


Django Programming Practice: A Complete Tutorial on Building Efficient and Scalable Web Applications
Django is a popular web framework that is favored by developers because it is easy to learn, efficient and scalable. This article will provide a complete practical guide to Django programming to help you build efficient and scalable web applications.
- Basic knowledge of Django
Django is a Web framework based on the MVC architecture, which uses Python as the programming language. Before you start, you need to learn the following basic concepts:
- Model: It represents an entity in the data, such as users, articles, etc.
- View (View): It is a direct or indirect representation of the model, which can read and modify data in the model.
- Template: It is the HTML code used to render the view.
- Create Django Project
To create a Django project, you can run the following command in the terminal:
django-admin startproject myproject
This will create a Django project named The Django project for "myproject". In this project, you create applications to manage domain-specific logic.
- Creating a Django application
To create a Django application, you can run the following command in the terminal:
python manage.py startapp myapp
This will create a file named Django application for "myapp". In the application, you can define models, views, and templates.
- Define the model
In your Django application, you define a data model by defining a model class. For example, to define a model class named User, you can write the following code:
from django.db import models class User(models.Model): name = models.CharField(max_length=100) email = models.EmailField(unique=True) password = models.CharField(max_length=100)
This model class will create a database table named "User", which includes three fields: name, email, and password. The types of these fields can be changed according to your needs.
- Define views
Views are closely related to models, and they are responsible for presenting data related to the model. To define a view function, write the following code:
from django.shortcuts import render from django.http import HttpResponse from .models import User def user_list(request): users = User.objects.all() context = { 'users': users, } return render(request, 'user_list.html', context)
In this view function, we get all the user data and render them along with the template.
- Define template
Template is responsible for rendering views and rendering HTML content. You can use the Django template language to write template code. For example, here is a simple user list template:
<ul> {% for user in users %} <li>{{ user.name }}</li> {% endfor %} </ul>
This template will render the names of all users.
- Configuring URL routing
In Django, URL routing maps requests to the appropriate view function. You define URL routes in your project's urls.py file. For example, the following is a simple URL route:
from django.urls import path from .views import user_list urlpatterns = [ path('users/', user_list, name='user_list'), ]
This route will map the request to the user_list view function.
- Running Django Application
After writing your Django application, you can run the following command in the terminal:
python manage.py runserver
This will Start Django's development server and run your application. You can view your user list by visiting http://127.0.0.1:8000/users/ in your browser.
- Deploy Django Application
To deploy your Django application into a production environment, you need to complete the following process:
- Push the code to a code hosting service such as GitHub.
- Set up the database using the database type required for the production environment (e.g. MySQL).
- Package source code and dependencies into a single file.
- Deploy static files (such as CSS and JS files) to the web server.
- Deploy the web server and application server and configure them to serve your application.
Summary
Django is a powerful web framework that provides many useful tools and libraries to help you build efficient and scalable web applications. With this tutorial, you can learn the basics of Django and how to create Django projects, applications, models, views, and templates. Additionally, we've covered relevant information about deploying Django applications. I hope this article helps you start writing high-quality, efficient web applications.
The above is the detailed content of Django Programming Practice: A Complete Tutorial on Building Efficient and Scalable Web Applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



This article details methods to resolve event ID10000, which indicates that the Wireless LAN expansion module cannot start. This error may appear in the event log of Windows 11/10 PC. The WLAN extensibility module is a component of Windows that allows independent hardware vendors (IHVs) and independent software vendors (ISVs) to provide users with customized wireless network features and functionality. It extends the capabilities of native Windows network components by adding Windows default functionality. The WLAN extensibility module is started as part of initialization when the operating system loads network components. If the Wireless LAN Expansion Module encounters a problem and cannot start, you may see an error message in the event viewer log.
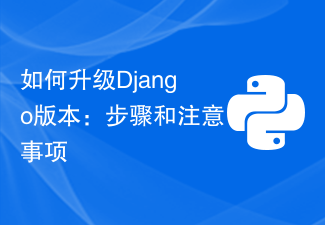
How to upgrade Django version: steps and considerations, specific code examples required Introduction: Django is a powerful Python Web framework that is continuously updated and upgraded to provide better performance and more features. However, for developers using older versions of Django, upgrading Django may face some challenges. This article will introduce the steps and precautions on how to upgrade the Django version, and provide specific code examples. 1. Back up project files before upgrading Djan
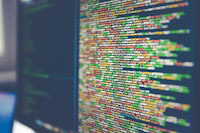
Using Prepared Statements Prepared statements in PDO allow the database to precompile queries and execute them multiple times without recompiling. This is essential to prevent SQL injection attacks, and it can also improve query performance by reducing compilation overhead on the database server. To use prepared statements, follow these steps: $stmt=$pdo->prepare("SELECT*FROMusersWHEREid=?");Bind ParametersBind parameters are a safe and efficient way to provide query parameters that can Prevent SQL injection attacks and improve performance. By binding parameters to placeholders, the database can optimize query execution plans and avoid performing string concatenation. To bind parameters, use the following syntax:
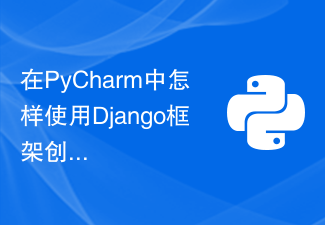
Tips on how to create projects using the Django framework in PyCharm, requiring specific code examples. Django is a powerful Python Web framework that provides a series of tools and functions for quickly developing Web applications. PyCharm is an integrated development environment (IDE) developed in Python, which provides a series of convenient functions and tools to increase development efficiency. Combining Django and PyCharm makes it faster and more convenient to create projects
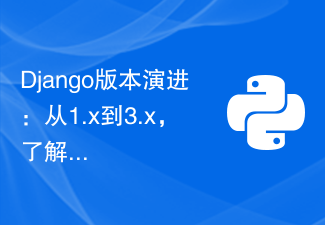
Django is a web framework written in Python. Its main features are fast development, easy expansion, high reusability, etc. Since its first launch in 2005, Django has grown into a powerful web development framework. As time goes by, Django versions are constantly updated. This article will provide an in-depth understanding of Django version evolution, changes from 1.x to 3.x, introduce new features, improvements, and changes that need attention, and provide detailed code examples. Djang
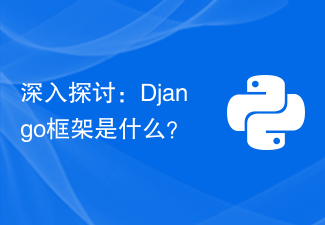
The Django framework is a Python framework for web applications that provides a simple and powerful way to create web applications. In fact, Django has become one of the most popular Python web development frameworks and has become the first choice for many companies, including Instagram and Pinterest. This article will delve into what the Django framework is, including basic concepts and important components, as well as specific code examples. Django basic conceptsDjan
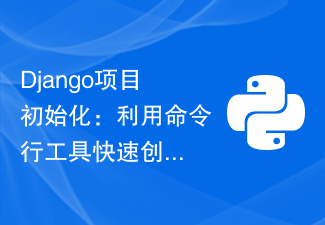
Django project initialization: Use command line tools to quickly create a new project. Django is a powerful Python Web framework. It provides many convenient tools and functions to help developers quickly build Web applications. Before starting a new Django project, we need to go through some simple steps to initialize the project. This article will introduce how to use command line tools to quickly create a new Django project, including specific code examples. First, make sure you have DJ installed
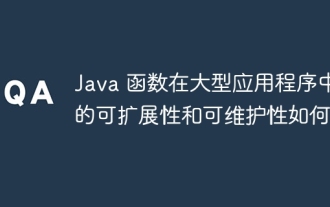
Java functions provide excellent scalability and maintainability in large applications due to the following features: Scalability: statelessness, elastic deployment and easy integration, allowing easy adjustment of capacity and scaling of deployment. Maintainability: Modularity, version control, and complete monitoring and logging simplify maintenance and updates. By using Java functions and serverless architecture, more efficient processing and simplified maintenance can be achieved in large applications.
