How to implement infinite scrolling list with Vue?
In web applications, scrolling lists are a very common way to display data, while infinite scrolling lists are a way to dynamically load more data. It is not difficult to implement an infinite scrolling list in Vue. With some simple operations, we can easily implement an infinite scrolling list.
- Preparing data
First, we need to prepare the data to be displayed. Generally, this data is obtained through interfaces. In this example, we can use a fake data source to simulate obtaining data:
const data = [ { id: 1, content: '第1条数据' }, { id: 2, content: '第2条数据' }, { id: 3, content: '第3条数据' }, { id: 4, content: '第4条数据' }, { id: 5, content: '第5条数据' }, { id: 6, content: '第6条数据' }, { id: 7, content: '第7条数据' }, { id: 8, content: '第8条数据' }, { id: 9, content: '第9条数据' }, { id: 10, content: '第10条数据' } ];
- Realize infinite scroll
Next, we need to use Vue instructionsv-infinite-scroll
to achieve infinite scrolling. First, in our template, we need a container to display data, and set a directive for this container, as shown below:
<div class="list" v-infinite-scroll="loadMore"> <div v-for="item in items" :key="item.id" class="item">{{ item.content }}</div> </div>
Here, we pass v-infinite-scroll
Instruction to trigger the corresponding method loadMore
. This method will dynamically load more data based on the currently displayed data. In addition, in this container, we use the v-for
directive to traverse the entire data list and display it on the page.
Next, we need to implement the loadMore
method. In this method, first get the index lastIndex
of the last data in the current data list, and then use some asynchronous operations to dynamically load more data and add these data to the current data list.
methods: { loadMore() { const lastIndex = this.items.length - 1; const lastItem = this.items[lastIndex]; const nextIndex = lastItem.id + 1; setTimeout(() => { const newData = data .slice(nextIndex - 1, nextIndex + 9) .map(item => { return { id: item.id, content: item.content }; }); this.items = [...this.items, ...newData]; }, 1000); } }
Here, we use setTimeout
to simulate the operation of asynchronously loading data. First, get the index lastIndex
of the last piece of data in the current data list and use it as the starting point for loading more data. Then, use the slice
method to intercept a piece of data from the data source, and use the map
method to convert it into the data format used by the current application. Finally, add these new data to the current data list.
It should be noted that when we load the data, we do not load all the data at once, but only load the subsequent ten pieces of data each time through the slice
method. The advantage of this is that it can improve the performance of the application and avoid loading a large amount of data at one time from causing excessive burden on the application.
- Complete code
The following is the complete sample code, including data preparation, template and method implementation.
<template> <div class="list" v-infinite-scroll="loadMore"> <div v-for="item in items" :key="item.id" class="item">{{ item.content }}</div> </div> </template> <script> const data = [ { id: 1, content: '第1条数据' }, { id: 2, content: '第2条数据' }, { id: 3, content: '第3条数据' }, { id: 4, content: '第4条数据' }, { id: 5, content: '第5条数据' }, { id: 6, content: '第6条数据' }, { id: 7, content: '第7条数据' }, { id: 8, content: '第8条数据' }, { id: 9, content: '第9条数据' }, { id: 10, content: '第10条数据' } ]; export default { data() { return { items: data.slice(0, 10).map(item => { return { id: item.id, content: item.content }; }) }; }, methods: { loadMore() { const lastIndex = this.items.length - 1; const lastItem = this.items[lastIndex]; const nextIndex = lastItem.id + 1; setTimeout(() => { const newData = data .slice(nextIndex - 1, nextIndex + 9) .map(item => { return { id: item.id, content: item.content }; }); this.items = [...this.items, ...newData]; }, 1000); } } };
In this example, we use a fake data source to simulate the operation of obtaining data. In practical applications, we need to use our own data source and then dynamically load more data through asynchronous operations. With such a simple operation, we can implement an infinite scrolling list based on Vue.
The above is the detailed content of How to implement infinite scrolling list with Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


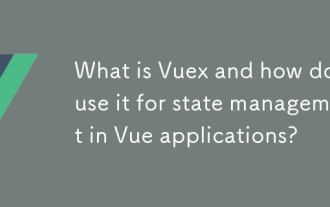
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri

This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf
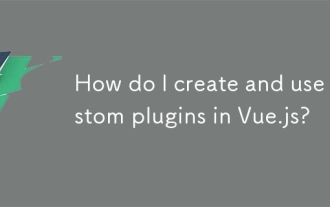
Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.
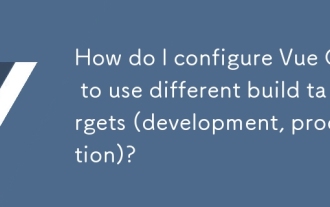
The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
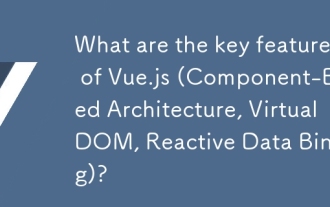
Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
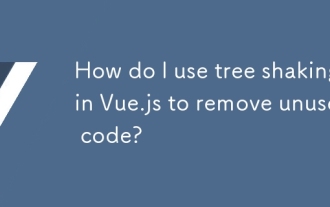
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159

The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.
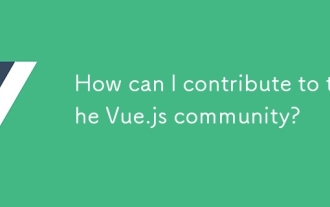
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje
