


Use regular expressions in golang to verify whether a string is a number
In golang, regular expressions can be used to easily verify whether a string is a number. Let's introduce how to use regular expressions for numerical verification in golang.
First, we need to import the related package of regular expressions, that is, the regexp package. In golang, the regexp package provides a function MatchString, which can be used to verify whether a string conforms to the rules of a certain regular expression.
Next, we need to write a regular expression for numerical verification. In golang, numbers can be integers or floating point numbers, so we need to write two regular expressions to verify integers and floating point numbers respectively.
Regular expression to verify integers:
^[0-9]+$
The meaning of this regular expression is that it starts with the number 0-9, contains one or more numbers 0-9 in the middle, and ends with the number 0 -9 ending. This regular expression can verify whether a string is an integer.
Regular expression to verify floating point numbers:
^[0-9]+(.[0-9]+)?$
The meaning of this regular expression is that it starts with the number 0-9, contains 1 or more numbers 0-9 in the middle, and then may A decimal point "." appears, followed by one or more digits 0-9, and finally ends with the digits 0-9. This regular expression can verify whether a string is a floating point number.
Now, let’s take a look at how to use these two regular expressions for number verification in golang:
package main import ( "fmt" "regexp" ) func isNumber(str string) bool { // 整数的正则表达式 integerPattern := "^[0-9]+$" // 浮点数的正则表达式 floatPattern := "^[0-9]+(\.[0-9]+)?$" match, _ := regexp.MatchString(integerPattern, str) if match { return true } match, _ = regexp.MatchString(floatPattern, str) if match { return true } return false } func main() { fmt.Println(isNumber("123")) // true fmt.Println(isNumber("12.3")) // true fmt.Println(isNumber("abc")) // false fmt.Println(isNumber("1a2b3c")) // false }
In the above code, we wrote an isNumber function to perform number verification verify. This function accepts a string as a parameter, and then uses integer regular expressions and floating point regular expressions to verify whether the string is a number. If the verification is successful, it returns true, otherwise it returns false.
Finally, in the main function, we call the isNumber function to verify whether some strings are numbers. As you can see, if the input string is a number, the return result of the function is true, otherwise it is false.
In actual development, digital verification is a very common operation. Using regular expressions makes it easier for us to perform numerical verification. The above is an introduction to using regular expressions to verify whether a string is a number in golang.
The above is the detailed content of Use regular expressions in golang to verify whether a string is a number. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
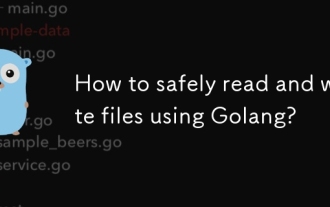
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
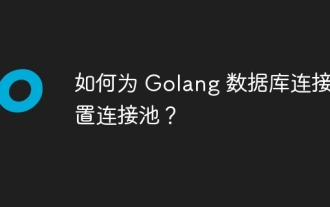
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
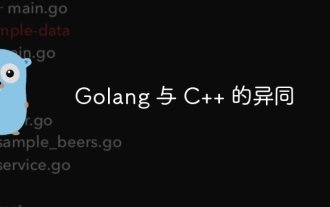
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
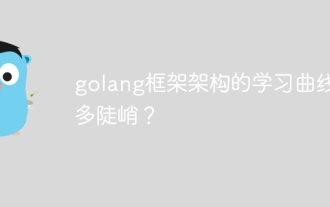
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
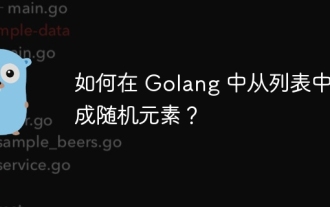
How to generate random elements of a list in Golang: use rand.Intn(len(list)) to generate a random integer within the length range of the list; use the integer as an index to get the corresponding element from the list.
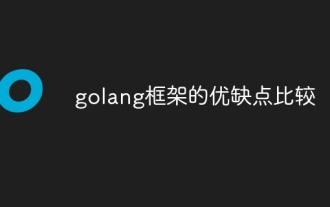
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
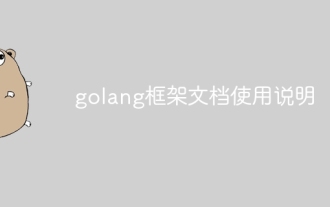
How to use Go framework documentation? Determine the document type: official website, GitHub repository, third-party resource. Understand the documentation structure: getting started, in-depth tutorials, reference manuals. Locate the information as needed: Use the organizational structure or the search function. Understand terms and concepts: Read carefully and understand new terms and concepts. Practical case: Use Beego to create a simple web server. Other Go framework documentation: Gin, Echo, Buffalo, Fiber.
