


How to verify that the input string is in the correct IP address format using PHP regular expressions
PHP regular expression is a powerful tool that can be used to verify whether the format of the input string is correct. In network programming, the correctness of the IP address format is very important. In order to ensure that the entered IP address is legal, we need to use regular expressions for verification.
Regular expression is a pattern used to match or compare strings. It describes the pattern of a string through specific symbols and syntax rules, and checks whether a string conforms to this pattern.
In PHP, we can use the regular expression function preg_match() to verify the format of the IP address. The following is a sample code:
<?php $ip = "192.168.0.1"; if (preg_match("/^(([1-9]|[1-9]d|1d{2}|2[0-4]d|25[0-5]).){3}([1-9]|[1-9]d|1d{2}|2[0-4]d|25[0-5])$/", $ip)) { echo "IP地址格式正确"; } else { echo "IP地址格式不正确"; } ?>
In this code, we define an IP address variable $ip and verify it using the preg_match() function. Among them, the regular expression "/^(([1-9]|[1-9]d|1d{2}|2[0-4]d|25[0-5]).){3}([ 1-9]|[1-9]d|1d{2}|2[0-4]d|25[0-5])$/" is used to match the format of IP addresses.
In regular expressions, the regular characters "^" and "$" are used to match the beginning and end of a string. Brackets "()" are used for grouping, allowing us to match each part of the IP address repeatedly. Grouping techniques can be used in the code to match numbers between 0 and 255, where "|" represents or, colon ":" represents range, and braces "{}" represent repetitions. For example, "d{3}" represents matching 3 numbers. . So the final regular expression can match the format of the IP address.
The output of this code is "The IP address format is correct." If the value of the $ip variable does not conform to the format of the IP address, the output result will be "IP address format is incorrect".
In addition to using the preg_match() function, we can also use other regular expression functions to perform IP address format verification, such as preg_replace() and preg_match_all(), etc.
In short, PHP regular expression is a very powerful tool that can be used to verify whether the format of the input string is correct. In network programming, it is very important to verify the correct format of the IP address. Pay attention to using regular expressions for verification.
The above is the detailed content of How to verify that the input string is in the correct IP address format using PHP regular expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


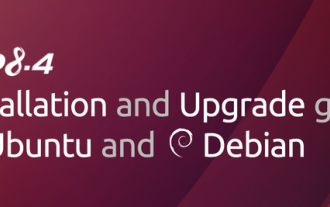
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
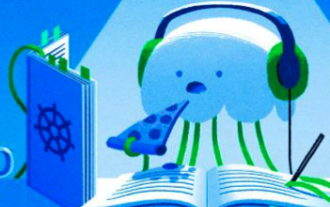
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
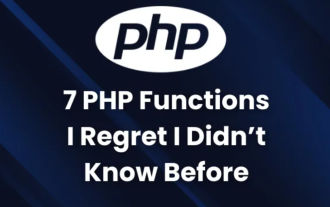
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
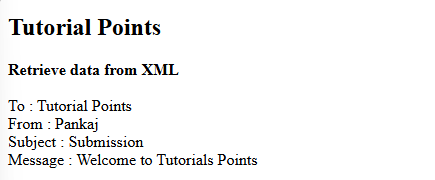
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
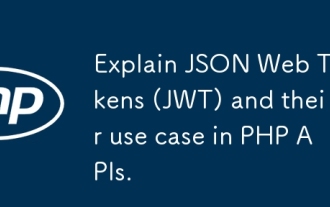
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
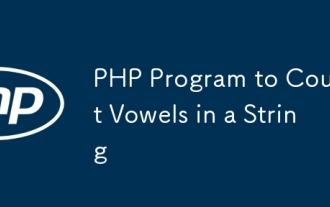
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
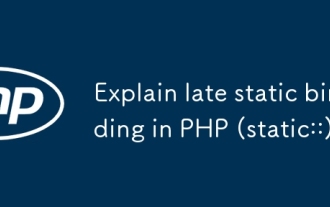
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
