Golang error: 'unexpected end of JSON input' How to solve it?
In recent years, the go language (also known as golang) developed and launched by Google has become one of the choices of many developers. Golang is widely used for its fast compilation speed, efficient memory management and powerful network programming capabilities. But during development, we may encounter various problems. For example, when using the JSON parsing library, we may encounter the error "unexpected end of JSON input".
What is the "unexpected end of JSON input" error?
This error will be triggered when the end of the text is encountered while parsing the JSON text and the entire JSON text cannot be parsed correctly.
Use the encoding/json package to parse JSON in the go language. When we convert JSON into a struct object or map object, we can use the json.Unmarshal method to parse it.
For example, we have such an HTTP response message:
HTTP/1.1 200 OK Content-Type: application/json {"code": 200, "message": "success", "data": {"name": "John", "age": 18}}
In order to convert this JSON string into a struct object, we can do this:
type Response struct { Code int `json:"code"` Message string `json:"message"` Data struct { Name string `json:"name"` Age int `json:"age"` } `json:"data"` } ... resp, err := http.Get(url) if err != nil { // handle error } defer resp.Body.Close() var result Response decoder := json.NewDecoder(resp.Body) err = decoder.Decode(&result) if err != nil { // handle error }
In the above In the example, we obtain the HTTP response from the URL through http.Get and convert the JSON format in the response body into a Response struct object. When the JSON format is incorrect, the "unexpected end of JSON input" error will be triggered.
how to solve this problem?
When processing JSON format, we need to pay attention to some details, such as the correctness of JSON format. When parsing JSON text, you may find that the JSON format is incorrect, such as missing commas, missing quotes, or missing parentheses, etc. If we use the json.Unmarshal method, we must ensure that the JSON format is correct, otherwise we will encounter the "unexpected end of JSON input" error.
In the sample code, we parse the response body in JSON format into a Response structure through decoder.Decode(&result). However, if the response body format is incorrect, "unexpected end of JSON input" will be triggered. "mistake.
In order to solve this problem, we should verify the JSON format of the response body. We can use some tools, such as JSONLint, to verify the JSON format. If the JSON is well-formed, it can be parsed successfully. If the JSON format is incorrect, you need to fix the JSON format to correctly parse the response body.
In actual encoding, we can use the following approach to verify the JSON format:
resp, err := http.Get(url) if err != nil { // handle error } defer resp.Body.Close() result := make(map[string]interface{}) decoder := json.NewDecoder(resp.Body) decoder.UseNumber() // 避免JSON数字溢出 err = decoder.Decode(&result) if err != nil { // handle error }
In the above example, we first created an empty map object. Then we obtain a decoder object through the json.NewDecoder method and parse the response body using the decoder.Decode method. We also call the decoder.UseNumber method to avoid JSON number overflow.
We need to handle errors when the JSON format is incorrect. We can handle errors using the following code:
respBody, err := ioutil.ReadAll(resp.Body) if err != nil { // handle error } if err := json.Unmarshal(respBody, &result); err != nil { fmt.Println("JSON parse error:", err) return err }
In the above example, we first read the response body and parsed the JSON text using the json.Unmarshal method. If the JSON format is incorrect, an error message is returned.
Through the above method, we can avoid the occurrence of "unexpected end of JSON input" errors and ensure that our code can correctly parse the JSON format. In actual development, we should also pay attention to the correctness and legality of the JSON format to ensure that our code can process data accurately and efficiently.
The above is the detailed content of Golang error: 'unexpected end of JSON input' How to solve it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
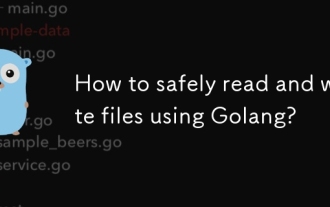
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
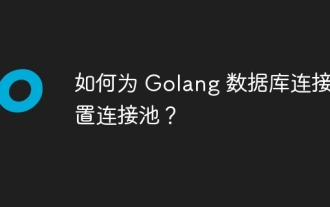
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
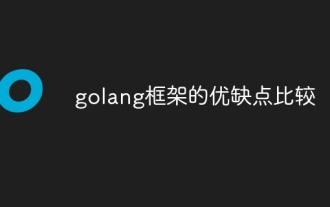
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
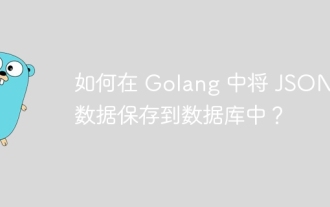
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
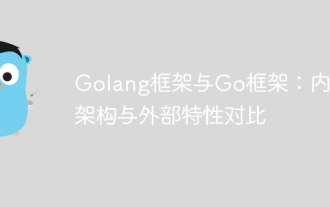
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
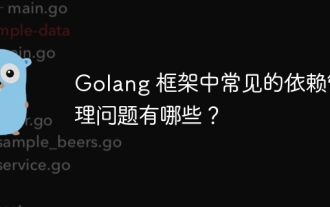
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
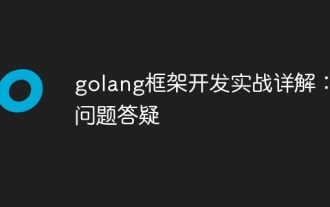
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
