


No subsequent object: How to solve Python's missing object error?
Python is a high-level programming language, and its error messages are relatively friendly, but sometimes "Missing Object" errors may occur. This error often occurs during method invocation or property access, and is usually caused by the object not existing. Next we’ll explore how to resolve missing object errors in Python.
The first thing to understand is that the "Missing Object" error is closely related to the "NoneType" type of error. In Python, the NoneType type represents a null object or null value, and is usually used to represent the situation when the return value of a function or method is null. In practice, if there is a missing object error in your code, you can try the following methods to solve it.
1. Check whether the object exists
Before the code refers to the object, you should make sure that the object already exists. Typically, this involves checking to see if a previous statement has already created the object. If this object is not part of the program, you can try to use a try-except statement to catch the exception to prevent the program from crashing.
For example, the following code calls a non-existent object:
x = y.z
To solve this problem, you can first check whether the y object exists:
if hasattr(y, 'z'): x = y.z else: print('Error: y object does not have attribute z')
Use hasattr() Function to check whether the object has a specific attribute, if so, continue the operation, otherwise print an error message.
2. Check whether the variable name is correct
Sometimes missing object errors may be caused by spelling errors. In Python programming, capitalization is case-sensitive, so if a variable name is spelled in the wrong case, Python will not be able to find the variable. Therefore, when checking for spelling errors in variable names, pay attention to correct capitalization and spelling.
For example, the case of the variable name in the following code is misspelled:
number = 10 print(NUMBER)
To solve this problem, you should ensure that the case of the variable name matches:
number = 10 print(NUMBER)
3. Check Is the code logic correct?
Sometimes missing object errors may be caused by code logic problems. In this case, you need to check whether the logical flow of the code is correct. For example, trying to access an undefined object, call an undefined function, or index an element from an empty list. In this case, you should first check whether the code logic is correct and make changes if necessary.
For example, in the following code, try to get the first element from an empty list:
my_list = [] first_element = my_list[0]
In order to solve this problem, you should first check whether the list is empty:
my_list = [] if my_list: first_element = my_list[0] else: print('Error: the list is empty')
When using a list or other data structure, you should first check if it is empty to prevent missing object errors.
4. Use default values
When dealing with null values returned by functions or methods, you can use default values to replace missing objects. In Python, you can use default parameters to define default values for a function or method to be used when the function or method does not return a value.
For example, in the following code, try to convert an empty object to an int type:
x = int(None)
To solve this problem, you can use the default value 0 to replace the missing object:
x = int(None or 0)
In this example, if None is empty, 0 is used as the default value.
It should be noted that when using default values, you should ensure that the default values match the actual parameter types, otherwise other errors will result.
In closing, it is important to emphasize that missing object errors are usually caused by some reason, and the problem can be found and solved by carefully inspecting the code. If you can't find the cause, you can try using debugging tools to diagnose the problem in your code. When dealing with such errors, you should be patient and always check whether the code logic is correct first to avoid blindly fixing the code.
The above is the detailed content of No subsequent object: How to solve Python's missing object error?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
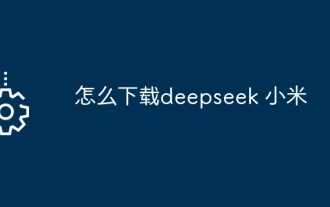
How to download DeepSeek Xiaomi? Search for "DeepSeek" in the Xiaomi App Store. If it is not found, continue to step 2. Identify your needs (search files, data analysis), and find the corresponding tools (such as file managers, data analysis software) that include DeepSeek functions.
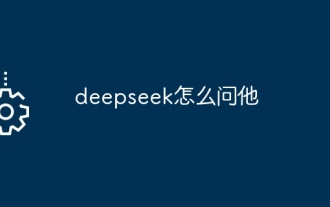
The key to using DeepSeek effectively is to ask questions clearly: express the questions directly and specifically. Provide specific details and background information. For complex inquiries, multiple angles and refute opinions are included. Focus on specific aspects, such as performance bottlenecks in code. Keep a critical thinking about the answers you get and make judgments based on your expertise.
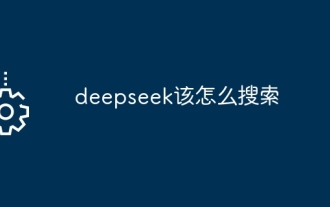
Just use the search function that comes with DeepSeek. Its powerful semantic analysis algorithm can accurately understand the search intention and provide relevant information. However, for searches that are unpopular, latest information or problems that need to be considered, it is necessary to adjust keywords or use more specific descriptions, combine them with other real-time information sources, and understand that DeepSeek is just a tool that requires active, clear and refined search strategies.
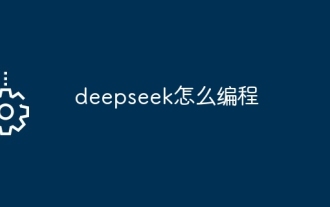
DeepSeek is not a programming language, but a deep search concept. Implementing DeepSeek requires selection based on existing languages. For different application scenarios, it is necessary to choose the appropriate language and algorithms, and combine machine learning technology. Code quality, maintainability, and testing are crucial. Only by choosing the right programming language, algorithms and tools according to your needs and writing high-quality code can DeepSeek be successfully implemented.
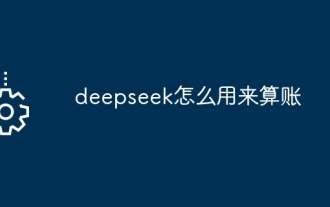
Question: Is DeepSeek available for accounting? Answer: No, it is a data mining and analysis tool that can be used to analyze financial data, but it does not have the accounting record and report generation functions of accounting software. Using DeepSeek to analyze financial data requires writing code to process data with knowledge of data structures, algorithms, and DeepSeek APIs to consider potential problems (e.g. programming knowledge, learning curves, data quality)
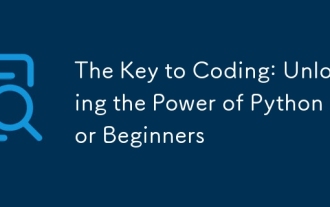
Python is an ideal programming introduction language for beginners through its ease of learning and powerful features. Its basics include: Variables: used to store data (numbers, strings, lists, etc.). Data type: Defines the type of data in the variable (integer, floating point, etc.). Operators: used for mathematical operations and comparisons. Control flow: Control the flow of code execution (conditional statements, loops).
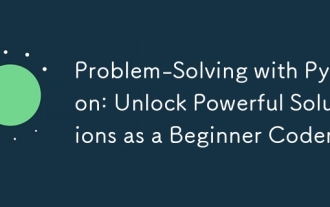
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
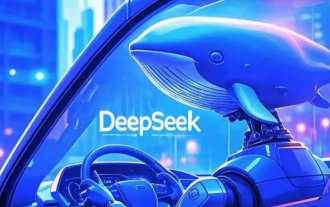
Detailed explanation of DeepSeekAPI access and call: Quick Start Guide This article will guide you in detail how to access and call DeepSeekAPI, helping you easily use powerful AI models. Step 1: Get the API key to access the DeepSeek official website and click on the "Open Platform" in the upper right corner. You will get a certain number of free tokens (used to measure API usage). In the menu on the left, click "APIKeys" and then click "Create APIkey". Name your APIkey (for example, "test") and copy the generated key right away. Be sure to save this key properly, as it will only be displayed once
