How to use Swoole to implement a high-performance HTTP client
In modern network applications, the HTTP client is a crucial component. They can be used to access REST APIs, exchange data and perform remote procedure calls. However, some conventional HTTP client implementations may face performance issues such as network latency, handling a large number of requests, etc. Swoole, a high-performance network library based on PHP, can effectively solve these problems.
In this article, we will explore how to use Swoole to implement a high-performance HTTP client.
1. Basic knowledge
Before we delve into how to use Swoole to implement a high-performance HTTP client, we need to understand the basic knowledge of Swoole.
Swoole is a network framework that supports asynchronous, multi-threading and can provide high-performance and low-latency network communication. Swoole includes support for TCP, UDP, HTTP and other network protocols, and provides event-driven, multi-process concurrency, coroutine and other features. The design concept of Swoole is for concurrent processing on modern computer hardware.
Swoole's core functionality is based on asynchronous I/O operations and allows you to create web servers and clients. Using Swoole can improve the performance of certain network applications and ensure high performance by reducing network latency, resource usage and other issues.
2. Use Swoole to implement HTTP client
Now, we begin to explore how to use Swoole to implement a high-performance HTTP client.
- Install Swoole
First, you need to install the Swoole extension. You can install Swoole using the following command:
pecl install swoole
- Using Swoole HTTP Client
The Swoole HTTP client can be used to send HTTP requests to other servers.
The following is an example of using the Swoole HTTP client to send an HTTP request to www.baidu.com:
<?php $client = new SwooleCoroutineHttpClient('www.baidu.com', 443, true); $client->setHeaders([ 'Host' => 'www.baidu.com', 'User-Agent' => 'Mozilla/5.0 (Windows NT 6.1; WOW64; rv:68.0) Gecko/20100101 Firefox/68.0', 'Accept' => 'text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8', 'Accept-Language' => 'en-US,en;q=0.5', 'Accept-Encoding' => 'gzip, deflate, br', 'Connection' => 'keep-alive', 'Upgrade-Insecure-Requests' => '1' ]); $client->set(['timeout' => 1]); $client->get('/'); echo $client->body;
In this example, we create a Swoole HTTP client and then send Baidu sent an HTTP GET request.
- Using coroutines and multiple requests
Using Swoole's coroutine feature, you can use the Swoole HTTP client to convert back and forth from one request to another. In many cases, this approach can significantly improve application performance.
The following is an example of using Swoole coroutine and multiple requests:
<?php go(function () { $cli = new SwooleCoroutineHttpClient('www.baidu.com', 443, true); $cli->setHeaders([ 'Host' => 'www.baidu.com', 'User-Agent' => 'Mozilla/5.0 (Windows NT 6.1; WOW64; rv:68.0) Gecko/20100101 Firefox/68.0', 'Accept' => 'text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8', 'Accept-Language' => 'en-US,en;q=0.5', 'Accept-Encoding' => 'gzip, deflate, br', 'Connection' => 'keep-alive', 'Upgrade-Insecure-Requests' => '1' ]); $cli->set(['timeout' => 1]); $cli->get('/'); $cli2 = new SwooleCoroutineHttpClient('www.google.com', 443, true); $cli2->setHeaders([ 'Host' => 'www.google.com', 'User-Agent' => 'Mozilla/5.0 (Windows NT 6.1; WOW64; rv:68.0) Gecko/20100101 Firefox/68.0', 'Accept' => 'text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8', 'Accept-Language' => 'en-US,en;q=0.5', 'Accept-Encoding' => 'gzip, deflate, br', 'Connection' => 'keep-alive', 'Upgrade-Insecure-Requests' => '1' ]); $cli2->set(['timeout' => 1]); $cli2->get('/'); echo $cli->body . PHP_EOL . $cli2->body; });
In this example, we use two Swoole HTTP clients to send HTTP requests to Baidu and Google . We use Swoole's coroutine feature to convert back and forth between different requests.
Summary
In modern network applications, HTTP clients are inevitable. However, traditional HTTP client implementations may face performance issues such as network latency, handling a large number of requests, etc. Using Swoole can improve the performance of your program and implement a high-performance HTTP client. Using Swoole's coroutine feature, you can quickly switch between different requests and further improve the performance of your application.
We hope this article can help you understand how to use Swoole to implement a high-performance HTTP client.
The above is the detailed content of How to use Swoole to implement a high-performance HTTP client. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


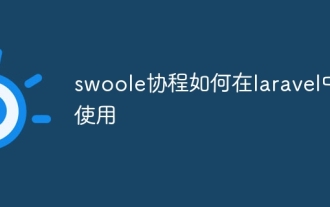
Using Swoole coroutines in Laravel can process a large number of requests concurrently. The advantages include: Concurrent processing: allows multiple requests to be processed at the same time. High performance: Based on the Linux epoll event mechanism, it processes requests efficiently. Low resource consumption: requires fewer server resources. Easy to integrate: Seamless integration with Laravel framework, simple to use.
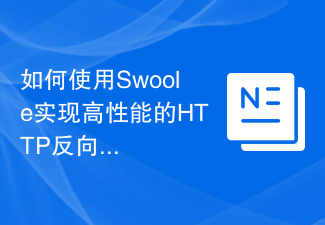
How to use Swoole to implement a high-performance HTTP reverse proxy server Swoole is a high-performance, asynchronous, and concurrent network communication framework based on the PHP language. It provides a series of network functions and can be used to implement HTTP servers, WebSocket servers, etc. In this article, we will introduce how to use Swoole to implement a high-performance HTTP reverse proxy server and provide specific code examples. Environment configuration First, we need to install the Swoole extension on the server
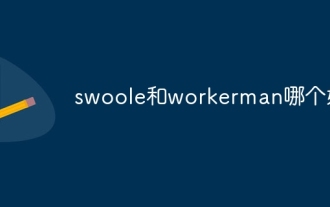
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.

Swoole Process allows users to switch. The specific steps are: create a process; set the process user; start the process.
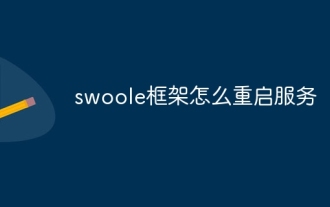
To restart the Swoole service, follow these steps: Check the service status and get the PID. Use "kill -15 PID" to stop the service. Restart the service using the same command that was used to start the service.
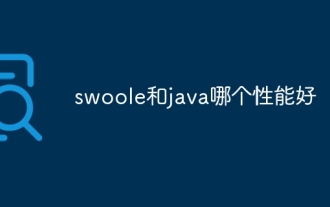
Performance comparison: Throughput: Swoole has higher throughput thanks to its coroutine mechanism. Latency: Swoole's coroutine context switching has lower overhead and smaller latency. Memory consumption: Swoole's coroutines occupy less memory. Ease of use: Swoole provides an easier-to-use concurrent programming API.
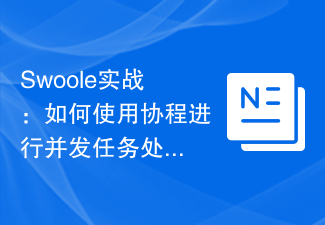
Swoole in action: How to use coroutines for concurrent task processing Introduction In daily development, we often encounter situations where we need to handle multiple tasks at the same time. The traditional processing method is to use multi-threads or multi-processes to achieve concurrent processing, but this method has certain problems in performance and resource consumption. As a scripting language, PHP usually cannot directly use multi-threading or multi-process methods to handle tasks. However, with the help of the Swoole coroutine library, we can use coroutines to achieve high-performance concurrent task processing. This article will introduce

Swoole is a high-performance PHP network development framework. With its powerful asynchronous mechanism and event-driven features, it can quickly build high-concurrency and high-throughput server applications. However, as the business continues to expand and the amount of concurrency increases, the CPU utilization of the server may become a bottleneck, affecting the performance and stability of the server. Therefore, in this article, we will introduce how to optimize the CPU utilization of the server while improving the performance and stability of the Swoole server, and provide specific optimization code examples. one,
