


How to solve the problem 'Error: No match found for location with path '/xxx'' when using vue-router in a Vue application?
Vue is a popular JavaScript framework that comes with a routing library called vue-router that makes it easy to manage routing in your application. But when using vue-router, you sometimes encounter the "Error: No match found for location with path "/xxx"" error, which is caused by path matching problems. This article explains how to resolve this issue.
In Vue applications, routing is managed through the vue-router library. This library provides a mechanism to map different URLs to application views. By using vue-router library we can easily route the application from one page to another.
The reason why "Error: No match found for location with path "/xxx"" appears is that sometimes the routing path defined in the application does not match the navigation link or is not defined, causing vue-router to be unable to parse the URL. path. This can be solved in a few different ways.
The first solution is to ensure that the routing path matching the application navigation link is defined in the router instance. In this case, you should check if the routes in your application are defined and make sure they match the navigation links. If such a path exists, it should be added to the routing definition in the application. Here is a sample code:
const router = new VueRouter({ routes: [ { path: '/', component: HomeComponent }, { path: '/about', component: AboutComponent }, { path: '/contact', component: ContactComponent }, { path: '/my-component', component: MyComponent }, ] })
In the above code, we have defined four different routing paths for the application. If the navigation link does not match any of these paths, "Error: No match found for location with path "/xxx"" will appear.
The second solution is to use wildcard paths. A wildcard path can match any path unless the path is explicitly defined in the route definition. Here is a sample code:
const router = new VueRouter({ routes: [ { path: '/', component: HomeComponent }, { path: '*', component: NotFoundComponent } ] })
In the above code, we have defined a wildcard route that can match any undefined path. This will redirect the navigation link to a separate "NotFoundComponent" instead of getting "Error: No match found for location with path "/xxx"".
The third solution is to use dynamic path segments. Dynamic path segment refers to the part of the routing path that contains dynamic parameters. You can use the colon ":" to define dynamic parameters, so that the value in the URL can be passed to the component. The following is a sample code:
const router = new VueRouter({ routes: [ { path: '/user/:id', component: UserComponent } ] })
In the above code, we define a dynamic path segment "/user/:id", where ":id" is a dynamic parameter. This way, when the user accesses "/user/1234", Vue will extract the parameter value from the URL and pass it into the component. This approach allows for flexibility in handling different routing paths and navigation links.
In short, when using vue-router, make sure that the route definition matches the navigation link and follow the best practices of the Vue routing library. If "Error: No match found for location with path "/xxx"" appears, you need to check whether the routing path and navigation link match, and try to use wildcard paths or dynamic path segments to solve the problem.
The above is the detailed content of How to solve the problem 'Error: No match found for location with path '/xxx'' when using vue-router in a Vue application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


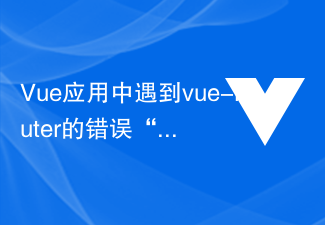
The vue-router error "NavigationDuplicated:Avoidedredundantnavigationtocurrentlocation" encountered in the Vue application – how to solve it? Vue.js is becoming more and more popular in front-end application development as a fast and flexible JavaScript framework. VueRouter is a code library of Vue.js used for routing management. However, sometimes
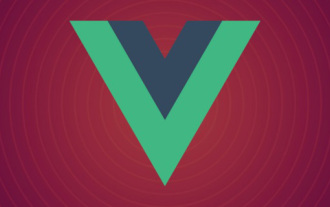
This article will give you a detailed explanation of the Vue-Router in the Vue family bucket, and learn about the relevant knowledge of routing. I hope it will be helpful to you!
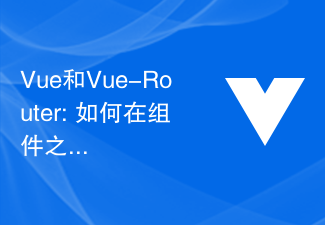
Vue and Vue-Router: How to share data between components? Introduction: Vue is a popular JavaScript framework for building user interfaces. Vue-Router is Vue's official routing manager, used to implement single-page applications. In Vue applications, components are the basic units for building user interfaces. In many cases we need to share data between different components. This article will introduce some methods to help you achieve data sharing in Vue and Vue-Router, and
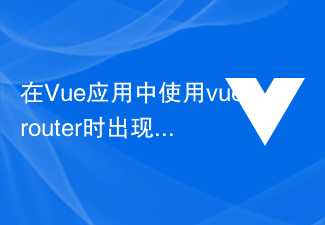
Vue is a popular front-end framework that allows developers to quickly build efficient, reusable web applications. Vue-router is a plug-in in the Vue framework that helps developers easily manage application routing and navigation. However, when using Vue-router, you sometimes encounter a common error: "Error:Invalidroutecomponent:xxx". This article will explain the causes and solutions to this error. The reason lies in Vu
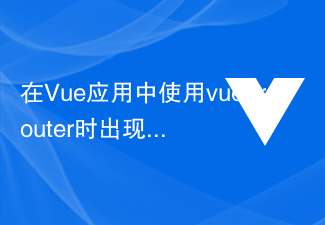
When using vue-router in a Vue application, the error message "Error: Avoidedredundantnavigationtocurrentlocation" sometimes appears. This error message means "avoiding redundant navigation to the current location" and is usually caused by clicking the same link repeatedly or using the same routing path. So, how to solve this problem? Use the exact modifier when defining the router
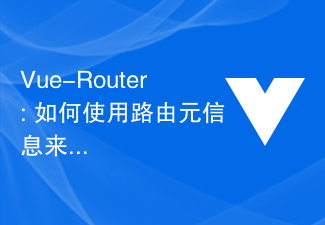
Vue-Router: How to use routing meta information to manage routes? Introduction: Vue-Router is the official routing manager of Vue.js, which can help us quickly build single-page applications (SPA). In addition to common routing functions, Vue-Router also supports the use of routing meta information to manage and control routing. Routing metainformation is a custom attribute that can be attached to a route, which can help us implement some special logic or permission control. 1. What is routing metainformation? The routing meta information is
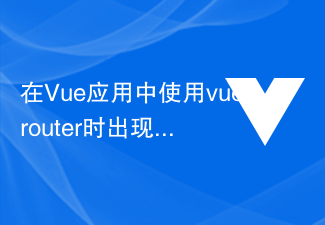
Using vue-router is a common way to implement routing control in Vue applications. However, when using vue-router, the error "Error: Failedtoresolveasynccomponent:xxx" sometimes occurs, which is caused by an asynchronous component loading error. In this article, we will explore this problem and provide solutions. Understand the principle of asynchronous component loading. In Vue, components can be created synchronously or asynchronously.
![How to deal with '[Vue warn]: Unknown custom element' error](https://img.php.cn/upload/article/000/887/227/169253293916658.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
How to deal with "[Vuewarn]:Unknowncustomelement" error When developing web applications using Vue.js, we often encounter various error and warning messages. One of the common warning messages is "[Vuewarn]:Unknowncustomelement". This error usually occurs when using custom components. In this article I will show you how to handle this error and provide some code examples to help you
