How to solve long function error in Python code?
When writing Python code, you may encounter a situation where the function is very long. Even if you are an experienced developer, you may be frustrated by long functions. Long functions make code difficult to understand and maintain, and may even lead to errors. In this article, we will explore some ways to resolve long function errors in Python code.
- Split long functions into smaller functions
Splitting large functions into smaller functions is probably one of the best ways to reduce the complexity of your code. Each small function is only responsible for performing a specific operation. In this way, the code becomes easy to understand and refactor. If a function is too lengthy, break it into parts first, and then split each part into smaller functions.
For example, suppose you have a long function named calculate_salary
whose purpose is to calculate the salary of a company's employees. You can create several other functions like get_employee_details
, calculate_basic_salary
, calculate_bonus
etc. and then call these functions in calculate_salary
. This approach makes the code structure clearer and easier to maintain.
- Using Lambda expressions
Lambda expression is a Python syntax that can effectively reduce the length of a function. A lambda expression is an anonymous function that can be defined in one line of code. Lambda expressions are often used to write short functions that do not require saving a name.
For example, within a long function, you might need to use a simple function to calculate the average of a set of numbers. You can use Lambda expressions, which will greatly shorten the code length. You can write code like this:
average = lambda nums: sum(nums) / len(nums)
Then, in your long function, you can call this Lambda expression to calculate the average without creating a function name. This approach makes the code more concise and easier to read.
- Writing Comments and Documentation
Even if you have a deep understanding of your code, others may still find it difficult to understand. When you are dealing with a long function, you may find that simply breaking the code into smaller functions is not enough. In this case, writing comments and documentation can help explain the code clearly to others.
Make sure your comments are concise and clear. Comments should explain important things in the code, not things that are obvious in the code. It's good practice to use well-formed docstrings and write documentation for every important part of your code.
- Using Exception Handling
When you write a long function, it can be difficult to understand how exceptions are handled throughout the function. In this case, you may want to use Python's exception handling mechanism to balance code correctness and safety with code length and readability.
Python's exception handling allows you to handle potential error conditions. If you write error handling along with your code, you can more clearly express what your code means. You can make your code more readable by using try
and except
blocks to locate and handle potential errors.
Conclusion
Long functions can make code difficult to understand and maintain, and can lead to errors. This article introduces some solutions for dealing with long functions, including splitting long functions into smaller functions, using lambda expressions, writing comments and documentation, and using exception handling. These tips can help you better organize your code and make it easier to understand and maintain. Whether you're a novice developer or an experienced pro, these tips can make your code clearer and easier to read.
The above is the detailed content of How to solve long function error in Python code?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


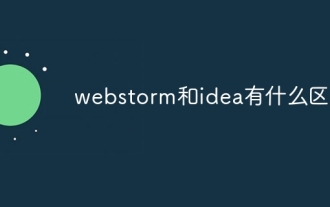
WebStorm is tailor-made for web development and provides powerful features for web development languages, while IntelliJ IDEA is a versatile IDE that supports multiple languages. Their differences mainly lie in language support, web development features, code navigation, debugging and testing capabilities, and additional features. The final choice depends on language preference and project needs.
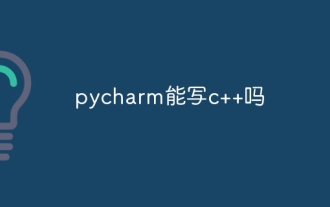
Yes, PyCharm can write C++ code. It is a cross-platform IDE that supports multiple languages, including C++. After installing the C++ plugin, you can use PyCharm's features such as code editor, compiler, debugger, and test runner to write and run C++ code.
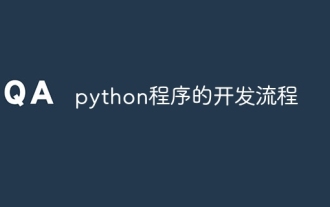
The Python program development process includes the following steps: Requirements analysis: clarify business needs and project goals. Design: Determine architecture and data structures, draw flowcharts or use design patterns. Writing code: Program in Python, following coding conventions and documentation comments. Testing: Writing unit and integration tests, conducting manual testing. Review and Refactor: Review code to find flaws and improve readability. Deploy: Deploy the code to the target environment. Maintenance: Fix bugs, improve functionality, and monitor updates.
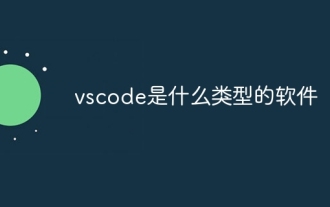
VSCode is a free and open source code editor. Its main functions include: syntax highlighting and intelligent code completion, debugging and diagnostic extensions, support for code navigation and refactoring, integrated terminal version control, integrated multi-platform support.
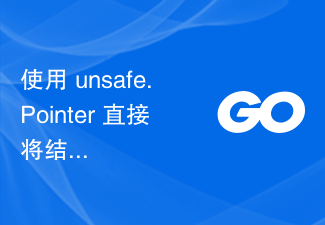
is it safe? (*teamdata)(unsafe.pointer(&team.id)) Sample code: functestTrans()[]*TeamData{teams:=createTeams()teamDatas:=make([]*TeamData,0,len(teams))for_, team:=rangeteams{//isthissafe?teamDatas=append(teamDatas,
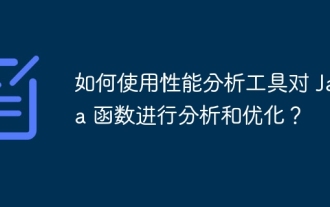
Java performance analysis tools can be used to analyze and optimize the performance of Java functions. Choose performance analysis tools: JVisualVM, VisualVM, JavaFlightRecorder (JFR), etc. Configure performance analysis tools: set sampling rate, enable events. Execute the function and collect data: Execute the function after enabling the profiling tool. Analyze performance data: identify bottleneck indicators such as CPU usage, memory usage, execution time, hot spots, etc. Optimize functions: Use optimization algorithms, refactor code, use caching and other technologies to improve efficiency.
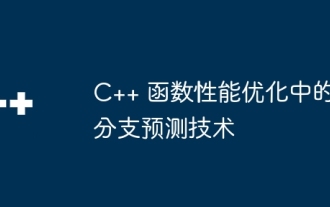
Branch prediction technology can optimize C++ function performance by predicting branch jump directions. Branch prediction techniques in C++ include: Static branch prediction: prediction based on branch patterns and history. Dynamic branch prediction: updates the prediction table based on runtime results. Optimization suggestion: Use likely() and unlikely() to prompt the compiler. Optimize branch conditions using simple comparisons. Reduce the number of branches, merge branches or use the ternary operator. Use loop unrolling to eliminate branches. Use inline functions to eliminate function call overhead. Benchmarking helps evaluate optimization effectiveness and determine the best strategy.
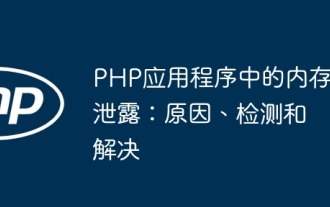
A PHP memory leak occurs when an application allocates memory and fails to release it, resulting in a reduction in the server's available memory and performance degradation. Causes include circular references, global variables, static variables, and expansion. Detection methods include Xdebug, Valgrind and PHPUnitMockObjects. The resolution steps are: identify the source of the leak, fix the leak, test and monitor. Practical examples illustrate memory leaks caused by circular references, and specific methods to solve the problem by breaking circular references through destructors.
