How to solve 'undefined: database/sql.Open' error in golang?
Go is a fast, efficient, compiled programming language. Due to its excellent performance and readability, it has gradually been favored by more and more developers in recent years. Among them, database/sql is an important package in Go, which provides an interface for developers to interact with the database. However, during the use of database/sql.Open, developers may encounter a classic error: "undefined: database/sql.Open". This article details the causes of this error and provides several solutions.
Error reason
In the Go language, a symbol starting with a capital letter indicates that the symbol is public and can be called and used in other packages. Symbols starting with a lowercase letter indicate that the symbol is private and can only be used in the package that defines the symbol. In the database/sql package, Open is a public function, so we can use it in other programs. However, when we use database/sql.Open in the program, we may encounter the following error message:
undefined: database/sql.Open
This error usually occurs in the following two situations:
- Forgot to import the database/sql package
- Forgot to import the database driver package
Solution
Import the database/sql package
General situation Next, we will import the database/sql package before using the Open function. However, if we forget to import the package or the imported package name is incorrect, it is easy to cause the above error. If you encounter this situation, you need to make sure you import the database/sql package first in your code. If you cannot find the path to the package, you can enter the following command on the command line to view the installation location of the Go environment used by the program:
go env GOROOT
Then, import the database/sql package in the code, for example:
import "database/sql"
Importing the database driver package
Another common mistake is forgetting to import the database driver package. When we use database/sql.Open to connect to the database, we need to import the driver for the specific database at the same time. For example, when using a MySQL database, we need to import the mysql package. If you encounter this issue, you need to make sure you import the correct driver package in your code. You can find the driver package of the corresponding database and its import path in the official documentation.
import "database/sql" import _ "github.com/go-sql-driver/mysql"
For example, when using a MySQL database, you can add the above code to your code, where "_" means that the package only performs initialization at runtime without using it explicitly in the code.
Specify database driver
In addition to importing the correct database driver package, you also need to specify the database driver to be used in the code. This can be done by specifying the MySQL driver after importing "github.com/go-sql-driver/mysql" in the code. The following is a specific example:
package main import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@/database") if err != nil { // 处理错误 } // 在这里使用 db 连接数据库 }
In the above code, we use the "github.com/go-sql-driver/mysql" package and specify the requirements in the program The MySQL driver used, the "mysql" string. This string will be passed to the sql.Open function to obtain a *sql.DB object that can be used to connect to a MySQL database.
Summary
When using Go's database/sql package for database operations, you often encounter the "undefined: database/sql.Open" error. This error is usually caused by forgetting to import the database/sql package or the driver package for a specific database. To resolve this error, you should ensure that these packages are imported correctly in your code and that you explicitly specify the database driver to use in your code. I believe this article provides a reference for everyone to solve this problem.
The above is the detailed content of How to solve 'undefined: database/sql.Open' error in golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


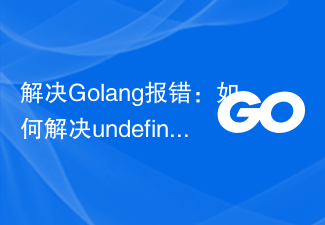
When developing with Golang, you often encounter undefinedidentifier errors. This error is caused by the presence of undefined identifiers in the code. In this article, we will cover common undefinedidentifier errors and their solutions. 1. Why does the undefinedidentifier error occur? Golang is a statically typed language, so

Advantages and Disadvantages of Using Golang to Develop Mobile Games With the popularity of mobile devices and the continuous improvement of their performance, the mobile game market is becoming more and more popular, attracting more and more developers to join it. When choosing a development language, Golang, as a fast, efficient and easy-to-learn language, attracts the attention of many developers. This article will discuss the advantages and disadvantages of using Golang to develop mobile games, and illustrate it through specific code examples. Advantages: Strong cross-platform: Golang can be compiled into binaries for different platforms
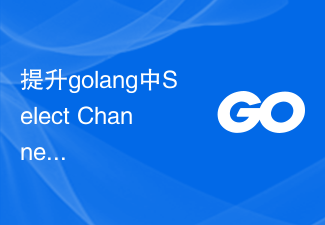
Methods to improve the efficiency of SelectChannelsGo concurrent programming in golang Introduction: With the continuous development of computer technology, multi-core and concurrent programming have gradually become an important direction in application development. In Go language, concurrent programming can be easily achieved by using goroutines and channels. The Select statement is a key tool for managing and controlling multiple channels. In this article, we will explore how to improve the use of Sele in golang

Analysis and practice of atomicity of variable assignment in Golang In concurrent programming, it is crucial to ensure the atomicity of data. In Golang, some mechanisms are provided to ensure the atomicity of variable assignment. This article will focus on the analysis and practice of this topic. 1. The concept of atomic operations In concurrent programming, atomic operations refer to operations that will not be interrupted by other threads. They are either completed or not executed at all. In Golang, atomic operations can be implemented through functions in the sync/atomic package. These functions
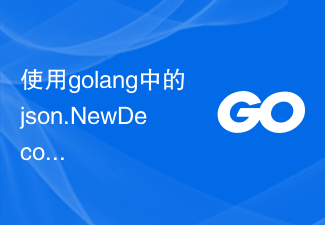
Streaming encoding and decoding of JSON using json.NewDecoder and json.NewEncoder functions in golang JSON is a lightweight data exchange format that is widely used in web applications and modern APIs due to its ease of reading and writing. In golang, we can use the json package to encode and decode JSON data. The json.NewDecoder and json.NewEncoder functions provide a stream
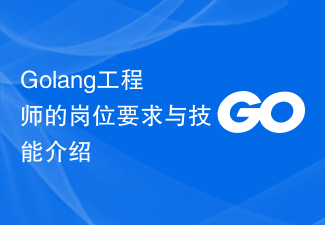
Job requirements and skill introduction for Golang engineers. With the rapid development of the Internet industry, Golang, as an efficient, concise and high-concurrency programming language, has gradually become favored by more and more companies. Therefore, the market demand for engineers with Golang skills is becoming increasingly strong. So, what job requirements and skills should a good Golang engineer have? Next, we'll introduce it, with specific code examples. 1. Job requirements: 1. Proficient in Golang programming
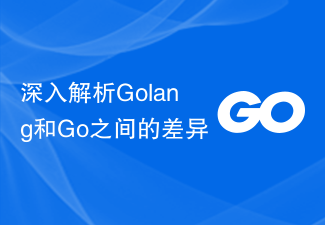
An in-depth analysis of the differences between Golang and Go Overview Golang and Go are two names for the same programming language. They refer to a simple, efficient, and concurrency-safe programming language developed by Google. Golang is the full name of the language, while Go is its more commonly used abbreviation. In this article, we will delve into the differences between Golang and Go and understand their development history, features, and usage scenarios. Development History The development of Golang can be traced back to 2007, by RobPike
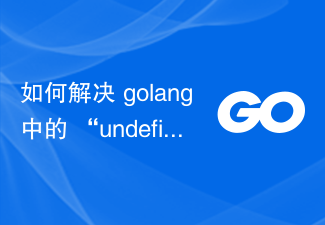
Go is a fast, efficient, compiled programming language. Due to its excellent performance and readability, it has gradually been favored by more and more developers in recent years. Database/sql is an important package in Go, which provides an interface for developers to interact with the database. However, in the process of using database/sql.Open, developers may encounter a classic error: "undefined: database/sql.Open". This article
