Golang learning Web application development based on AbanteCart
Golang is a fast, efficient and powerful programming language with powerful concurrent processing capabilities. It is also widely used in the field of web application development. This article will introduce Golang's web application development based on AbanteCart (a free and open source e-commerce platform).
1. Introduction to AbanteCart
AbanteCart is a free and open source e-commerce platform that can help developers easily build a fully functional online store. AbanteCart is characterized by strong scalability, easy customization, ease of use and high performance.
2. Start Golang development
In order to start Golang development, we need to install the Golang environment. You can download the installation program for the corresponding operating system from https://golang.org/dl/ for installation.
Next, we will use Golang’s web framework gin to create a web application based on AbanteCart.
1. Create a project
We first need to create a Golang project. Use the following command:
mkdir abantecart cd abantecart go mod init github.com/username/abantecart
2. Introduce the required dependency packages
Create a file named go.mod in the project root directory, and then enter the following content:
module github.com/username/abantecart go 1.16 require ( github.com/gin-gonic/gin v1.6.3 github.com/go-sql-driver/mysql v1.6.0 )
This file describes our project and its dependencies. Here we specify the gin framework and go-sql-driver/mysql driver version.
Use the following command to download the dependencies:
go mod download
Now we are ready to start developing our web application.
3. Connect to the database
AbanteCart uses the MySQL database to store data. We need to use the go-sql-driver/mysql driver to connect to the database:
import ( "database/sql" "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@/dbname") if err != nil { log.Fatal(err) } defer db.Close() }
Here, we use the sql.Open function to open a MySQL database connection. Please replace "user", "password" and "dbname" with the username, password and database name of your own MySQL database.
4. Create API routing
In order to create API routing, we will use the gin framework. We can use gin's Default function in the main function to create a new route:
import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() defer router.Run(":8080") }
Here, we use the gin.Default function to create a default gin engine, and then call the Run function to start the service and listen on the port to 8080.
5. Handling API requests
Now that we have created the API route, we can start handling requests from clients. We will use gin's GET function to create a GET request handler:
func main() { // ... router.GET("/products", func(c *gin.Context) { rows, err := db.Query(` SELECT products.product_id, products.product_name, products.product_price, products.product_quantity FROM abt_product_description INNER JOIN abt_products ON abt_product_description.product_id = abt_products.product_id `) var products []Product if err != nil { log.Printf("Error retrieving products from database: %v", err) } else { for rows.Next() { var product Product err := rows.Scan(&product.ID, &product.Name, &product.Price, &product.Quantity) if err != nil { log.Printf("Error scanning product data: %v", err) continue } products = append(products, product) } } c.JSON(http.StatusOK, gin.H{ "products": products, }) }) }
In this GET request handler, we use the db.Query function to retrieve product data from the database. We then convert this data into a Product object and add it to an array containing all the products. Finally, we return this array to the client as a JSON response.
6. Complete code example
package main import ( "database/sql" "log" "net/http" "github.com/gin-gonic/gin" _ "github.com/go-sql-driver/mysql" ) type Product struct { ID int `json:"id"` Name string `json:"name"` Price int `json:"price"` Quantity int `json:"quantity"` } func main() { db, err := sql.Open("mysql", "user:password@/dbname") if err != nil { log.Fatal(err) } defer db.Close() router := gin.Default() router.GET("/products", func(c *gin.Context) { rows, err := db.Query(` SELECT products.product_id, products.product_name, products.product_price, products.product_quantity FROM abt_product_description INNER JOIN abt_products ON abt_product_description.product_id = abt_products.product_id `) var products []Product if err != nil { log.Printf("Error retrieving products from database: %v", err) } else { for rows.Next() { var product Product err := rows.Scan(&product.ID, &product.Name, &product.Price, &product.Quantity) if err != nil { log.Printf("Error scanning product data: %v", err) continue } products = append(products, product) } } c.JSON(http.StatusOK, gin.H{ "products": products, }) }) defer router.Run(":8080") }
7. Run the application
Use the following command to start the application:
go run main.go
Now we have completed the Development of AbanteCart's web application, after running the application we can test it on the web page.
8. Summary
In this article, we have introduced how to use Golang's web framework gin and AbanteCart's API to develop an AbanteCart-based web application. We learned how to connect to a MySQL database and handle API requests. Hopefully our tutorial can help you start to better understand web application development in Golang.
The above is the detailed content of Golang learning Web application development based on AbanteCart. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
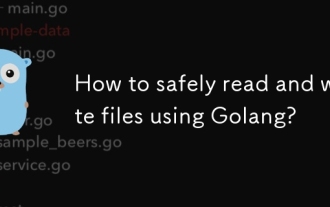
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
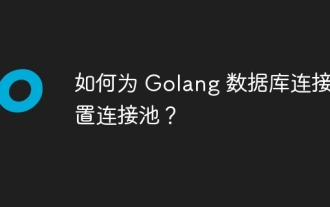
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
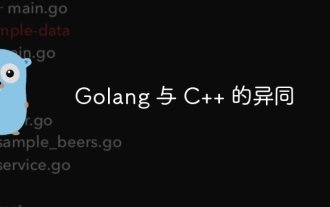
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
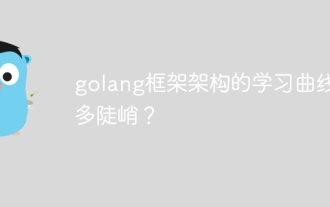
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
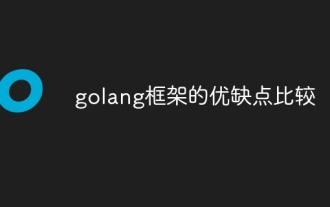
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
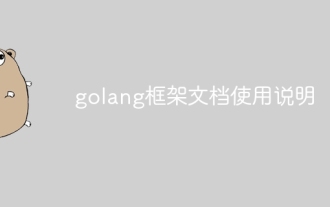
How to use Go framework documentation? Determine the document type: official website, GitHub repository, third-party resource. Understand the documentation structure: getting started, in-depth tutorials, reference manuals. Locate the information as needed: Use the organizational structure or the search function. Understand terms and concepts: Read carefully and understand new terms and concepts. Practical case: Use Beego to create a simple web server. Other Go framework documentation: Gin, Echo, Buffalo, Fiber.
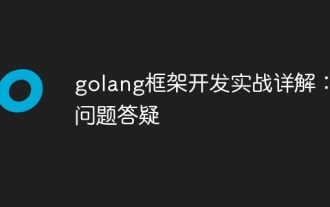
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
