


How to solve the 'cannot use x (type y) as type z in range' error in golang?
Golang is a powerful programming language with features such as high efficiency, concurrency, and built-in garbage collection mechanism, and is favored by many programmers. However, when programming with Golang, sometimes you will encounter the problem as shown in the title: "cannot use x (type y) as type z in range" error. What does this error mean? How do we solve it? This article will provide you with a solution.
Error explanation
First, let us understand the background of this error.
The range syntax in Golang can be used in various data types such as arrays, slices, maps, and channels. When using the range syntax, you can use two variables to receive the value in the loop body, for example:
1 2 3 4 |
|
The program will output:
1 2 3 |
|
However, sometimes we will encounter an error message: "cannot use x (type y) as type z in range", this error is caused by the variable type mismatch when the range statement traverses the data. Among them, x is the current element, y is the type of the current element, and z is the type of the variable. For example:
1 2 3 4 5 6 7 8 |
|
The above code will generate the following error message:
1 |
|
This is due to the When using the range syntax on a slice a of type myInt, the type of variable v is inferred to be myInt instead of int.
Solution
So, how do we solve this problem? According to the characteristics of the Go language, this problem can be solved through type conversion. Therefore, we need to convert the type of the current element to the type of the variable, for example:
1 2 3 4 5 6 7 8 |
|
The above code will output:
1 2 3 |
|
In addition, sometimes we can also directly modify the type of the variable to the current The type of element, for example:
1 2 3 4 5 6 7 8 |
|
The above code will produce the following error message:
1 |
|
This is due to using the range syntax on an empty slice a, and there are no elements to traverse at this time. In order to solve the above problem, we can change the type of the variable to the type of the current element. The code is as follows:
1 2 3 4 5 6 7 8 |
|
The above code will output a blank line because there are no elements in slice a to traverse.
Summary
This article introduces the background and solution of the "cannot use x (type y) as type z in range" error in Golang. When using range syntax to traverse data, you need to pay attention to whether the type of the variable matches the type of the current element. If the types do not match, you can use type conversion or modify the type of the variable to solve the problem. At the same time, we also need to pay attention to whether data structures such as slices are empty to prevent similar error messages.
The above is the detailed content of How to solve the 'cannot use x (type y) as type z in range' error in golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
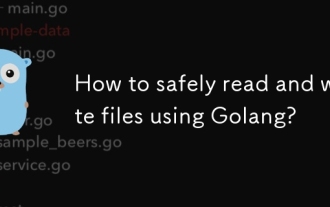
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
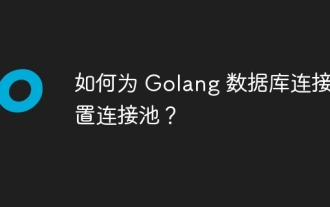
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
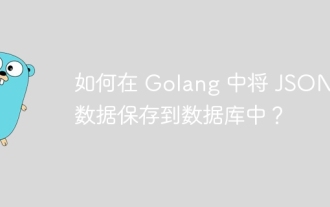
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
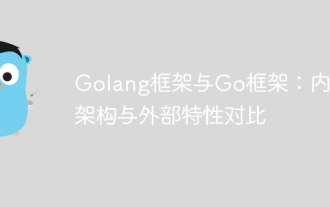
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
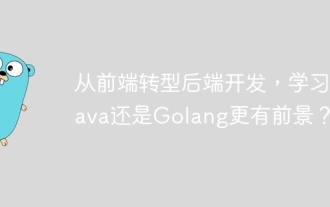
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
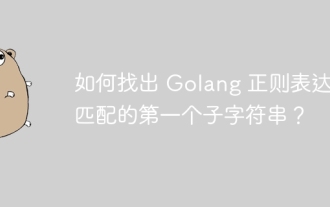
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
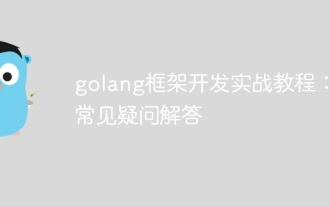
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
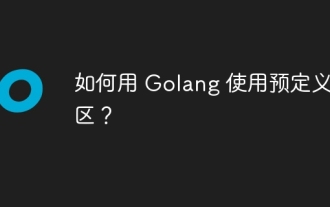
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
