


Implement distributed processing of large-scale tasks through go-zero
With the continuous development of the Internet, we are facing more and more data processing problems. Therefore, distributed systems have become a necessary means to solve these problems. In distributed systems, the processing of large-scale tasks is a key issue. In this article, we will explore how to use go-zero to implement distributed processing of large-scale tasks.
Go-zero is an open source, golang-based microservice framework. It features high availability, performance and scalability. It provides many components, such as RPC, cache, log, config, etc. Among these components, we will focus on the distributed task processing component in go-zero - job.
The job component is a distributed task queue in go-zero. It provides producer and consumer models that can help us build large-scale distributed task processing systems. In this system, users can add tasks to the queue and then wait for the consumer to execute them.
In go-zero, implementing large-scale task processing through the job component requires us to follow the following steps:
Step 1: Create a task queue
First, we need Create a task queue. This can be done by calling the job.NewQueue function. When creating a task queue, we need to specify the name of the queue and the number of consumers.
For example, we can create a task queue named "TaskQueue" with a number of consumers:
import "github.com/tal-tech/go-zero/core/jobs" queue := jobs.NewQueue("TaskQueue", 5)
The queue name needs to be unique, because in subsequent operations, we need to use Queue name to add tasks and start consumers.
Step 2: Define the task processing method
Before task processing, we need to define the task processing method. This method will be called when the task in the queue is consumed. In go-zero, we can define a task processor and register it into the task queue using the job.RegisterJobFunc function.
For example, we can define a task processor named "TaskHandler":
import "github.com/tal-tech/go-zero/core/jobs" func TaskHandler(payload interface{}) { // 处理任务 } jobs.RegisterJobFunc("TaskHandler", TaskHandler)
In this processor function, we can perform any required operations based on the load of the task.
Step 3: Add tasks to the queue
Once the queue and processor are defined, we can add the task to the queue. In go-zero, we can use the job.Enqueue function to achieve this.
For example, we can add a task with a load of {"task_id": 1001, "data": "hello world"} to a queue named "TaskQueue":
import "github.com/tal-tech/go-zero/core/jobs" queue.Enqueue("TaskQueue", "TaskHandler", `{"task_id":1001,"data":"hello world"}`)
When calling the Enqueue function, we need to specify the queue name, task processor name and task load.
Step 4: Start the consumer
Finally, we need to start the consumer to process the task. In go-zero, we can use the job.Worker function to start the consumer. For example, we can start 5 consumers to process the task queue named "TaskQueue":
import "github.com/tal-tech/go-zero/core/jobs" job.NewWorker("TaskQueue", jobs.HandlerFuncMap{ "TaskHandler": TaskHandler, }, 5).Start()
The first parameter is the queue name, and the second parameter is the processor name and the processor function. The third parameter is the number of consumers.
When the consumer starts, it will immediately start to obtain tasks from the queue and execute the task processor function. If there are no tasks in the queue, the consumer will wait until there is a task.
Through the above four steps, we can implement a distributed system in go-zero that can handle large-scale tasks. The system can be scaled horizontally and has high availability and performance.
Summary
In terms of large-scale task processing, distributed systems have become a necessary means. go-zero provides job components to help us build distributed task processing systems. Using this component, we can easily create task queues, define task processors, add tasks, start consumers, and more. I hope this article can help you better understand how to implement distributed processing of large-scale tasks in go-zero.
The above is the detailed content of Implement distributed processing of large-scale tasks through go-zero. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
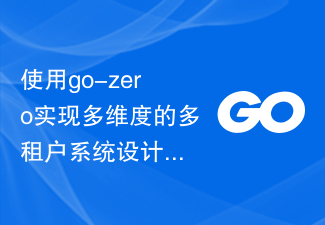
With the development of the Internet, more and more enterprises are beginning to transform towards multi-tenancy to improve their competitiveness. Multi-tenant systems allow multiple tenants to share the same set of applications and infrastructure, each with their own data and privacy protection. In order to implement a multi-tenant system, multi-dimensional design needs to be considered, involving issues such as data isolation and security. This article will introduce how to use the go-zero framework to implement multi-dimensional multi-tenant system design. go-zero is a microservice framework based on gRPC, which is high-performance, efficient and easy to expand.
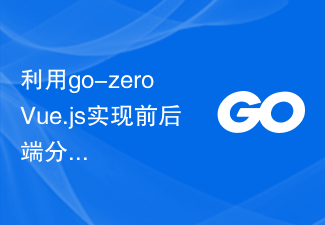
In today's rapidly developing Internet era, front-end and back-end separated API service design has become a very popular design idea. Using this design idea, we can develop front-end code and back-end code separately, thereby achieving more efficient development and better system maintainability. This article will introduce how to implement front-end and back-end separated API service design by using go-zero and Vue.js. 1. Advantages of front-end and back-end separated API service design The advantages of front-end and front-end separated API service design mainly include the following aspects: Development
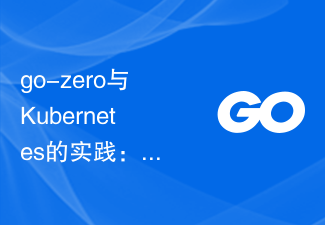
As the scale of the Internet continues to expand and user needs continue to increase, the advantages of microservice architecture are receiving more and more attention. Subsequently, the containerized microservice architecture has become particularly important, which can better meet the needs of high availability, high performance, high scalability and other aspects. Under this trend, go-zero and Kubernetes have become the most popular containerized microservice frameworks. This article will introduce how to use the go-zero framework and Kubernetes container orchestration tools to build high-availability, high-performance
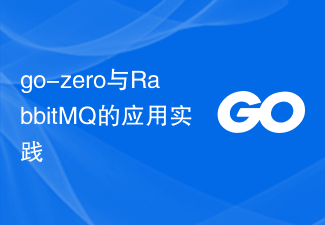
Now more and more companies are beginning to adopt the microservice architecture model, and in this architecture, message queues have become an important communication method, among which RabbitMQ is widely used. In the Go language, go-zero is a framework that has emerged in recent years. It provides many practical tools and methods to allow developers to use message queues more easily. Below we will introduce go-zero based on practical applications. And the usage and application practice of RabbitMQ. 1.RabbitMQ OverviewRabbit
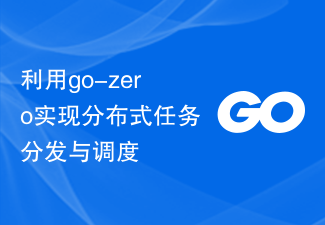
With the rapid development of Internet business and the gradually increasing business volume, the amount of data that a single server can process is far from meeting demand. In order to meet the requirements of high concurrency, high availability, and high performance, distributed architecture emerged as the times require. In a distributed architecture, task distribution and scheduling is a very critical component. The quality of task distribution and scheduling will directly affect the performance and stability of the entire system. Here, we will introduce how to use the go-zero framework to implement distributed task distribution and scheduling. 1. Distributed task distributionTask distribution
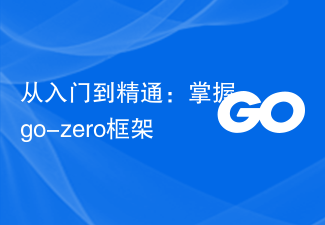
Go-zero is an excellent Go language framework that provides a complete set of solutions, including RPC, caching, scheduled tasks and other functions. In fact, it is very simple to build a high-performance service using go-zero, and you can even go from beginner to proficient in a few hours. This article aims to introduce the process of building high-performance services using the go-zero framework and help readers quickly grasp the core concepts of the framework. 1. Installation and configuration Before starting to use go-zero, we need to install it and configure some necessary environments. 1

With the popularity of microservice architecture, communication between microservices becomes more and more important. The REST API communication method commonly used in the past has the following shortcomings when microservices call each other: frequent network requests will cause delays and performance bottlenecks; for high-frequency requests, a large number of requests in a short period of time may cause service failure. Crash; For scenarios with a large amount of data transmission, the transmission method based on the HTTP protocol is also prone to inefficiency. Therefore, based on message queue (MessageQueue), the implementation of microservices
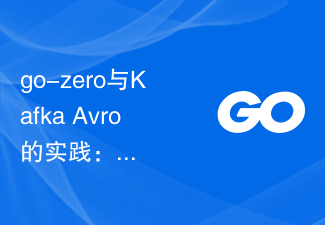
In recent years, with the rise of big data and active open source communities, more and more enterprises have begun to look for high-performance interactive data processing systems to meet the growing data needs. In this wave of technology upgrades, go-zero and Kafka+Avro are being paid attention to and adopted by more and more enterprises. go-zero is a microservice framework developed based on the Golang language. It has the characteristics of high performance, ease of use, easy expansion, and easy maintenance. It is designed to help enterprises quickly build efficient microservice application systems. its rapid growth
