How to handle InstantiationException in Java?
Java is a widely used programming language. It has powerful object-oriented programming features and rich class libraries. However, it is inevitable to encounter various abnormal situations during the development process using Java. One of the common exceptions is InstantiationException. This article will focus on how to handle InstantiationException in Java.
1. What is InstantiationException
InstantiationException is an exception class in Java. When trying to instantiate an abstract class or interface through the newInstance() method in the Class class during runtime, or When an error occurs during the creation of a class instance object through the class's constructor, an InstantiationException is thrown.
When using these two methods to create objects, you need to ensure that the specified class must have a public parameterless constructor, otherwise an InstantiationException will be thrown.
2. Causes of InstantiationException
- Abstract classes or interfaces cannot be instantiated: even when trying to instantiate an abstract class or interface using the newInstance() method in the Class class, InstantiationException will be thrown.
- The class has no parameterless constructor: If you want to instantiate an object of a class through the Class.newInstance() method, then the class must have a public parameterless constructor. If there is no such constructor, an InstantiationException will be thrown.
3. How to handle InstantiationException
- Use the parameterless constructor to create an object
Using the parameterless constructor of a class to create an object is Best solution. If there is no parameterless constructor in the class, you must add such a constructor so that you can create an object instance when you use the static newInstance() method to create an object.
For example:
1 2 3 |
|
- Use reflection to create objects
In the reflection API, use the newInstance() method of the java.lang.reflect.Constructor class When creating an object, if you include parameters, you must provide the class type and value of the corresponding parameter.
For example:
1 2 3 |
|
- Throw exception
If the correct constructor is not provided when creating the object, use a try-catch block to throw abnormal.
For example:
1 2 3 4 5 6 7 |
|
4. How to avoid InstantiationException
- Add a parameterless constructor to the class
In order to avoid InstantiationException , the best way is to add a parameterless constructor to the class.
- Use its subclasses
If the parent class cannot be instantiated, you can use its subclass to create objects, because the subclass itself has a no-argument constructor Function class. For example:
1 2 3 |
|
When creating objects, you can use the Student class instead of the Person class.
3. Summary
In Java development, InstantiationException often occurs. To avoid this exception, the best way is to add a parameterless constructor to the class. If you cannot change the source code of a class, you can use a subclass of the class to create an object. Creating objects using the reflection API may be more tedious, but it is another way to solve the InstantiationException exception. Either way, you need to make sure you provide the correct constructor when creating the object.
The above is the detailed content of How to handle InstantiationException in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


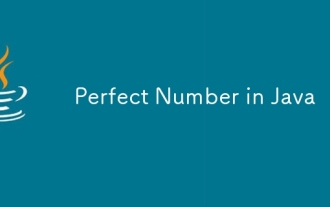
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
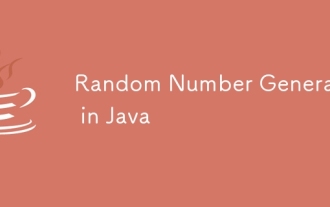
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
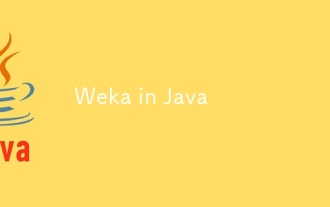
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
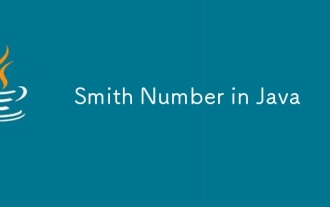
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
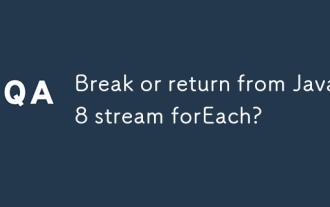
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
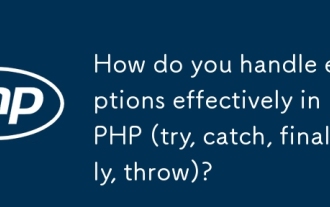
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
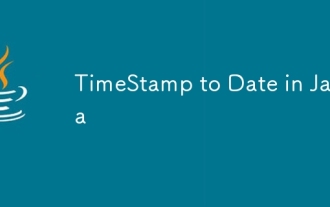
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
