Golang learning the use of web framework gin
Usage of Web framework gin for Golang learning
With the development of the Internet, Web applications have become the standard configuration of various software systems. The server-side development languages for web applications are becoming more and more diversified. Among them, Golang's high performance and concise syntax style are increasingly favored by developers. This article will introduce one of the commonly used web frameworks in Golang: gin.
1. What is gin
gin is a web application framework written in Go language. It is based on the httprouter package and the net/http standard library, providing high performance, efficiency, ease of use and powerful functions. Use gin to quickly build a web server and support functions such as RESTful API, routing grouping, middleware, parameter binding, and template rendering.
2. Installation of gin
Before using the gin framework, you need to install gin first. You can use the following command line to install:
go get -u github.com/gin-gonic/gin
3. Basic use of gin
Below, we use an example to demonstrate how to use gin to write a simple Web service.
- Import the gin package
Import the gin package at the head of the code file:
import "github.com/gin-gonic/gin"
- Create the gin engine
Use the gin.New() method to create a new gin engine.
r := gin.New()
- Define routes
Use r.GET(), r.POST(), r.PUT() and r.DELETE() methods to define routes, Among them, the first parameter is the routing path, and the second parameter is the routing processing function.
r.GET("/", func(c *gin.Context) { c.String(http.StatusOK, "Hello World") })
- Start the service
Use the r.Run() method to start the service and specify the service address and port number.
r.Run(":8080")
The complete code is as follows:
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.New() r.GET("/", func(c *gin.Context) { c.String(http.StatusOK, "Hello World") }) r.Run(":8080") }
4. Gin routing
- Basic routing
In gin, the routing is It consists of routing methods, request paths and processing functions. The routing methods include GET, POST, PUT, DELETE, PATCH, HEAD, OPTIONS, etc. Use methods such as r.GET(), r.POST(), r.PUT(), and r.DELETE() to define routes.
r.GET("/index", func(c *gin.Context) { c.String(http.StatusOK, "Hello World") })
- Routes with parameters
Use colon (:) to define routes with parameters.
r.GET("/hello/:name", func(c *gin.Context) { name := c.Param("name") c.String(http.StatusOK, "Hello %s", name) })
- Routing for multiple request types
Use the r.Handle() method to define routing for multiple request types.
r.Handle("GET", "/hello", func(c *gin.Context) { c.String(http.StatusOK, "Hello World") }) r.Handle("POST", "/hello", func(c *gin.Context) { c.String(http.StatusOK, "Hello POST") })
- Routing grouping
Use the r.Group() method to implement routing grouping. You can add middleware and shared routing processing functions to the routing group.
v1 := r.Group("/v1") { v1.GET("/hello", func(c *gin.Context) { c.String(http.StatusOK, "Hello v1") }) }
5. Gin middleware
- Using middleware
Using gin middleware can achieve many useful functions, such as authentication and logging , caching, performance analysis, etc. Use the r.Use() method to register middleware.
r.Use(gin.Logger()) r.Use(gin.Recovery())
- Writing middleware
Writing middleware method is very simple. You only need to create a function with a parameter of type gin.Context and call it in the function. c.Next() method can realize the function of middleware.
func Auth() gin.HandlerFunc { return func(c *gin.Context) { if c.GetHeader("Authorization") != "token" { c.AbortWithStatus(http.StatusUnauthorized) } c.Next() } } r.GET("/hello", Auth(), func(c *gin.Context) { c.String(http.StatusOK, "Hello World") })
6. Gin parameter binding
- Binding Query parameters
Use the c.Query() method to obtain query parameters.
r.GET("/hello", func(c *gin.Context) { name := c.Query("name") age := c.Query("age") c.String(http.StatusOK, "name: %s, age: %s", name, age) })
- Bind POST form
Use the c.PostForm() method to obtain POST form data.
r.POST("/login", func(c *gin.Context) { username := c.PostForm("username") password := c.PostForm("password") c.JSON(http.StatusOK, gin.H{ "username": username, "password": password, }) })
- Bind JSON data
Use the c.BindJSON() method to bind JSON data.
type User struct { Name string `json:"name"` Password string `json:"password"` } r.POST("/login", func(c *gin.Context) { var user User if err := c.BindJSON(&user); err == nil { c.JSON(http.StatusOK, gin.H{ "name": user.Name, "password": user.Password, }) } else { c.JSON(http.StatusBadRequest, gin.H{ "error": err.Error(), }) } })
7. Gin template rendering
Using the gin framework can facilitate template rendering and supports multiple template engines, such as html, json, xml, etc.
- Install the template engine
Use the go get command to install the template engine.
go get -u github.com/gin-gonic/gin go get -u github.com/gin-gonic/contrib/jwt go get -u github.com/jinzhu/gorm
- Load template
Use the gin.LoadHTMLGlob() method to load the template file.
r.LoadHTMLGlob("html/*")
- Rendering template
Use the c.HTML() method to render the template.
r.GET("/html", func(c *gin.Context) { c.HTML(http.StatusOK, "index.html", gin.H{ "title": "Welcome Gin Web Framework", }) })
8. Conclusion
This article introduces the basic steps and techniques to build a Web server using the gin framework. Golang's high performance and concise syntax style make it more and more popular among developers in the development of web services. I hope this article can bring some inspiration and help to Golang web server developers.
The above is the detailed content of Golang learning the use of web framework gin. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
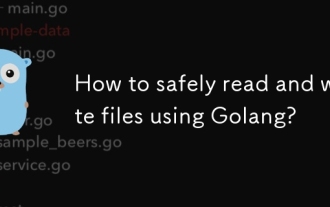
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
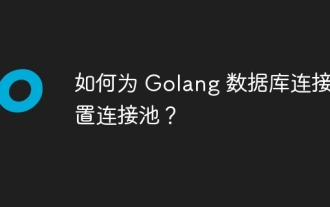
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
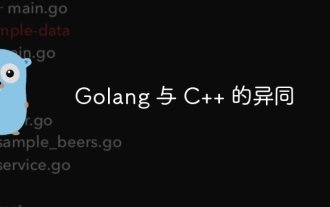
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
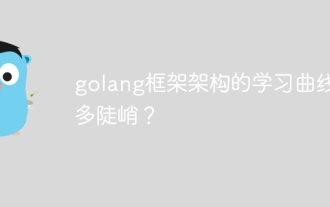
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
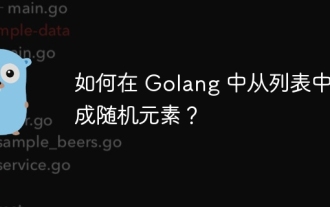
How to generate random elements of a list in Golang: use rand.Intn(len(list)) to generate a random integer within the length range of the list; use the integer as an index to get the corresponding element from the list.
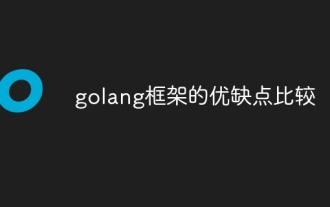
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
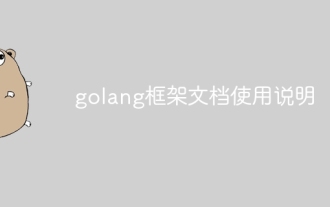
How to use Go framework documentation? Determine the document type: official website, GitHub repository, third-party resource. Understand the documentation structure: getting started, in-depth tutorials, reference manuals. Locate the information as needed: Use the organizational structure or the search function. Understand terms and concepts: Read carefully and understand new terms and concepts. Practical case: Use Beego to create a simple web server. Other Go framework documentation: Gin, Echo, Buffalo, Fiber.
