


Achieve multi-language support and international applications through Spring Boot
With the development of globalization, more and more websites and applications need to provide multi-language support and internationalization functions. For developers, implementing these functions is not an easy task because it requires consideration of many aspects, such as language translation, date, time and currency formats, etc. However, using the Spring Boot framework, we can easily implement multi-language support and international applications.
First, let us understand the LocaleResolver interface provided by Spring Boot. The LocaleResolver interface is an interface used to parse and return Locale objects. The Locale object represents the language, region and related information used by the user. In Spring Boot, the LocaleResolver interface has two implementations: CookieLocaleResolver and AcceptHeaderLocaleResolver.
CookieLocaleResolver parses Locale objects through browser cookies. When the user selects a language, the Locale object will be set to the browser and saved in a cookie. Therefore, the next time the user visits the website, CookieLocaleResolver is able to read the Locale object from the cookie.
AcceptHeaderLocaleResolver parses the Locale object through the Accept-Language field in the HTTP request header. When the user sends a request with the Accept-Language header, AcceptHeaderLocaleResolver will parse out the Locale object and return it.
In addition to the LocaleResolver interface, Spring Boot also provides the MessageSource interface. The MessageSource interface is used to parse and process multi-language messages. In Spring Boot, the MessageSource interface has two implementations: ResourceBundleMessageSource and ReloadableResourceBundleMessageSource.
ResourceBundleMessageSource is a simple implementation that parses multilingual messages from a provided properties file. The properties file contains key-value pairs, where the key is the message identifier and the value is the message itself. For example, for English and Chinese messages, the properties file may look like this:
# messages_en_US.properties hello=Hello world! # messages_zh_CN.properties hello=你好,世界!
When we need to display a message to the user, we can use the MessageSource interface to obtain the corresponding message, and then display it to the user .
ReloadableResourceBundleMessageSource is very similar to ResourceBundleMessageSource, but it allows us to reload the properties file while the application is running. This is useful for applications that require frequently updated messages.
When implementing multi-language support and international applications, we usually need to consider date, time and currency format issues. Spring Boot provides a FormattingConversionServiceFactoryBean class to solve these problems. We can use it to define date, time, and currency formats and make them valid in multilingual applications.
The following is an example of using the FormattingConversionServiceFactoryBean class to define date, time and currency formats:
@Configuration public class AppConfig { @Bean public FormattingConversionServiceFactoryBean formattingConversionServiceFactoryBean() { FormattingConversionServiceFactoryBean factoryBean = new FormattingConversionServiceFactoryBean(); factoryBean.setRegisterDefaultFormatters(false); factoryBean.setFormatters(Set.of(dateTimeFormatter(), dateFormatter(), numberFormatter())); return factoryBean; } private DateTimeFormatter dateTimeFormatter() { return DateTimeFormatter.ofLocalizedDateTime(FormatStyle.MEDIUM).withLocale(LocaleContextHolder.getLocale()); } private DateTimeFormatter dateFormatter() { return DateTimeFormatter.ofLocalizedDate(FormatStyle.MEDIUM).withLocale(LocaleContextHolder.getLocale()); } private NumberFormatter numberFormatter() { return new NumberFormatter(NumberFormat.getCurrencyInstance(LocaleContextHolder.getLocale())); } }
In the above code, we create a FormattingConversionServiceFactoryBean to define date, time and currency formats. We use DateTimeFormatter and NumberFormatter to define date time and currency formats, and use the LocaleContextHolder.getLocale() method to obtain the current user's Locale object.
Finally, we need to set up the LocaleResolver and MessageSource in our application to support multiple languages. We can achieve this using the @EnableWebMvc and @Configuration annotations provided by Spring Boot. The following is a sample configuration class:
@Configuration @EnableWebMvc public class WebConfig implements WebMvcConfigurer { @Bean public LocaleResolver localeResolver() { AcceptHeaderLocaleResolver resolver = new AcceptHeaderLocaleResolver(); resolver.setDefaultLocale(Locale.US); return resolver; } @Bean public MessageSource messageSource() { ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource(); messageSource.setBasenames("messages"); messageSource.setFallbackToSystemLocale(false); return messageSource; } @Override public void addInterceptors(InterceptorRegistry registry) { LocaleChangeInterceptor localeChangeInterceptor = new LocaleChangeInterceptor(); localeChangeInterceptor.setParamName("lang"); registry.addInterceptor(localeChangeInterceptor); } }
In the above code, we create a WebConfig configuration class and enable the Spring MVC feature using the @EnableWebMvc annotation. We use localeResolver() Bean and messageSource() Bean to set LocaleResolver and MessageSource. We also added a LocaleChangeInterceptor using the addInterceptors() method, and in this interceptor we used the "lang" parameter to switch the user's language.
To sum up, we can easily implement multi-language support and international applications through the LocaleResolver interface, MessageSource interface and FormattingConversionServiceFactoryBean class provided by Spring Boot. Using the above methods, we can more easily and conveniently build a global application that meets the different language, culture, and regional needs of users.
The above is the detailed content of Achieve multi-language support and international applications through Spring Boot. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
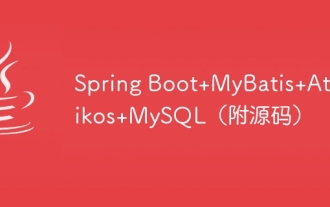
In actual projects, we try to avoid distributed transactions. However, sometimes it is really necessary to do some service splitting, which will lead to distributed transaction problems. At the same time, distributed transactions are also asked in the market during interviews. You can practice with this case, and you can talk about 123 in the interview.
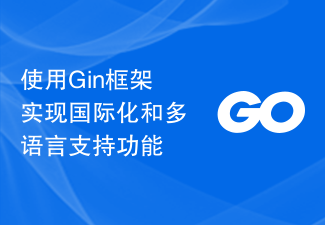
With the development of globalization and the popularity of the Internet, more and more websites and applications have begun to strive to achieve internationalization and multi-language support functions to meet the needs of different groups of people. In order to realize these functions, developers need to use some advanced technologies and frameworks. In this article, we will introduce how to use the Gin framework to implement internationalization and multi-language support capabilities. The Gin framework is a lightweight web framework written in Go language. It is efficient, easy to use and flexible, and has become the preferred framework for many developers. besides,
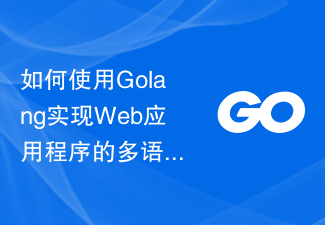
With the continuous advancement of globalization, the need for multi-language is becoming more and more common, and multi-language support for web applications has become an issue that developers need to consider. Golang, as an efficient and easy-to-use programming language, can also solve this problem well. In this article, we will discuss how to implement multi-language support for web applications using Golang. 1. Basic principles of multi-language support The key to multi-language support for web applications lies in how to identify and store text in different languages. A common approach is to use

ThinkPHP6 multi-language switching: realizing international applications With the rapid development of the Internet and the process of globalization, more and more websites and applications need to support multi-language functions to meet the needs of users in different countries and regions. When using ThinkPHP6 to develop web applications, achieving multi-language switching is an important task. This article will introduce how to implement international applications in ThinkPHP6 to provide users with a convenient multi-language experience. Why do you need multi-language switching? In the context of globalization, users use the Internet
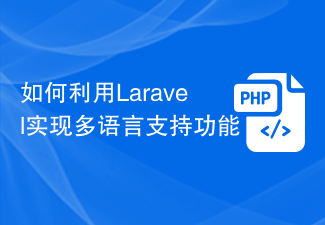
Laravel is a very popular PHP framework that provides a large number of features and libraries that make web application development easier and more efficient. One of the important features is multi-language support. Laravel achieves multi-language support through its own language package mechanism and third-party libraries. This article will introduce how to use Laravel to implement multi-language support and provide specific code examples. Using Laravel's language pack function Laravel comes with a language pack mechanism that allows us to easily implement multilingualism
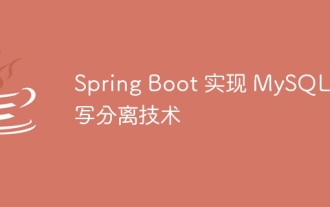
How to achieve read-write separation, Spring Boot project, the database is MySQL, and the persistence layer uses MyBatis.
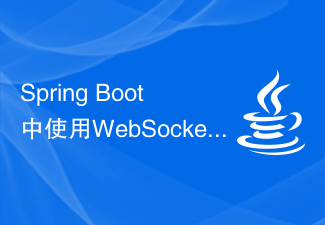
In modern web application development, WebSocket is a common technology for instant communication and real-time data transfer. The SpringBoot framework provides support for integrated WebSocket, making it very convenient for developers to implement push and notification functions. This article will introduce how to use WebSocket to implement push and notification functions in SpringBoot, and demonstrate the implementation of a simple real-time online chat room. Create a SpringBoot project First, we need to create a
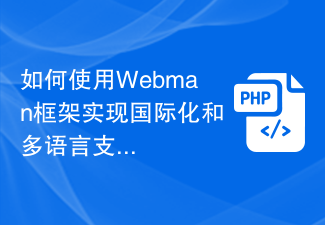
Nowadays, with the continuous development of Internet technology, more and more websites and applications need to support multi-language and internationalization. In web development, using frameworks can greatly simplify the development process. This article will introduce how to use the Webman framework to achieve internationalization and multi-language support, and provide some code examples. 1. What is the Webman framework? Webman is a lightweight PHP-based framework that provides rich functionality and easy-to-use tools for developing web applications. One of them is internationalization and multi-
