


PHP and Lumen integrate to realize microservice architecture development
With the rapid development of IT technology, modern enterprises are facing more and more complex business systems. The traditional single application architecture can no longer meet the needs, and the microservice architecture emerged as the times require. Microservice architecture is an architectural style in which applications are composed of multiple small services that can be deployed, run, expanded, and maintained independently to achieve better scalability, reusability, and flexibility. sex.
So, how to use PHP to implement microservice architecture? The answer is to use Lumen - a lightweight PHP framework. Based on Laravel, Lumen is a PHP framework designed for building microservices and APIs. This article will introduce how to integrate microservice architecture in Lumen framework.
Step 1: Install Lumen
First, we need to install the Lumen framework locally. The installation steps are the same as for Laravel. It can be installed through composer, as shown below:
1 |
|
After completion, we can start the Lumen framework running environment locally:
1 |
|
When "Lumen development server started:
Step Two: Write Microservices
Next, we will use the Lumen framework to write our first microservice. We are going to create a simple API that gets a list of all users. The steps to implement this API are as follows:
- Create API route
In the routing file routes/web.php, we need to write a route to handle requests to the API. In this example, we will write a GET route in the route file to handle /get_users requests, as shown below:
1 2 3 |
|
At this point, when you request http://localhost:8000/get_users, The string "List of all users" will be returned.
- Handling API Requests
Now, we need to create a controller class for our API. Let's create the UserController class that will be responsible for handling the get_users request and returning the list of users. We can create the UserController.php file in the app/Http/Controllers directory and copy the following code into it:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
In the above code, we define a public function getAllUsers(), which will Returns a list of all users as a JSON formatted response.
- Register API routing to the controller
Now we need to register the API routing to the UserController controller in the routing file and map our route /api/get_users to the method UserController@getAllUsers, and modify the route file as follows:
1 |
|
At this time, when you request http://localhost:8000/api/get_users, a user list in JSON format will be returned, as shown below :
1 2 3 4 5 |
|
Finally, we also need to import the UserController class at the top, as shown below:
1 2 3 |
|
In this way, we have successfully implemented a most basic API, and it can be used under the Lumen framework run.
Step Three: Integrate the Microservice Framework
Before this, we have created our first API in the Lumen framework, but it is not a real microservice application. In order to turn Lumen into a microservice architecture application, we need to use DDD domain model design ideas to rebuild our application. Then, we will use the idea of microservices to decompose each of our services into microservices.
- Domain Design
First, we need to define our domain model, which is the core of our application. In this example, we will create a simple user management microservice whose main function is to manage user data.
Our user management microservice must have the following functions:
- User Registration
- User Authentication
- User Authorization
- User information management
Now we need to map these functions to the interface of the microservice.
- Microservice interface
We will design different microservice interfaces according to different functions in the domain model. Here we will define the following microservice interface:
- User registration interface (registerUser)
- User authentication interface (authenticateUser)
- User authorization interface (authorizeUser)
- User information management interface (manageUserInfo)
- Microservice module
Now, we need to divide our microservice interface into different microservices in the service module. In this example, we will use the following three microservice modules:
- User Authentication and Authorization Microservice
- User Information Management Microservice
- User Registration Microservice Services
These microservice modules will interact directly with our database.
- Microservice Architecture
We now have our domain model, microservice interface and microservice module components, and we can now simply combine them into A microservices architecture. The entire architecture looks like this:
1 |
|
We enable the Lumen API Gateway, which is the initial point of our system and will receive all requests and route them to the appropriate microservice module. Our domain model is our business logic, and the microservice interface will control our data interactions.
第四步:实现微服务
现在,我们已经设计了微服务模块、微服务接口和整体微服务架构,我们可以开始实现我们的微服务。我们将针对上述三个微服务模块分别进行介绍。
1. 用户认证与授权微服务
我们的用户认证与授权微服务负责处理所有与用户认证相关的任务。它将接收用户凭据并验证它们的凭证是否正确。如果验证成功,它将生成一个JWT标记并将其返回给用户。
我们将针对以下任务编写用户认证与授权微服务:
- 为用户生成JWT标记
- 验证用户凭据
我们可以通过安装tymon / jwt-auth组件来编写我们的用户认证与授权微服务。使用以下命令进行安装:
1 |
|
然后,我们需要在配置文件中配置JWT密钥。现在,我们可以使用以下代码为用户认证与授权微服务创建一个新控制器:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
|
2. 用户信息管理微服务
用户信息管理微服务将负责向用户提供用户相关信息。在本例中,我们将创建以下操作的API:
- 获取用户个人信息
- 更新用户密码
首先,我们将创建一个新控制器来管理用户信息:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
3. 用户注册微服务
注册微服务将处理用户注册过程。在本例中,我们将创建以下功能:
- 创建一个新用户,保存到数据库中
- 发送电子邮件以验证用户的电子邮件地址
首先,我们将创建一个新控制器来处理用户注册。它应该读取POST有效载荷并保存新用户到数据库中。然后,它应该生成用户验证令牌并将其发送到用户的电子邮件地址。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
|
现在,我们可以在Lumen框架中实现我们的微服务。我们的系统现在有三个微服务模块:“用户认证和授权微服务”、“用户信息管理微服务”和“用户注册微服务”,这些微服务与数据库交互,并由Lumen API Gateway处理。
总结
本文介绍了如何在Lumen框架中集成微服务架构,包括领域模型设计、微服务接口和微服务模块的开发。以上代码展示了Lumen框架如何开发微服务的过程。通过使用Lumen框架,开发者可以快速构建微服务,提高代码质量,加快项目进程。
The above is the detailed content of PHP and Lumen integrate to realize microservice architecture development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




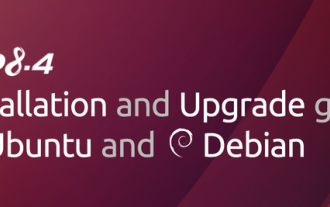
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
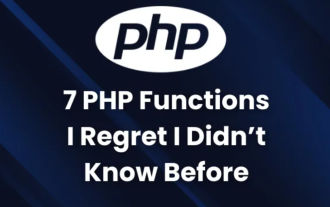
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
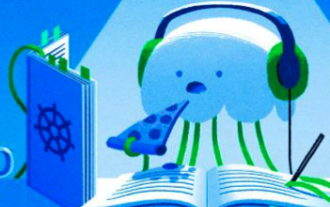
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
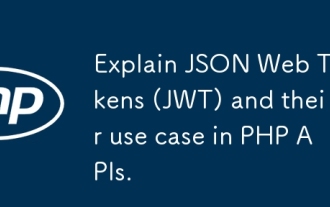
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
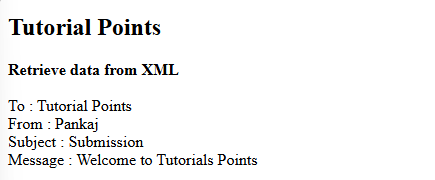
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
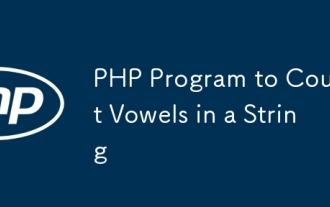
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
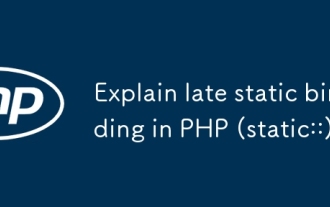
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
