How to use Golang to implement email sending in web applications
With the popularity of Web applications, email sending has become a necessary function for many applications. As a fast, safe and easy-to-write programming language, Golang is increasingly favored by developers. In this article, we will introduce how to use Golang to implement email sending functionality in web applications.
Step 1: Install the SMTP sending library
In order to implement the email sending function, you first need to install the SMTP sending library. Currently, there are many different SMTP libraries in the Golang community, and these libraries have different features and functions. We have chosen GoMail as our SMTP library here.
Enter the following command on the command line to install GoMail:
go get gopkg.in/mail.v2
This command will automatically download GoMail and install it under your GOPATH.
Step 2: Write the code
After installing GoMail, we can start writing our email sending code. Below is a simple Golang email sending example, which contains the necessary information needed to send an email.
package main import ( "gopkg.in/mail.v2" "log" ) func main() { // smtp服务器配置 smtpHost := "smtp.gmail.com" smtpPort := 587 smtpUserName := "myemail@gmail.com" smtpPassword := "mypassword" // 发件人信息 from := "myemail@gmail.com" to := []string{"receiver@domain.com"} // 邮件内容 subject := "Test Email" body := "This is a test email sent using Golang." // 创建邮件 m := mail.NewMessage() m.SetHeader("From", from) m.SetHeader("To", to...) m.SetHeader("Subject", subject) m.SetBody("text/html", body) // 连接SMTP服务器 d := mail.NewDialer(smtpHost, smtpPort, smtpUserName, smtpPassword) // 发送邮件 if err := d.DialAndSend(m); err != nil { log.Fatalln(err) } }
In this example, we first define the host, port, username and password of the SMTP server we need to connect to. We then specified the sender and recipient information, as well as the subject and content of the email. Next, we use the NewMessage function to create a new email, and use the SetHeader and SetBody methods to set the sender, recipient, subject, and content of the email. Finally, we use the NewDialer function to create a new Dialer and use the DialAndSend method to connect to the SMTP server and send emails.
Step 3: Test the code
After we finish writing the code, we can run the program and test whether our code can successfully send emails. Execute the following command on the command line to start the program:
go run main.go
If there are no errors in your code, you should receive a test email in the recipient's mailbox.
Summary
By using Golang and the SMTP library to implement email sending functionality, we can provide a very important feature to our web application. Of course, this is just an entry-level example. In actual projects, you may need to integrate the email sending function with other functions, or use more advanced functions such as email templates and attachments. But the core idea of the basic email sending functionality is the same. We hope this article helps you get started using Golang to implement email sending functionality in web applications.
The above is the detailed content of How to use Golang to implement email sending in web applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


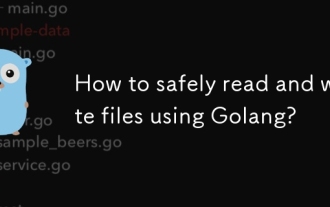
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
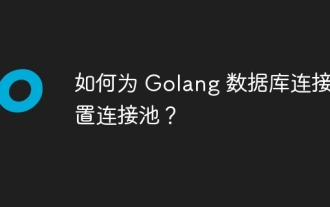
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
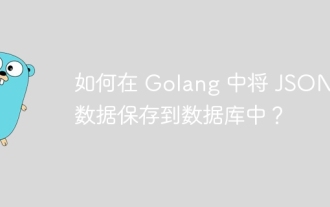
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
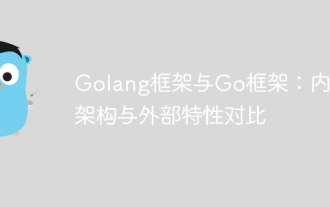
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
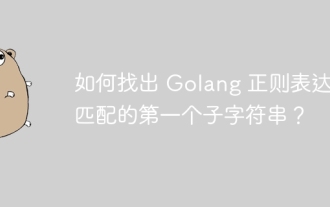
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
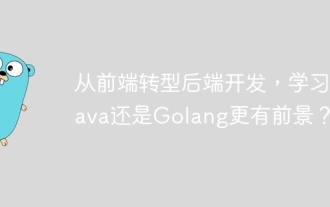
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
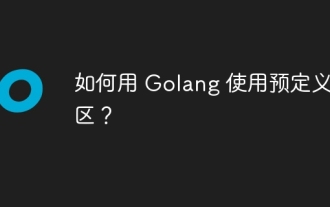
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
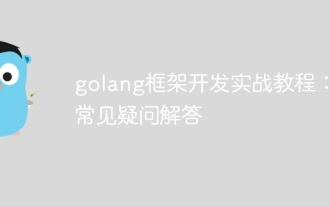
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
