


How to set expiration time in PHP form to strengthen security measures
PHP form is a commonly used interactive method in website development. It can receive user input and pass the data to the server for processing. However, since form data is transmitted over HTTP, there are certain security risks. One of them is that it is easy for malicious attackers to use replay attacks to carry out data tampering or disguise attacks. In order to prevent such attacks, we can set the expiration time in the PHP form and strengthen security measures. This article will introduce how to set the expiration time in PHP forms to strengthen security protection.
- What is the expiration time
The expiration time refers to setting a time limit before the form is submitted. If it is not submitted within the specified time, the form will become invalid. This mechanism prevents forms from being used by malicious attackers to perform data tampering or disguise attacks through replay attacks. At the same time, the expiration time can also limit the validity period of the form to avoid useless data occupying server resources.
- How to set the expiration time
You can use session technology to set the expiration time in PHP. The sample code is as follows:
<?php session_start(); if (isset($_SESSION['LAST_ACTIVITY']) && (time() - $_SESSION['LAST_ACTIVITY'] > 1800)) { session_unset(); session_destroy(); } $_SESSION['LAST_ACTIVITY'] = time(); ?>
The above code means, If the current time minus the time of the last operation is greater than 1800 seconds (30 minutes), the session is destroyed.
- How to use expiration time to enhance form security
After setting the expiration time, we also need to verify the form data when the form is submitted, such as keywords Perform data verification and filtering on each segment to prevent security threats such as SQL injection, XSS attacks, and CSRF attacks. The following are some specific measures:
3.1 Data verification
When receiving form submission data, we can use PHP's filter function to filter, verify and clean the form submission data. , improve data security. The filter function can verify data type, length, case, and special characters. The code example is as follows:
<?php // 过滤输入的字符串 $username = filter_input(INPUT_POST, 'username', FILTER_SANITIZE_STRING); // 验证电子邮箱格式 $email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL); // 校验输入的 URL $url = filter_input(INPUT_POST, 'url', FILTER_VALIDATE_URL); // 验证IP地址 $ip = filter_input(INPUT_POST, 'ip', FILTER_VALIDATE_IP); ?>
3.2 Preventing SQL Injection
Before the form data is stored in the database, we can Prevent SQL injection by using parameter binding, escape characters, etc. Among them, parameter binding can use PDO or mysqli library, and escape characters can use functions such as addslashes. The sample code is as follows:
<?php // 参数绑定防止 SQL 注入 $stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username'); $stmt->bindParam(':username', $username); $stmt->execute(); // 转义字符串防止 SQL 注入 $username = addslashes($_POST['username']); $password = addslashes($_POST['password']); ?>
3.3 Preventing XSS attacks
When outputting form data, we can filter the submitted data to prevent XSS attacks. You can use PHP's htmlspecialchars and other functions to convert special characters into HTML escape characters. The sample code is as follows:
<?php // 输出转义后的字符串 echo htmlspecialchars($input, ENT_QUOTES, 'UTF-8'); ?>
3.4 Preventing CSRF attacks
In order to prevent CSRF attacks, we can add a csrf_token, used to verify whether the source of the form is legal. You can use PHP session to store tokens. The sample code is as follows:
<?php if ($_SERVER['REQUEST_METHOD'] === 'POST') { $csrf_token = $_POST['csrf_token']; if (!isset($_SESSION['csrf_token']) || $_SESSION['csrf_token'] !== $csrf_token) { die('非法请求'); } } $csrf_token = md5(uniqid(rand(), true)); $_SESSION['csrf_token'] = $csrf_token; ?> <form method="post"> <input type="hidden" name="csrf_token" value="<?php echo $csrf_token;?>"> <input type="text" name="username"> <input type="password" name="password"> <button>提交</button> </form>
- Summary
By setting the expiration time for the PHP form, you can effectively prevent replay attacks and disguise attacks. , thereby enhancing form security. In addition, we can further strengthen the security of the form through data verification, preventing SQL injection, preventing XSS attacks and preventing CSRF attacks. In website development, strengthening security protection is crucial. We should start from all aspects to improve the security of the website.
The above is the detailed content of How to set expiration time in PHP form to strengthen security measures. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


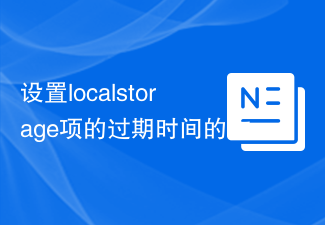
How to set the expiration time of localstorage requires specific code examples. With the rapid development of the Internet, front-end development often requires saving data in the browser. Localstorage is a commonly used WebAPI that aims to provide a way to store data locally in the browser. However, localstorage does not provide a direct way to set the expiration time. This article will introduce how to set the expiration time of localstorage through code examples.
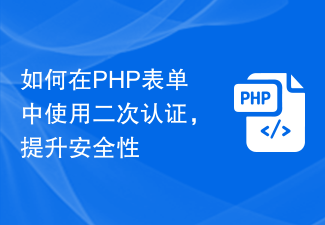
Nowadays, in the era of digitalization and networking, security has become one of the important factors that cannot be ignored in the Internet world. Especially in business scenarios with high data sensitivity, how to improve the security of websites, applications and user data is particularly important. Using two-step authentication in PHP forms to enhance security is a feasible solution. Two-Factor Authentication (2FA), also known as double authentication and multi-factor authentication, refers to the process where the user completes the regular account password.
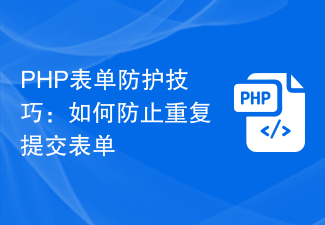
When using PHP forms for data submission, the problem of repeated form submission often occurs. This can lead to inaccurate data or, worse, system crashes. Therefore, it is very important to understand how to prevent duplicate submissions. In this article, I will introduce some PHP form protection techniques to help you effectively prevent repeated form submission problems. 1. Add a token to the form Adding a token to the form is a common way to prevent repeated submissions. The principle of this method is to add a hidden field to the form, which contains

How to extend the expiration time of Ajax requests? When making network requests, we often encounter situations where we need to process large amounts of data or complex calculations, which may cause the request to time out and fail to return data normally. In order to solve this problem, we can ensure that the request can be completed successfully by extending the expiration time of the Ajax request. The following will introduce some methods and specific code examples to extend the expiration time of Ajax requests. When making an Ajax request using the timeout attribute, you can set the timeout attribute to
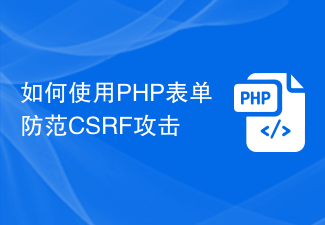
With the continuous development of network technology, security issues have increasingly become an issue that cannot be ignored in network application development. Among them, the cross-site request forgery (CSRF) attack is a common attack method. Its main purpose is to use the user to initiate an illegal request to the background by allowing the user to initiate a malicious request in the browser when the user is logged in to the website. This leads to server-side security vulnerabilities. In PHP applications, using form validation is an effective means of preventing CSRF attacks. Add CSRFToken to verify CSRF attacks
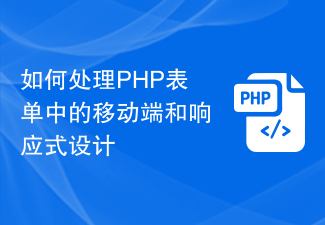
How to deal with mobile and responsive design in PHP forms. With the popularity and frequency of mobile devices increasing, and more and more users using mobile devices to access websites, adapting to mobile has become an important issue. When dealing with PHP forms, we need to consider how to achieve a mobile-friendly interface and responsive design. This article explains how to handle mobile and responsive design in PHP forms and provides code examples. 1. Responsive forms using HTML5 HTML5 provides some new features that can easily implement responsive forms.
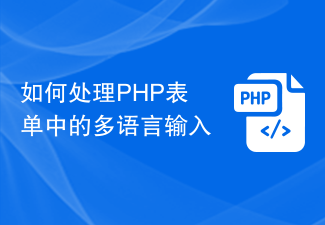
How to handle multi-language input in PHP forms With the development of globalization, multi-language support for websites has become a necessary feature. In PHP development, how to handle multi-language input so that users can enter data in different languages in the form and store and display the data correctly is an important issue that developers need to solve. This article will introduce how to use PHP to handle multi-language input and give corresponding code examples. 1. Set the language setting of the website In the PHP code, we can use setlocale
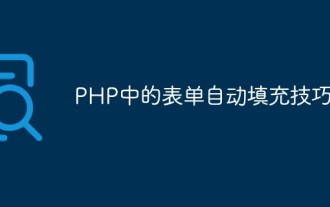
With the continuous development of the Internet, forms have become one of the functions we use on our daily websites. Getting users to fill out a form is undoubtedly a tedious task, so it’s necessary to use some tricks to simplify the process. This article will introduce techniques for implementing form autofill in PHP. 1. Use default value When setting the default value of the form, you can use the "value" attribute in the form tag to specify it. Here is an example: <inputtype="text"name=&q
