com/hashicorp/consul/api" ) // 创建一个consul客户端连接 client, err := api.NewClient(&api.Config{Address: "127.0.0.1:8500"}) // 注册服务 registration := &api.AgentServiceRegistration{ Name: "service_name", ID: "service_id", Address: "service_ip", Port: service_port, Tags: []string{"tag1", "tag2"}, Check: &api.AgentServiceCheck{ Interval: "10s", HTTP: "http://127.0.0.1:8080/health", }, } err = client.Agent().ServiceRegister(registration) // 查询服务 services, _, err := client.Health().Service("service_name", "", true, nil)
2. Distributed system management
In distributed systems, management is also a very important aspect. System monitoring, log management, error handling, etc. all need to be managed to facilitate quick location and solution of problems. These aspects will be introduced one by one below.
- System monitoring
In a distributed system, system monitoring is very important. Through monitoring, problems in the system can be quickly discovered and corresponding measures can be taken to deal with them.
Using the Gin framework, we can use prometheus and other monitoring frameworks for monitoring. Specifically, prometheus-promhttp can be used to implement it, as shown below:
import ( "github.com/gin-gonic/gin" "github.com/prometheus/client_golang/prometheus" "github.com/prometheus/client_golang/prometheus/promhttp" ) var ( httpRequestsTotal = prometheus.NewCounterVec(prometheus.CounterOpts{ Name: "http_requests_total", Help: "Total number of HTTP requests", }, []string{"method", "path", "status"}) ) func main() { ... router.Use(prometheusMiddleware()) router.GET("/metrics", promhttp.Handler().ServeHTTP) ... } func prometheusMiddleware() gin.HandlerFunc { return func(c *gin.Context) { start := time.Now() c.Next() httpRequestsTotal.With(prometheus.Labels{ "method": c.Request.Method, "path": c.Request.URL.Path, "status": strconv.Itoa(c.Writer.Status()), }).Inc() prometheusRequestDuration.Observe(float64(time.Since(start).Milliseconds())) } }
- Log management
In distributed systems, log management is also very important. Through logs, you can quickly discover problems in the system and quickly locate and solve problems.
Using the Gin framework, we can use log frameworks such as logrus for log management. Specifically, logrus can be used to implement it, as shown below:
import ( "os" "github.com/sirupsen/logrus" ) func main() { ... // 设置日志输出 jsonFormatter := &logrus.JSONFormatter{} logPath := "./logs/gin.log" logFile, err := os.OpenFile(logPath, os.O_CREATE|os.O_WRONLY|os.O_APPEND, 0666) if err == nil { gin.DefaultWriter = io.MultiWriter(logFile, os.Stdout) gin.DefaultErrorWriter = io.MultiWriter(logFile, os.Stdout) } // 添加钩子 logrus.AddHook(new(LogrusGinHook)) ... } type LogrusGinHook struct{} func (h *LogrusGinHook) Levels() []logrus.Level { return logrus.AllLevels } func (h *LogrusGinHook) Fire(entry *logrus.Entry) error { /** 自定义日志输出内容,例如: access: referer agent */ if entry.Data != nil { if entry.Data["deferred"] != nil { entry.Data["deferred"] = fmt.Sprintf("%+v", entry.Data["deferred"]) } } return nil }
- Error handling
In a distributed system, error handling is also very important. Through error handling, we can quickly solve problems and improve system stability.
Using the Gin framework, we can implement error handling through recover(), as shown below:
func main() { ... router.Use(recoveryMiddleware()) ... } func recoveryMiddleware() gin.HandlerFunc { return func(c *gin.Context) { defer func() { if r := recover(); r != nil { logrus.Errorf("recover error:%v", r) } }() c.Next() } }
3. Summary
By using the Gin framework, we can It is very convenient to implement the deployment and management functions of distributed systems. In practical applications, it can also be combined with other technologies to achieve more powerful functions, such as using grpc to implement distributed system calls, and combining containerization technologies such as k8s to achieve automated deployment and management of the system.
The above is the detailed content of Use Gin framework to implement distributed deployment and management functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


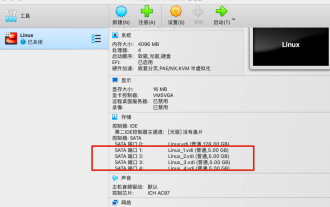
Since I have recently started to be responsible for the construction and stability operation and maintenance of object storage-related systems, as a novice in "object storage", I need to strengthen my learning in this area. Since the company currently uses MinIO to build the company's object storage system, I will gradually share my learning experience about MinIO in the future. Everyone is welcome to continue to pay attention. This article mainly introduces how to set up MinIO in a test environment, which is also the most basic step in building a MinIO learning environment. 1. Prepare the experimental environment using OracleVMVirtualBox virtual machine, install a minimum version of Linux, and then add 4 virtual disks to serve as MinIO virtual disks. The experimental environment is as follows: Next, let me briefly introduce it to you
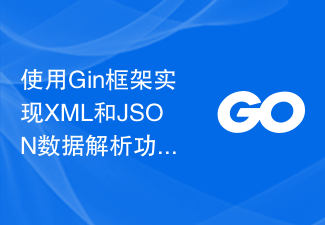
In the field of web development, XML and JSON, one of the data formats, are widely used, and the Gin framework is a lightweight Go language web framework that is simple, easy to use and has efficient performance. This article will introduce how to use the Gin framework to implement XML and JSON data parsing functions. Gin Framework Overview The Gin framework is a web framework based on the Go language, which can be used to build efficient and scalable web applications. The Gin framework is designed to be simple and easy to use. It provides a variety of middleware and plug-ins to make the development
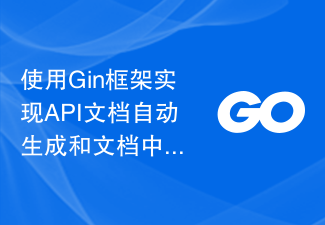
With the continuous development of Internet applications, the use of API interfaces is becoming more and more popular. During the development process, in order to facilitate the use and management of interfaces, the writing and maintenance of API documents has become increasingly important. The traditional way of writing documents requires manual maintenance, which is inefficient and error-prone. In order to solve these problems, many teams have begun to use automatic generation of API documents to improve development efficiency and code quality. In this article, we will introduce how to use the Gin framework to implement automatic generation of API documents and document center functions. Gin is one
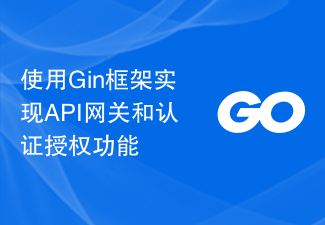
In the modern Internet architecture, API gateway has become an important component and is widely used in enterprise and cloud computing scenarios. The main function of the API gateway is to uniformly manage and distribute the API interfaces of multiple microservice systems, provide access control and security protection, and can also perform API document management, monitoring and logging. In order to better ensure the security and scalability of the API gateway, some access control and authentication and authorization mechanisms have also been added to the API gateway. Such a mechanism can ensure that users and services
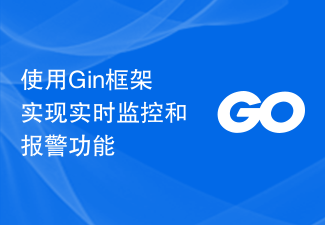
Gin is a lightweight Web framework that uses the coroutine and high-speed routing processing capabilities of the Go language to quickly develop high-performance Web applications. In this article, we will explore how to use the Gin framework to implement real-time monitoring and alarm functions. Monitoring and alarming are an important part of modern software development. In a large system, there may be thousands of processes, hundreds of servers, and millions of users. The amount of data generated by these systems is often staggering, so there is a need for a system that can quickly process this data and provide timely warnings.

With the rapid development of web applications, more and more enterprises tend to use Golang language for development. In Golang development, using the Gin framework is a very popular choice. The Gin framework is a high-performance web framework that uses fasthttp as the HTTP engine and has a lightweight and elegant API design. In this article, we will delve into the application of reverse proxy and request forwarding in the Gin framework. The concept of reverse proxy The concept of reverse proxy is to use the proxy server to make the client
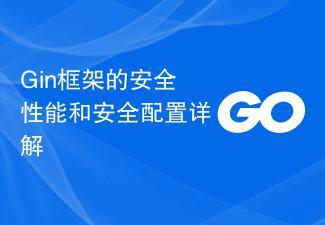
The Gin framework is a lightweight web development framework based on the Go language and provides excellent features such as powerful routing functions, middleware support, and scalability. However, security is a crucial factor for any web application. In this article, we will discuss the security performance and security configuration of the Gin framework to help users ensure the security of their web applications. 1. Security performance of Gin framework 1.1 XSS attack prevention Cross-site scripting (XSS) attack is the most common Web
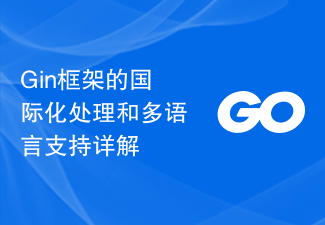
The Gin framework is a lightweight web framework that is characterized by speed and flexibility. For applications that need to support multiple languages, the Gin framework can easily perform internationalization processing and multi-language support. This article will elaborate on the internationalization processing and multi-language support of the Gin framework. Internationalization During the development process, in order to take into account users of different languages, it is necessary to internationalize the application. Simply put, internationalization processing means appropriately modifying and adapting the resource files, codes, texts, etc.
