Implementing Web API using Golang's Web framework Buffalo framework
Go language is an efficient, fast and easy-to-use programming language. Its concise syntax and powerful concurrency performance make it very popular among developers. Buffalo is an open source web framework based on the Go language. It provides a complete tool chain that can be used to quickly build web applications and APIs. In this article, we will explore how to build a web API using the Buffalo framework.
1. Overview of Buffalo
Buffalo is a Web framework based on Go language. It provides a complete tool chain that allows developers to easily build web applications and APIs. The Buffalo framework mainly has the following features:
- Fast: Buffalo's design goal is to minimize work and time.
- Easy to use: The Buffalo framework provides simple and easy-to-use CLI tools and template engines, so that developers do not need to pay too much attention to the underlying details.
- Extensible: The Buffalo framework has good scalability, supports plug-in mechanisms, and provides external API interfaces.
- Lightweight: The Buffalo framework code is clear and concise, easy to understand and maintain.
Before starting to use the Buffalo framework, we need to do some basic configuration and installation.
2. Install the Buffalo framework
Before using Buffalo, we need to install the Go language environment and the Buffalo framework. To install the Go language, you can refer to the instructions on the official website. To install the Buffalo framework, you can use the following command:
$ go get -u -v github.com/gobuffalo/buffalo/buffalo
3. Create a Buffalo project
After installing the Buffalo framework, we can create a new Buffalo project. Use the following command:
$ buffalo new myproject --api
where, myproject is the project name, and the --api parameter indicates that what we want to create is an API, not a Web application. After the project is successfully created, we can use the following command to enter the project directory:
$ cd myproject
Next, we can start the Buffalo application development server using the following command:
$ buffalo dev
After executing the above command , we can open http://127.0.0.1:3000/ in the browser and see the Buffalo framework default page.
4. Implement Web API
Next we will implement a simple Web API. We need to create an API method first. In the project directory, we can enter the following command:
$ buffalo generate action greet --method=GET
After executing the above command, we can find a new greet.go file in the project directory. This file defines a greet method, which can be accessed through GET requests.
In the greet.go file, we need to write the following code:
package actions import "github.com/gobuffalo/buffalo" func Greet(c buffalo.Context) error { name := c.Param("name") return c.Render(200, r.JSON(map[string]string{"message": "Hello, " + name + "!"})) }
This method will read the passed name parameter and return a JSON-formatted response that contains the greeting information. Now we need to add this method to Buffalo's routes. We can find the randomly generated routes file in the project directory and add the following code:
app.GET("/greet/{name}", Greet)
In the routes file, we define a route named /greet/{name}, which will match a name for Greet's method. This method will read the passed name parameter and return a JSON formatted response.
Now we have completed the creation of the Web API. Restart the Buffalo application development server using the following command:
$ buffalo dev
Enter the following URL in the browser to test our Web API:
http://127.0.0.1:3000/greet/world
Open the above URL in the browser, You should see a response in JSON format, which will greet "world":
{"message": "Hello, world!"}
5. Conclusion
In this article, we introduced how to create a Web API using Buffalo, the web framework of Go language . The Buffalo framework is fast, easy to use, scalable, and lightweight, making it ideal for building web applications and APIs. For example, in this article, we used the Buffalo framework to quickly and easily create a web application with a RESTful API. If you need to build efficient and easy-to-maintain web applications or APIs, Buffalo Framework will be a very good choice.
The above is the detailed content of Implementing Web API using Golang's Web framework Buffalo framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


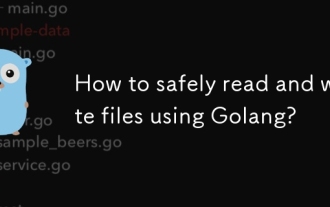
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
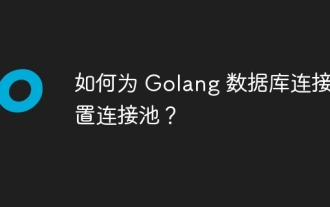
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
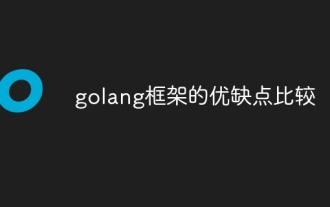
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
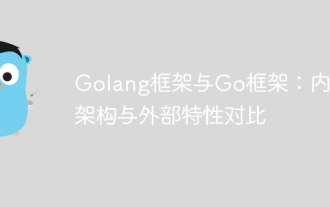
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
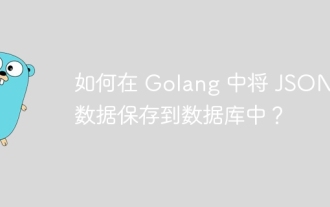
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
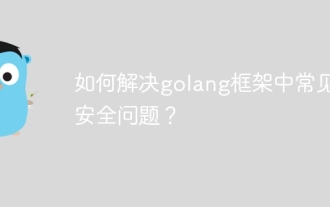
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
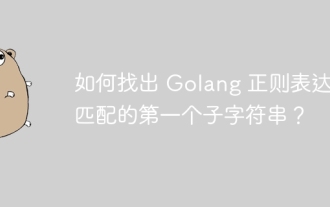
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
